for vs each in Ruby
- The Basics of Iteration in Ruby
- Using the For Loop
- Using the Each Method
- Performance Considerations
- Conclusion
- FAQ
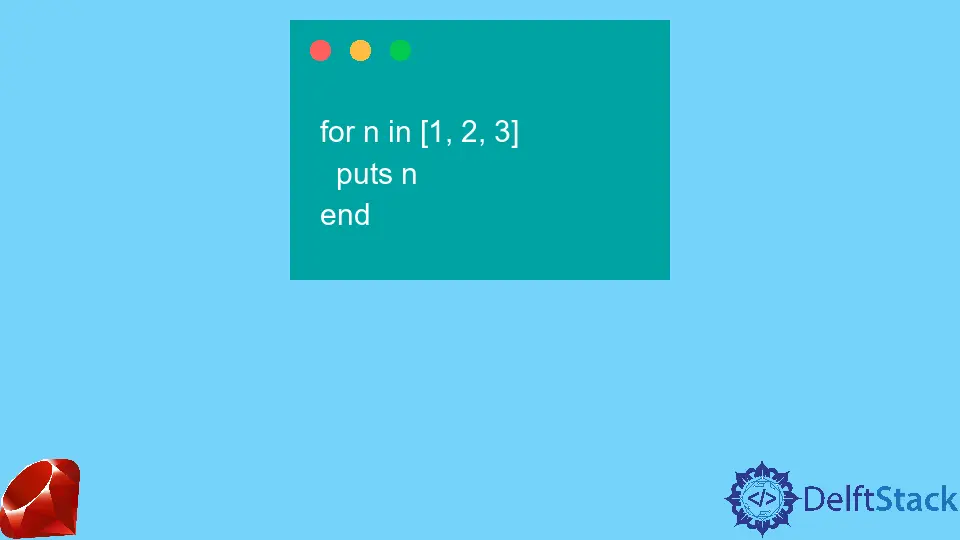
When working with Ruby, you’ll often encounter two common ways to iterate over collections: for
loops and the each
method. While both serve the same fundamental purpose of iterating through elements, they have distinct characteristics and use cases. Understanding these differences can enhance your coding efficiency and readability.
In this article, we’ll explore the nuances between for
and each
, helping you determine when to use each approach effectively. Whether you’re a beginner or looking to refine your Ruby skills, this guide will provide you with clear examples and insights.
The Basics of Iteration in Ruby
Before diving into the specifics, it’s essential to grasp what iteration means in the context of Ruby. Iteration is the process of accessing each element in a collection, such as an array or a hash. Ruby offers several ways to perform this operation, with for
and each
being two of the most popular methods.
The for
loop is a traditional control structure that has been part of many programming languages, while each
is a method that belongs to Ruby’s Enumerable module. Both can be used to traverse collections, but they do so in slightly different ways.
Using the For Loop
The for
loop is straightforward and easy to understand. It allows you to iterate over a range or an array by declaring a variable that takes on the value of each element in turn.
Here’s a simple example of using a for
loop to iterate through an array of numbers:
numbers = [1, 2, 3, 4, 5]
for number in numbers
puts number
end
Output:
1
2
3
4
5
In this example, we define an array called numbers
and then use a for
loop to print each number. The loop iterates through the array, assigning each value to the variable number
, which is then printed to the console.
One notable aspect of the for
loop is that it creates a new scope for the variable, which can lead to unintended consequences if you’re not careful. For instance, if you define a variable outside the loop with the same name, it won’t be affected by the loop’s variable.
Using the Each Method
On the other hand, the each
method is more idiomatic in Ruby and is often preferred for its expressive nature. It allows for more concise and readable code, especially when working with blocks.
Here’s how you can achieve the same result using the each
method:
numbers = [1, 2, 3, 4, 5]
numbers.each do |number|
puts number
end
Output:
1
2
3
4
5
In this example, the each
method is called on the numbers
array. The block (defined by do |number| ... end
) is executed for each element in the array, with number
taking on the value of the current element. This method is not only cleaner but also aligns with Ruby’s object-oriented principles.
Another advantage of using each
is that it allows for chaining with other enumerable methods, enhancing the flexibility of your code. For instance, you could easily add a map
or select
method after each
to further process the elements.
Performance Considerations
When it comes to performance, both for
and each
are generally efficient for most use cases. However, the choice between them can impact the readability and maintainability of your code.
The for
loop may be slightly faster in some circumstances since it operates at a lower level. Yet, this difference is negligible for most applications. The real advantage of using each
lies in its integration with Ruby’s Enumerable module, which provides a wealth of additional methods for collection manipulation.
Conclusion
In summary, both for
and each
are valuable tools for iteration in Ruby, each with its unique strengths. The for
loop is straightforward and familiar, while each
offers a more idiomatic, readable approach that aligns with Ruby’s design philosophy. Ultimately, the choice between them should depend on your specific use case and coding style preferences. By understanding the differences and nuances, you can write clearer, more efficient Ruby code.
FAQ
-
What is the main difference between for and each in Ruby?
The main difference lies in their syntax and scope. Thefor
loop creates a new scope for the iterator variable, whileeach
uses a block to iterate over elements within the same scope. -
Which method is preferred in Ruby for iteration?
Theeach
method is generally preferred in Ruby due to its readability and compatibility with other enumerable methods.
-
Can I use for and each interchangeably?
While both can achieve similar results, they are not interchangeable due to differences in scope and syntax. It’s essential to choose the one that best fits your coding style and requirements. -
Are there performance differences between for and each?
There may be slight performance differences, but they are generally negligible for most applications. The choice should focus more on readability and maintainability. -
Can I use blocks with the for loop?
No, thefor
loop does not support blocks in the same way thateach
does. If you need to use a block,each
is the better choice.