How to Show and Hide Element in React
- Using State for Show and Hide Functionality
- Conditional Rendering with Ternary Operator
- Using CSS Classes for Show and Hide
- Conclusion
- FAQ
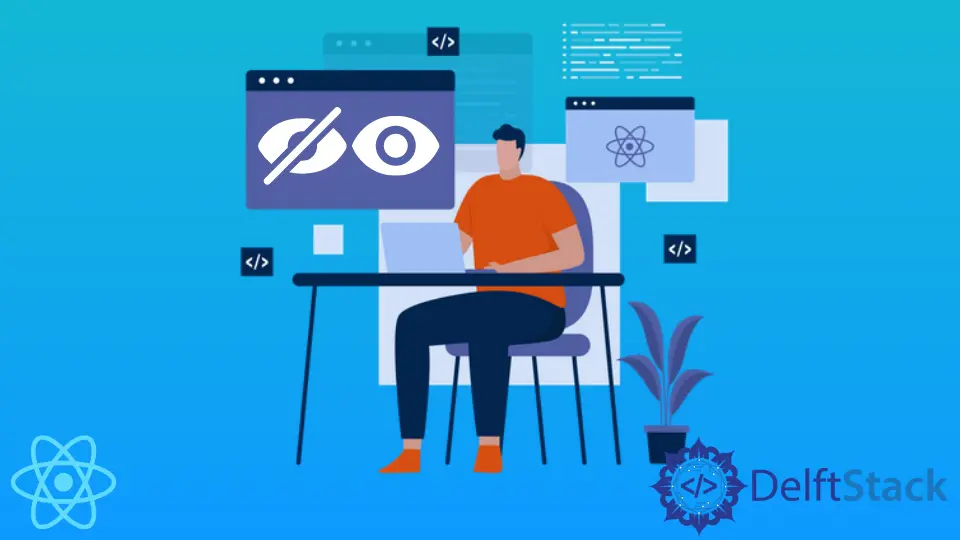
In the world of web development, user interaction is key to creating engaging applications. One common requirement in React applications is the ability to show and hide elements dynamically based on user actions. Whether you’re building a form that reveals additional fields or a menu that expands upon clicking, understanding how to toggle visibility is essential.
In this tutorial, we’ll explore various methods to achieve this functionality in React. We will delve into the use of state, conditional rendering, and event handling, providing clear examples to guide you through the process. By the end of this article, you’ll have the tools you need to enhance your React applications with interactive elements.
Using State for Show and Hide Functionality
The most straightforward way to show and hide elements in React is by using the component’s state. By maintaining a piece of state that tracks whether an element should be visible or hidden, you can easily toggle this state based on user interactions.
Here’s a simple example that demonstrates this concept:
import React, { useState } from 'react';
function ToggleComponent() {
const [isVisible, setIsVisible] = useState(false);
const toggleVisibility = () => {
setIsVisible(!isVisible);
};
return (
<div>
<button onClick={toggleVisibility}>
{isVisible ? 'Hide' : 'Show'} Element
</button>
{isVisible && <div>This is a toggleable element!</div>}
</div>
);
}
export default ToggleComponent;
In this code, we start by importing the necessary React hooks. The useState
hook initializes a state variable called isVisible
, which is set to false
by default. The toggleVisibility
function updates this state when the button is clicked. The conditional rendering ({isVisible && <div>...</div>}
) ensures that the element is only displayed when isVisible
is true
.
Output:
Using state to manage visibility is a clean and effective approach. It allows for easy integration with other components and ensures that your UI is responsive to user actions. As you build more complex applications, this method will become invaluable.
Conditional Rendering with Ternary Operator
Another effective way to show and hide elements in React is through conditional rendering using the ternary operator. This approach provides a concise way to conditionally render different elements based on the state.
Here’s how you can implement this:
import React, { useState } from 'react';
function TernaryToggleComponent() {
const [isVisible, setIsVisible] = useState(false);
const toggleVisibility = () => {
setIsVisible(!isVisible);
};
return (
<div>
<button onClick={toggleVisibility}>
Toggle Element
</button>
{isVisible ? (
<div>This element is now visible!</div>
) : (
<div>This element is hidden.</div>
)}
</div>
);
}
export default TernaryToggleComponent;
In this example, we again use the useState
hook to manage the visibility state. The button toggles the isVisible
state just like before. However, instead of using an if
statement or logical AND, we utilize a ternary operator to render different content based on the visibility state.
The ternary operator allows for a more compact syntax, making your code cleaner and easier to read. This method is particularly useful when you have more than two states to manage or when you want to display different elements based on the same condition.
Using CSS Classes for Show and Hide
Sometimes, you may want to manage visibility through CSS classes instead of purely relying on conditional rendering. This method allows for more complex styling and animations when showing or hiding elements.
Consider the following example:
import React, { useState } from 'react';
import './ToggleStyles.css'; // Assuming you have a CSS file for styles
function ClassToggleComponent() {
const [isVisible, setIsVisible] = useState(false);
const toggleVisibility = () => {
setIsVisible(!isVisible);
};
return (
<div>
<button onClick={toggleVisibility}>
Toggle Element
</button>
<div className={isVisible ? 'visible' : 'hidden'}>
This element's visibility is controlled by CSS classes!
</div>
</div>
);
}
export default ClassToggleComponent;
In this example, we define two CSS classes: visible
and hidden
. The visible
class could set the display to block
, while the hidden
class could set it to none
. The button click toggles the isVisible
state, which in turn changes the class of the div
.
Output:
Using CSS classes for visibility control not only provides a cleaner separation of concerns but also allows for smoother transitions and animations. You can easily add fade effects, slide downs, or other animations by manipulating CSS properties, enhancing the user experience significantly.
Conclusion
Showing and hiding elements in React is a fundamental skill that enhances interactivity in your applications. By leveraging state management, conditional rendering, and CSS classes, you can create dynamic user interfaces that respond to user actions. Whether you choose to use state for straightforward toggling or CSS for more complex visual effects, understanding these methods will empower you to build engaging applications. As you continue to develop your React skills, keep experimenting with these techniques to find the best approach for your specific use cases.
FAQ
-
What is the best way to show and hide elements in React?
Using state management with conditional rendering is the most common and effective method. -
Can I use CSS animations when showing or hiding elements?
Yes, you can apply CSS classes to manage visibility and incorporate animations for a smoother user experience. -
How does the ternary operator work in React?
The ternary operator is a shorthand for an if-else statement, allowing you to conditionally render elements based on a boolean value. -
Is it possible to show multiple elements at once?
Yes, you can manage an array of states or use more complex logic to control the visibility of multiple elements simultaneously. -
Can I combine these methods?
Absolutely! You can mix and match state management, conditional rendering, and CSS classes to achieve the desired functionality in your React applications.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn