How to Use Safe Navigation in Ruby
- Understanding Safe Navigation Operator
- Using Safe Navigation with Nested Objects
- Safe Navigation in Collections
- Best Practices for Using Safe Navigation
- Conclusion
- FAQ
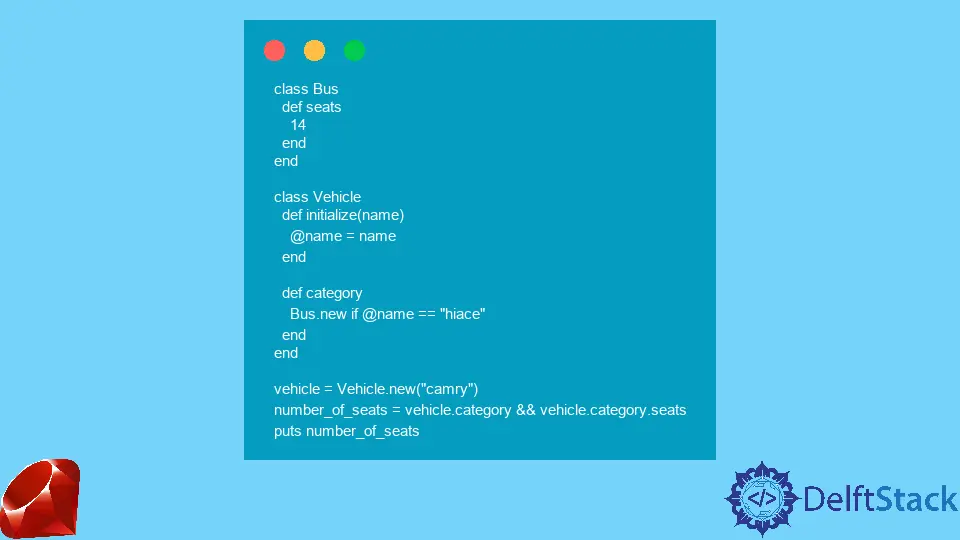
In the world of programming, especially with Ruby, developers often encounter situations where they need to access properties of objects that might not exist. This can lead to errors if not handled correctly. Fortunately, Ruby provides a powerful feature known as safe navigation, which allows developers to navigate through objects without worrying about nil values causing exceptions.
In this tutorial, we will explore how to utilize safe navigation in Ruby effectively. We will cover its syntax, use cases, and best practices, ensuring you can confidently implement this feature in your own projects. Whether you’re a beginner or an experienced Rubyist, this guide will enhance your understanding and help you write cleaner, safer code.
Understanding Safe Navigation Operator
The safe navigation operator in Ruby is represented by the &.
syntax. This operator provides a way to call methods on an object only if that object is not nil. If the object is nil, the expression will return nil instead of throwing an error. This feature is particularly useful when dealing with nested objects or optional attributes.
Here’s a simple example to illustrate how it works:
user = nil
user_name = user&.name
puts user_name
Output:
In this example, since user
is nil, user&.name
does not raise an error; instead, it simply returns nil. This prevents the dreaded NoMethodError that would occur if you tried to call user.name
directly.
Safe navigation is a great way to simplify your code and make it more readable. It eliminates the need for verbose nil checks, allowing you to focus on the logic of your application.
Using Safe Navigation with Nested Objects
One of the most powerful applications of the safe navigation operator is when dealing with nested objects. In many applications, you may have objects that contain other objects, and accessing properties of these nested objects can lead to complications if any of them are nil.
Consider the following scenario where we have a company
object that contains an address
object, which in turn has a city
attribute:
company = nil
city_name = company&.address&.city
puts city_name
Output:
Here, if company
is nil, the entire expression evaluates to nil without raising an error. This is incredibly useful when you are unsure if an object exists at various levels of nesting. Instead of writing multiple nil checks, you can simply chain the safe navigation operator.
This approach not only makes your code cleaner but also enhances its maintainability. It allows you to focus on the core logic without being bogged down by repetitive nil checks.
Safe Navigation in Collections
Another area where the safe navigation operator shines is when working with collections. Often, you may want to access elements within an array or a hash that might not exist. The safe navigation operator can streamline this process.
Let’s say you have an array of users, and you want to access the name of the first user, but the array might be empty:
users = []
first_user_name = users.first&.name
puts first_user_name
Output:
In this example, if the users
array is empty, users.first
returns nil, and consequently, first_user_name
will also be nil. This prevents potential errors that could arise from trying to access the name of a non-existent user.
Using the safe navigation operator in collections not only reduces the amount of conditional logic you need to write but also improves the readability of your code. It conveys the intent clearly that you are aware of the possibility of nil values and are handling them gracefully.
Best Practices for Using Safe Navigation
While the safe navigation operator is a powerful tool, it’s essential to use it wisely. Here are some best practices to keep in mind:
-
Use it for Optional Attributes: Reserve the safe navigation operator for attributes that are genuinely optional or might be nil. Overusing it can lead to code that is hard to read and understand.
-
Combine with Other Methods: Don’t hesitate to combine the safe navigation operator with other Ruby methods like
map
,select
, oreach
for more complex data manipulations. -
Keep it Simple: Avoid chaining too many calls with the safe navigation operator. If you find yourself doing this, consider refactoring your code to make it clearer.
-
Readability Matters: Always prioritize readability. If using the safe navigation operator makes your code harder to understand, consider using traditional nil checks or other techniques.
By following these best practices, you can harness the power of the safe navigation operator while keeping your codebase clean and maintainable.
Conclusion
In conclusion, the safe navigation operator is a valuable feature in Ruby that allows developers to handle nil values gracefully. By using the &.
syntax, you can avoid unnecessary errors and write cleaner, more readable code. Whether you’re dealing with nested objects, collections, or optional attributes, the safe navigation operator provides a straightforward solution. By following the best practices outlined in this tutorial, you can enhance your Ruby programming skills and build more robust applications. Embrace safe navigation, and you’ll find that your code becomes more resilient and easier to maintain.
FAQ
-
what is the safe navigation operator in Ruby?
The safe navigation operator, represented by&.
, allows you to call methods on an object only if it is not nil, preventing errors. -
when should I use the safe navigation operator?
Use it when you are unsure if an object or its attributes may be nil. It simplifies your code and reduces the need for multiple nil checks. -
can I chain multiple safe navigation calls?
Yes, you can chain multiple calls, but be cautious not to overdo it, as it can make your code harder to read. -
does safe navigation work with collections?
Absolutely! It works well with arrays and hashes, allowing you to safely access elements that may not exist. -
are there any downsides to using the safe navigation operator?
While it simplifies code, overuse can lead to reduced readability. Use it judiciously for optional attributes.