How to Use the inject Method in Ruby
- What is the Inject Method?
- Summing Numbers with Inject
- Concatenating Strings with Inject
- Building Hashes with Inject
- Using Inject with Custom Objects
- Conclusion
- FAQ
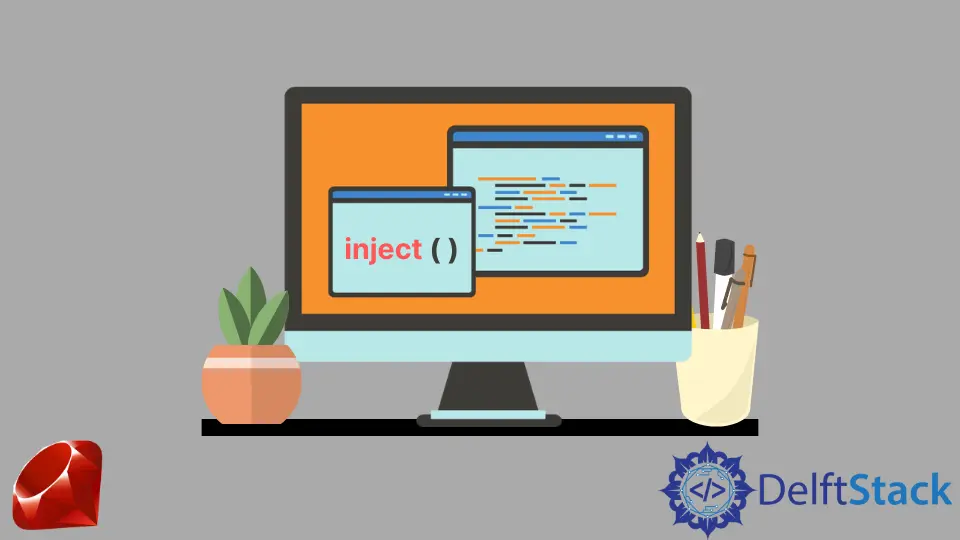
The inject method in Ruby is a powerful and versatile tool that can transform how you handle collections. Whether you’re summing numbers, concatenating strings, or even building complex data structures, inject can streamline your code and make it more readable.
In this article, we will explore the various uses of the inject method in Ruby, providing practical examples and explanations along the way. By the end, you’ll have a solid understanding of how to leverage this method to enhance your Ruby programming skills. So, let’s dive into the world of inject and discover its potential!
What is the Inject Method?
The inject method, also known as fold or reduce in other programming languages, is used to accumulate values from a collection. It takes a block and an optional initial value, iterating through each element of the collection while maintaining a cumulative result. The beauty of inject lies in its flexibility; you can use it for various operations, such as summing numbers, concatenating strings, and even more complex tasks like building hashes or arrays.
Here’s a simple example to illustrate how inject works:
numbers = [1, 2, 3, 4, 5]
sum = numbers.inject(0) { |accum, num| accum + num }
In this code, we initialize the accumulation with 0 and then add each number from the array to it.
Output:
15
The final result is 15, which is the sum of all numbers in the array.
Summing Numbers with Inject
One of the most common uses of the inject method is summing up numbers in an array. Instead of using a loop, you can achieve this in a more elegant way. Here’s how you can sum an array of integers:
numbers = [10, 20, 30, 40, 50]
total = numbers.inject(0) { |sum, number| sum + number }
In this example, we start with an initial sum of 0. The block takes each number from the array, adds it to the cumulative sum, and returns the updated value.
Output:
150
The total of the numbers in the array is 150. This concise approach not only makes your code cleaner but also enhances its readability.
Concatenating Strings with Inject
The inject method can also be effectively used for string concatenation. If you have an array of strings and want to combine them into one single string, inject can help you accomplish this with ease. Here’s an example:
words = ["Hello", "world", "from", "Ruby"]
sentence = words.inject("") { |result, word| result + word + " " }
In this case, we start with an empty string and concatenate each word from the array, adding a space after each word for clarity.
Output:
Hello world from Ruby
The final output is a complete sentence. This method is particularly useful when you need to create a string from multiple components without resorting to cumbersome loops or additional methods.
Building Hashes with Inject
Inject is not limited to numbers and strings; you can also use it to build complex data structures like hashes. Let’s say you want to count the occurrences of each word in an array. You can achieve this using inject as follows:
words = ["apple", "banana", "apple", "orange", "banana", "apple"]
word_count = words.inject(Hash.new(0)) do |count, word|
count[word] += 1
count
end
Here, we initialize a new hash with a default value of 0. For each word in the array, we increment its count in the hash.
Output:
{"apple"=>3, "banana"=>2, "orange"=>1}
The resulting hash shows the count of each word. This method is exceptionally handy for tasks that require data aggregation and analysis.
Using Inject with Custom Objects
Inject can also be beneficial when working with custom objects. Suppose you have a collection of objects and want to aggregate a specific attribute. Here’s an example:
class Product
attr_accessor :name, :price
def initialize(name, price)
@name = name
@price = price
end
end
products = [
Product.new("Laptop", 1000),
Product.new("Phone", 500),
Product.new("Tablet", 300)
]
total_price = products.inject(0) { |sum, product| sum + product.price }
In this example, we define a Product class with attributes for name and price. We then create an array of products and use inject to calculate the total price.
Output:
1800
The total price of all products is 1800. This demonstrates how inject can be seamlessly integrated with custom data types, making your code adaptable and powerful.
Conclusion
The inject method in Ruby is a versatile tool that can simplify your code and enhance its readability. From summing numbers and concatenating strings to building hashes and working with custom objects, inject offers a streamlined approach to handling collections. By incorporating this method into your programming toolkit, you can write cleaner, more efficient Ruby code. So, the next time you find yourself looping through a collection, consider using inject to make your life easier!
FAQ
-
What does the inject method do in Ruby?
The inject method accumulates values from a collection, applying a block of code to each element and maintaining a cumulative result. -
Can I use inject with custom objects?
Yes, inject can be used with custom objects by accessing their attributes within the block. -
What is the difference between inject and each in Ruby?
The each method simply iterates over a collection without returning a value, while inject accumulates a result based on the logic defined in the block. -
Is inject the same as reduce?
Yes, inject is synonymous with reduce in Ruby; both methods perform the same function of accumulating values. -
Can I use inject without an initial value?
Yes, if you do not provide an initial value, inject will use the first element of the collection as the initial accumulator.