The Purpose of Three Dots in React
- the JavaScript EcmaScript6
-
the Three Dots
...
Operator in React - the Rest Parameter in React
-
Use the
Spread
Operator to Refer All Elements of the Array in React -
Use the
...
to Combine Arrays in React -
Use the
...
Operator to Unpack the Property Names and Values of theProps
Object in React -
Use the
setState()
Method to Update the State of Class Components in React
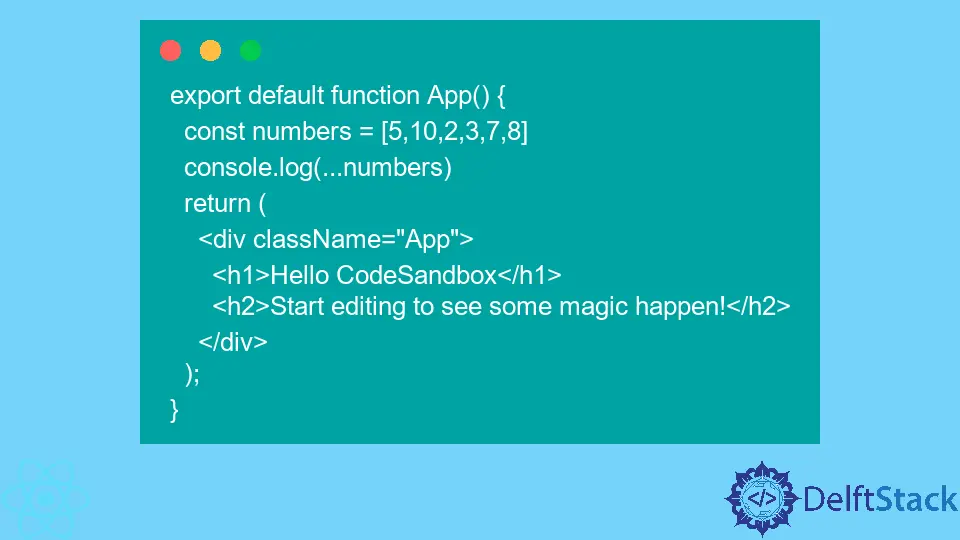
These days React the most popular framework for building modern web applications. React environment includes compilers, transpilers, and similar tools that allow you to declaratively write reusable components.
The same tools allow React developers to use JavaScript’s modern features and syntax even before being officially introduced to the language.
the JavaScript EcmaScript6
In 2015, web developers were first introduced to the new major JavaScript revision called EcmaScript6, or ES6 for short.
It introduced many useful methods, the new and improved syntax for writing functions, new types of variables, and many other cool features.
As it stands today, ES6 is the modern JavaScript, and most web developers are expected to be fluent in it.
We can confidently say that ES6 is not the final version of JavaScript, and more changes are expected.
We can certainly say that JavaScript will become simpler based on current trends. Some operators and syntax will disappear, and new ones will take their place.
Expressions that were once complicated, such as destructuring, will become much simpler. And JavaScript most likely will continue evolving in this direction.
the Three Dots ...
Operator in React
The introduction of the ellipsis
operator was one of the biggest, game-changing features of ES6.
The three dots syntax is used for multiple purposes and behaves differently based on how and where it’s used.
the Rest Parameter in React
When used in a function with one of the arguments, JavaScript ellipses can indicate that the function will accept an unlimited number of arguments.
Let’s look at an example:
function addition(x, y, ...arguments) {
sum(x,y); // 15
sum(arguments); // 20
};
addition(5,10,2,3,7,8)
In this case, the order of arguments is respected: 5 is accepted as the x
argument, 10 as the y
argument.
The rest are all lumped in the arguments
variable because the rest operator precedes it.
Use the Spread
Operator to Refer All Elements of the Array in React
Three dots in React can also represent a spread
operator. Developers use it to refer to all elements of the array, pass them in a function as an argument, like in this example:
export default function App() {
const numbers = [5,10,2,3,7,8]
console.log(...numbers)
return (
<div className="App">
<h1>Hello CodeSandbox</h1>
<h2>Start editing to see some magic happen!</h2>
</div>
);
}
All items in the numbers
array will be passed as individual arguments to the console.log()
in this react component.
In the above example, the call to the function is equivalent to console.log(5,10,2,3,7,8)
. You can look at the example on CodeSandBox.
Use the ...
to Combine Arrays in React
There are multiple ways to combine arrays in JavaScript, using spread
operators. It’s probably the easiest to write and read.
const yellowFruits = ['peach', 'banana', 'lemon'] const greenFruits =
['apple', 'kiwi', 'lime'] const fruits = [...yellowFruits, ...greenFruits]
console.log(fruits) // ["peach", "banana", "lemon", "apple", "kiwi", "lime"]
As you can see, the spread
operator combines two arrays. Without it, the two arrays would be combined in one, but the fruits array would consist of two separate arrays.
Not the individual array items within them. Let’s look at an example:
const yellowFruits = ['peach', 'banana', 'lemon'] const greenFruits =
['apple', 'kiwi', 'lime'] const fruits = [yellowFruits, greenFruits]
console.log(
fruits) // [["peach", "banana", "lemon"], ["apple", "kiwi", "lime"]]
Use the ...
Operator to Unpack the Property Names and Values of the Props
Object in React
The most recent JavaScript update, ES2018, introduced a new use for the ...
operator. It allows you to take every key-value pair from an object.
Most often, React developers use it to unpack the properties and values of the props
object, which is used to manually pass down data from the parent component to the child.
For instance, imagine we have a Container
component that receives props from its parent and wants to pass it down to its own child CustomText
component.
You could use the property spread
operator to unpack the property names and values of the props
object. Let’s take a look at the code:
export default function Container(props) {
return (
<div className="general">
<CustomText {...props}></CustomText>
</div>
);
}
Let’s imagine the props
object looked something like this:
{ size: 15, font: 'Calibri', color: 'yellow' }
In this case, using the property spread
operator ...
will assign the following attributes to the child component:
export default function Container(props) {
return (
<div className="general">
<CustomText size={this.props.size} font={this.props.font} color={this.props.color}></CustomText>
</div>
);
}
If the props
object has 10 properties, all 10 would be added to the CustomText
component.
Use the setState()
Method to Update the State of Class Components in React
To update the state of class components in React, we use the setState()
method. However, you can’t update the value of a state property directly.
Instead, you must pass an entire state and the specific property with an updated value. Let’s look at an example of the setState()
method call:
this.setState(previousState => {textStyles: {...previousState.textStyles, size:20}})
In this case, we use the default behavior of the property spread
operator to easily copy all properties with their values from the previous state.
If the previous state already had size
property, the new state won’t contain it two times. The size
property will have a value of 20
since it was specified in the most recent setState
call.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn