How to Select DOM Elements in React
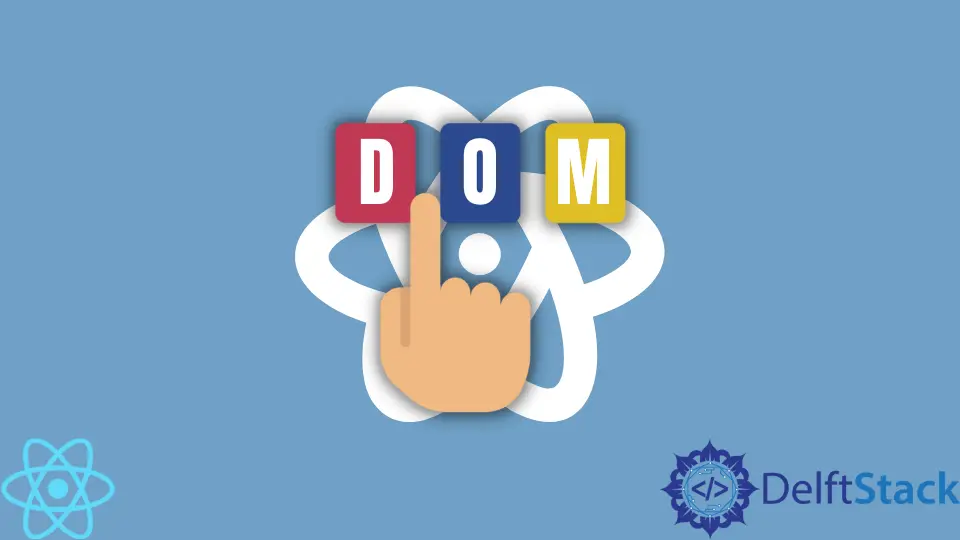
At one point or another, every JavaScript developer has used the getElementById()
method. It takes one argument id
and returns an HTML element with that ID. This method is extremely useful for doing manual DOM mutations.
Grabbing an HTML element node is a common necessity. Many developers who are experienced in JavaScript, but new to React framework, want to know how to perform this operation in React.
Refs in React
Refs, short for references, are an easy way to access the DOM in React. In order to link the element (or component) with a reference, the element must have a ref
attribute that equals your ref. A sample <div>
element can be linked to testRef
this way: <div ref={this.testRef}></div>
. It is a good practice to store ref
instances in a variable.
in Functional Components
Since the introduction of hooks about two years ago, functional components have become much more robust. If you’re running a modern version of React (for instance, 16.8.0), you can import the useRef()
hook, which can be used to define a ref. Here’s an example of a functional component that references a random variable during the render:
function App() {
const elementVariable = useRef(null)
useEffect(() => console.log(elementVariable), [] )
return (
<div ref={elementVariable}>
<h1></h1>
</div>
)
}
In this example, by setting elementVariable
equal to the instance of the useRef()
hook, we’re storing the HTML node in the variable.
Hook won’t work unless the HTML element has a ref
attribute set to the variable name.
One argument passed to the useRef()
hook specifies the initial value of the ref
instance. Once the <div></div>
element has mounted, the variable will hold the HTML node value. Here’s what the console will look like:
in Class Components
For a long time, using class components was the default way to create interactive React components.
Since the release of version 16.3, React developers can use React.createRef()
to create a ref. The API might be different, but both React.createRef()
and useRef()
hook create essentially the same ref instances.
In class components, the ref
instance is stored in a class property. Here’s an example:
class App extends Component {
constructor(props){
super(props)
this.ref = React.createRef()
}
componentDidMount(){
console.log(this.ref);
}
render() {
return <h1 ref={this.ref}>Referenced Text</h1>;
}
}
If you look at the console, you’ll see an object with the current
property that contains the HTML node.
Developers using React version >16.3 must use slightly different syntax to create refs. Example:
<div ref={(element) => this.ref = element}></div>
The reference created this way is called a callback ref
because we set the ref
attribute to a callback function that takes the HTML element as an argument and assigns this value to the ref
property of the class instance.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn