How to Accept User Input With Gets in Ruby
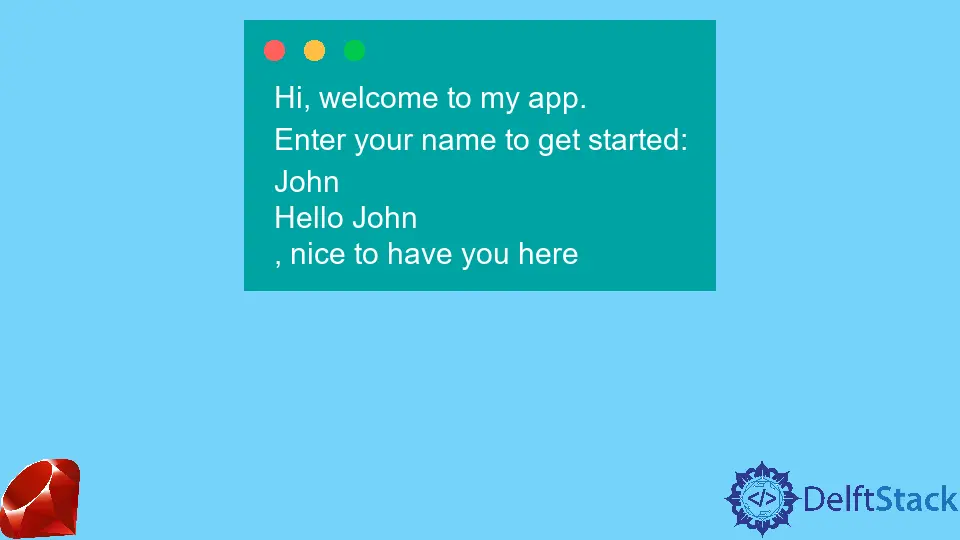
In the world of programming, accepting user input is a fundamental skill that allows applications to be interactive and responsive. In Ruby, the gets
method is a straightforward way to capture input from users. However, raw input can often include unwanted newline characters or leading/trailing spaces that can complicate processing. That’s where the chomp
method comes in handy.
This tutorial will guide you through the process of accepting user input using gets
and sanitizing it with chomp
. By the end of this article, you will be equipped with the knowledge to effectively handle user input in your Ruby applications. Let’s dive into the details!
Understanding the Gets Method
The gets
method in Ruby reads a line from standard input (usually the keyboard) until the user presses Enter. This method returns the input as a string, including the newline character at the end. To make the input more manageable, we often use chomp
, which removes the trailing newline character from a string. This combination of gets
and chomp
is essential for clean and usable user input.
Here’s a simple example of how to use gets
and chomp
together:
puts "Please enter your name:"
name = gets.chomp
puts "Hello, #{name}!"
Output:
Please enter your name:
John
Hello, John!
In this snippet, we prompt the user to enter their name. The gets
method captures the input, and chomp
removes the newline character. Finally, we greet the user with their name. This simple interaction demonstrates the power of combining gets
and chomp
.
Accepting Numeric Input
When dealing with numeric input, it’s crucial to ensure that the user enters valid data. Using gets
and chomp
can help, but we also need to handle potential errors gracefully. Here’s how you can accept a numeric input and ensure it’s a valid number:
puts "Please enter your age:"
age_input = gets.chomp
if age_input.match?(/^\d+$/)
age = age_input.to_i
puts "You are #{age} years old."
else
puts "That's not a valid age."
end
Output:
Please enter your age:
25
You are 25 years old.
In this example, we first prompt the user to enter their age. After capturing the input with gets
, we use a regular expression to check if the input consists solely of digits. If it does, we convert the input to an integer and display the age. If not, we inform the user that their input is invalid. This method ensures that we only accept valid numeric input, enhancing the robustness of your application.
Handling Multiple Inputs
Sometimes, you may need to capture multiple pieces of input from the user. Ruby allows you to easily gather several inputs in succession. Here’s how you can do that:
puts "Please enter your first name:"
first_name = gets.chomp
puts "Please enter your last name:"
last_name = gets.chomp
puts "Hello, #{first_name} #{last_name}!"
Output:
Please enter your first name:
Jane
Please enter your last name:
Doe
Hello, Jane Doe!
In this code snippet, we prompt the user for their first and last names separately. Each input is captured using gets
and sanitized with chomp
. Finally, we greet the user using their full name. This approach is simple yet effective for gathering multiple user inputs in an organized manner.
Conclusion
Accepting user input in Ruby using gets
and sanitizing it with chomp
is a fundamental skill that every Ruby developer should master. By understanding how to effectively capture and manage user input, you can create more interactive and user-friendly applications. Whether you’re gathering a single piece of information or multiple inputs, the combination of gets
and chomp
provides a robust solution. With the examples and explanations provided in this tutorial, you should feel confident in implementing user input in your own Ruby projects.
FAQ
-
What does the
gets
method do in Ruby?
Thegets
method reads a line of input from standard input until the user presses Enter. -
Why do we use
chomp
withgets
?
We usechomp
to remove the trailing newline character from the input string, making it cleaner and easier to work with. -
How can I validate numeric input in Ruby?
You can use regular expressions to check if the input consists only of digits before converting it to a number. -
Can I accept multiple inputs in Ruby?
Yes, you can usegets
multiple times to capture different pieces of input from the user. -
What happens if the user inputs invalid data?
You should implement error handling to inform the user of invalid input and prompt them to try again.