Is Ruby Single Threaded
- What Is Threading?
- Is Ruby Single-Threaded or Multi-Threaded?
- Ruby’s Threading Model Overview
- The Single-Threaded Nature of Ruby
- Concurrency in Ruby
- An Example of a Ruby Multi-Threading Program
- Conclusion
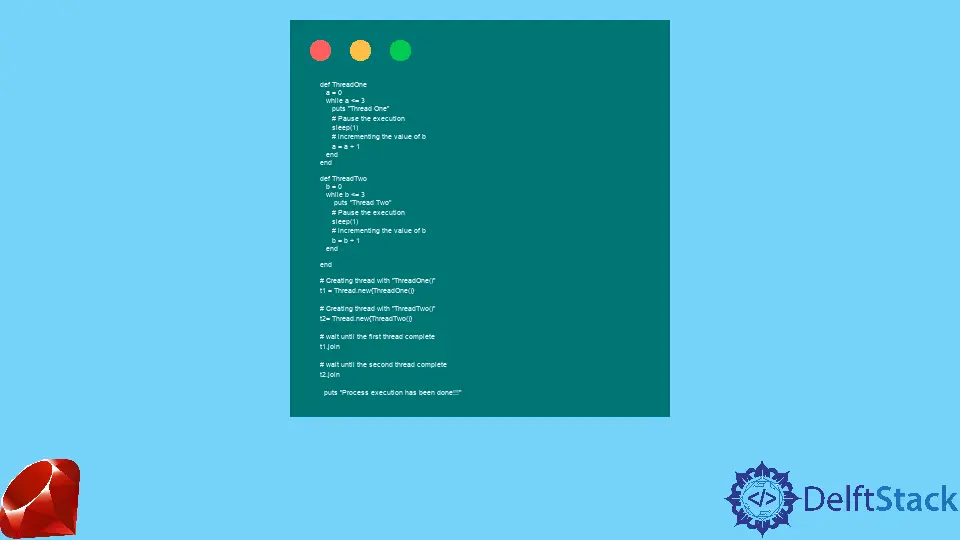
Ruby, a dynamic and object-oriented programming language, is renowned for its elegant syntax and developer-friendly features. One aspect that often sparks curiosity is its threading model.
Multi-threading is an important topic in modern programming languages. But we must run a program in a single thread for other purposes.
The question of whether Ruby is single-threaded or supports multi-threading is a topic that merits exploration. In this article, we’ll delve into the details of Ruby’s threading model, examining its origins, single-threaded nature, concurrent execution, and practical implications for developers.
What Is Threading?
A thread is a block of codes and commands that execute on the CPU sequentially. It is a lightweight process that runs on the CPU.
If multiple threads exist on your program, they can share the same resources, like variables and methods. Threading makes efficient use of the CPU and provides faster execution.
Is Ruby Single-Threaded or Multi-Threaded?
Yes, Ruby supports multi-threading. It allows you to create concurrent programming of two or more parts (thread) for using the CPU efficiently.
If you program in Ruby with a single thread, it will execute through one core of the CPU, so if your CPU is quad-core, then the other three cores will not be involved in executing your program.
So, the program without threading or a single thread is slower than a multithread program. That’s why single-thread programs are not recommended in modern programming.
Besides, a web application performs more than one task simultaneously. So, it is very hard to design a web application using a single thread.
Ruby’s Threading Model Overview
Ruby, at its core, is single-threaded. This means that it executes one instruction at a time in a sequential manner. However, this statement requires some clarification to understand the nuances of Ruby’s concurrency model.
Threads vs. Processes
In computing, a thread is the smallest unit of execution within a process, and a process is an independent program in execution. Ruby programs run within a process, and within that process, multiple threads can be spawned.
Global Interpreter Lock (GIL)
One crucial aspect impacting Ruby’s concurrency is the Global Interpreter Lock (GIL).
The GIL is a mutex that protects access to Ruby objects, preventing multiple threads from executing Ruby code simultaneously. This lock is a characteristic of the standard Ruby interpreter (MRI - Matz’s Ruby Interpreter).
The GIL ensures thread safety by allowing only one thread to execute Ruby code at any given time. While this design simplifies the implementation of the interpreter, it also introduces limitations to concurrent execution.
The Single-Threaded Nature of Ruby
Given the presence of the GIL, Ruby’s MRI can execute only one thread at a time, making it effectively single-threaded concerning Ruby code. This means that even on a multi-core machine, concurrent Ruby threads won’t execute simultaneously.
Implications of the GIL
The GIL’s presence affects the performance of CPU-bound tasks in multi-threaded Ruby programs. Since only one thread can execute Ruby code at a time, parallelization of CPU-bound tasks doesn’t see significant performance improvements.
However, the GIL’s impact is less pronounced for I/O-bound tasks. When a thread is waiting for I/O operations (e.g., reading from a file or making a network request), other threads can execute Ruby code.
This makes Ruby threads suitable for scenarios with heavy I/O operations, such as web servers handling numerous concurrent requests.
Concurrency in Ruby
While MRI’s GIL introduces limitations to multi-core parallelization, Ruby provides options for concurrency, particularly for I/O-bound tasks. The Thread
class in Ruby allows developers to create multiple threads within a single process.
Thread Creation in Ruby
thread1 = Thread.new { puts 'Thread 1 executing' }
thread2 = Thread.new { puts 'Thread 2 executing' }
thread1.join
thread2.join
Output:
Thread 1 executing
Thread 2 executing
In this example, two threads (thread1
and thread2
) are created, and each prints a message. The join
method is then used to ensure that the main program waits for both threads to finish before proceeding.
Fiber and Concurrent Programming
In addition to threads, Ruby 1.9 and later versions introduced Fibers, lightweight and cooperative concurrency primitives.
Fibers allow developers to achieve concurrency without the GIL restrictions. However, they require explicit cooperation between fibers using methods like Fiber.yield
.
An Example of a Ruby Multi-Threading Program
In our example below, we will illustrate how we can use multithread in a Ruby program. Have a look at the below example code.
def ThreadOne
a = 0
while a <= 3
puts 'Thread One'
# Pause the execution
sleep(1)
# incrementing the value of b
a += 1
end
end
def ThreadTwo
b = 0
while b <= 3
puts 'Thread Two'
# Pause the execution
sleep(1)
# incrementing the value of b
b += 1
end
end
# Creating thread with "ThreadOne()"
t1 = Thread.new { ThreadOne() }
# Creating thread with "ThreadTwo()"
t2 = Thread.new { ThreadTwo() }
# wait until the first thread is complete
t1.join
# wait until the second thread is complete
t2.join
puts 'Process execution has been done!!!'
The purpose of each line is already left as comments. After running the above Ruby program, you will get the below output in your console.
Thread One
Thread Two
Thread One
Thread Two
Thread One
Thread Two
Thread One
Thread Two
Process execution has been done!!!
Conclusion
In conclusion, Ruby is single-threaded in the sense that only one thread can execute Ruby code at a time due to the presence of the Global Interpreter Lock (GIL) in the standard Ruby interpreter (MRI). While this makes Ruby less suitable for CPU-bound parallelism, it still offers concurrency benefits for I/O-bound tasks.
Developers seeking parallel execution on multi-core machines or exploring options for CPU-bound tasks may consider alternative Ruby implementations like JRuby or Rubinius, which do not have a Global Interpreter Lock and allow for true multi-threading.
Understanding the nuances of Ruby’s threading model empowers developers to make informed decisions when designing concurrent applications, selecting the appropriate tools, and optimizing performance based on the nature of the tasks at hand.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn