How to Use Ternary Operators in React
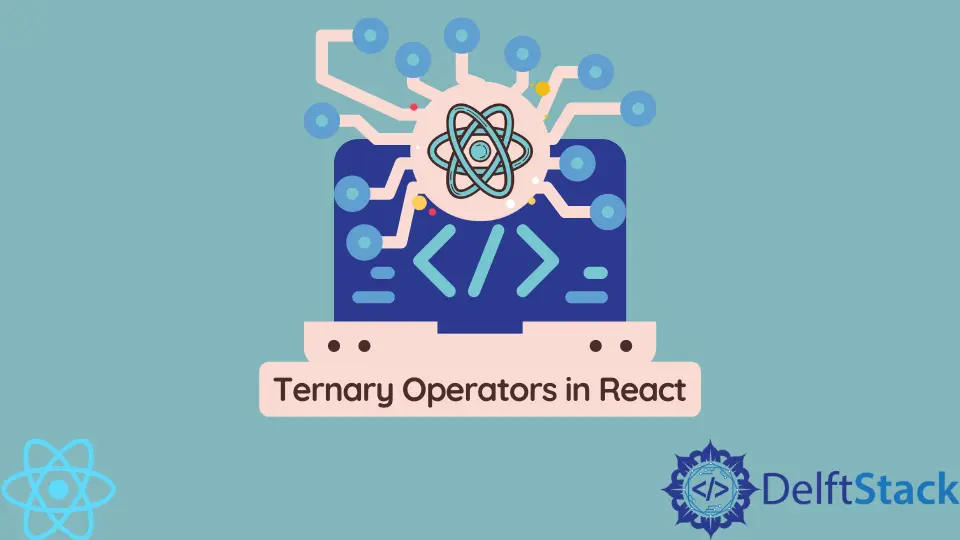
React is a framework used for building user interfaces with more features than basic HTML websites, but it’s still possible to change the appearance of elements using CSS styles.
Ternary operators, in particular, can be used to generate dynamic values.
Use Ternary Operators in React
React applications are commonly written in a templating language called JSX. It’s similar to HTML in many ways, but it offers more features.
Within JSX, there are multiple ways for React developers to set up conditions. You can use the if/else
or switch
statements, but a third way is a ternary operator.
The ternary operator is a fairly simple way to set up a condition and return specific values based on whether true or false. This can set up dynamic classes, apply dynamic inline styles, or conditionally render string values.
You can even add custom functionality to your application using a ternary operator.
Use Ternary Operators in React Outside of the return
Statement
Since React is a JavaScript-based framework, you can use regular JavaScript outside the return
statement. Any conditions, such as if/else
or switch
, can be set up here.
In this example, we will use a ternary operator to generate dynamic values for the className
attribute. We have a string and add the result of a ternary operator, which is another string.
Example:
export default function App() {
const [night, setNight] = useState(true);
const classNames = "base " + night ? "nightmode" : "";
return (
<div className={classNames}>
<h1>Hello, World</h1>
</div>
);
}
In this example, we used a ternary operator to conditionally generate a class name for the <div>
container. You can check the appearance of the App
component in this live demo.
The final value of the classNames
variable will depend on the value of the night
state variable. By default, we set the value of the night
variable to true
.
Unless we change that value using the setNight()
function, the ternary operator will return the 'nightmode'
string value.
Use Ternary Operators in JSX
JSX is the default templating language for building React applications. You can use curly braces inside JSX and write valid JavaScript expressions between them, including a ternary operator.
You can use the ternary operator to configure dynamic classes in React. It is a short and simple syntax.
First, you must write a JavaScript expression that evaluates true
or false
, followed by a question mark.
Afterward, you’ll have two values separated by a colon. The first one will be returned if the condition is true, and the second one will be returned if it is false.
Example:
export default function App() {
const [night, setNight] = useState(true);
return (
<div className={"base " + night ? "nightmode" : ""}>
<h1>Hello, World</h1>
</div>
);
}
This way, you can generate dynamic values inline. You can also use a ternary operator to render texts dynamically. For instance, to output an error text when there’s an error.
Example:
export default function App() {
const [night, setNight] = useState(true);
const [error, setError] = useState(false);
const classNames = "base" + night ? "nightmode" : "";
const handleInputChange = (data) => {
if (data.indexOf("@") == true) {
return null;
} else {
setError(true);
}
};
return (
<div>
<div className={classNames}>
<h1>Hello, World</h1>
</div>
Enter your email address:
<input onChange={(e) => handleInputChange(e.target.value)}></input>
<p style={{ color: error ? "red" : "black" }}>
{error ? "please enter a valid email address" : "Everything is fine"}
</p>
</div>
);
}
Here, we prompt users to enter their email addresses and perform a very simple validation to make sure what they enter is consistent with general patterns of email addresses.
First, we created an <input>
element, and listened for onChange
event to call the handleInputChange()
event handler. Everything we type into the text input element will be passed to the event handler as an argument.
In the event handler, we used a simple if/else
statement to check the value provided by users. If it contains the ‘@’ symbol, the value passes the test, and we can assume it’s a valid email address.
If not, we will raise the alarm using the setError
function.
We used the error
state variable, a boolean that indicates whether or not users should be alarmed. We also defined a setError
function, used to update the value of this state variable.
Finally, we used a ternary operator to check whether or not we should display a text warning users about entering a valid email address. If the error
state variable is true, our ternary operator will return a warning message; if not, it will return the string 'Everything is fine'
.
We also conditionally apply styles to make the text red if it contains a warning message for additional benefits to the UX. Otherwise, it will stay the default black color.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn