How to Load Screen While DOM Is Rendering Using React
-
Use the
this.state
Function to Load Screen While DOM Is Rendering Using React -
Use the
useEffect
Hook to Load Screen While DOM Is Rendering Using React -
Use the
react-loader-spinner
Dependency to Load Screen While DOM Is Rendering Using React - Conclusion
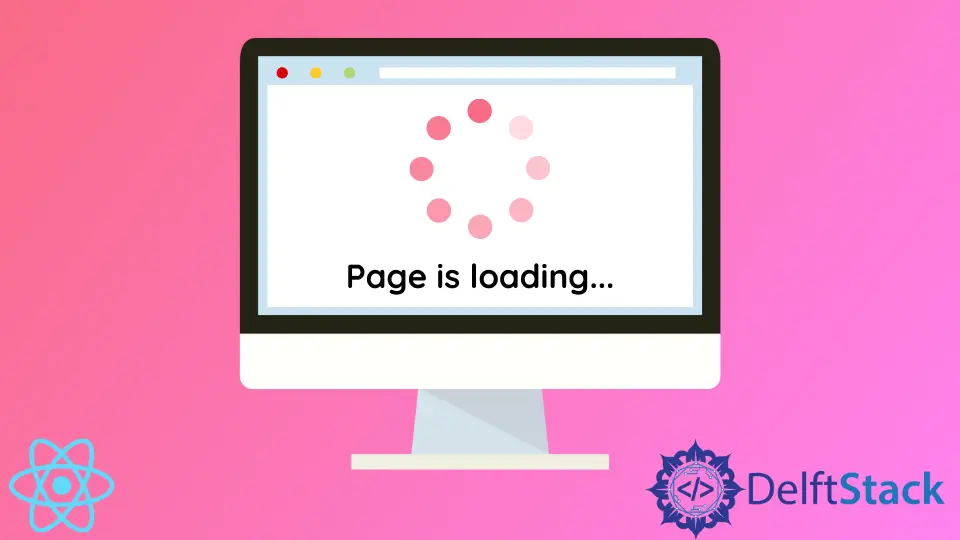
Web development is not just about building apps that load fast and without bugs, it is also about making them beautiful, attractive, and pleasing to the eye, and one cannot say the blank page that greets us when we launch an app in React is pleasing to the eye.
We can do something about this blank page by creating effects that are displayed just before the React DOM renders the page’s main contents, and there are different ways to do this.
Use the this.state
Function to Load Screen While DOM Is Rendering Using React
This example provides a much more straightforward approach by taking advantage of the application’s loading time. Inside the App.js
file of our project
folder, we will input these codes:
Code Snippet- App.js
:
import React, {Component} from "react"
class App extends Component {
constructor() {
super()
this.state = {
loading: false,
character: {}
}
}
componentDidMount() {
this.setState({loading: true})
fetch("https://swapi.dev/api/people/1/")
.then(response => response.json())
.then(data => {
this.setState({
loading: false,
character: data
})
})
}
render() {
const text = this.state.loading ? " page is loading..." : this.state.character.name
return (
<div>
<p>{text}</p>
</div>
)
}
}
export default App
Output:
We are using the fetch()
API to fetch the name of a movie character, that is, the main content displayed on the page. We use the this.state
function to tell React that while the page content is still being fetched, display page is loading...
instead, and after a few seconds, the main content, Luke Skywalker
comes up.
Use the useEffect
Hook to Load Screen While DOM Is Rendering Using React
React useEffect
is a nice approach to displaying contents and moving animations around the screen, and we will use this approach to create a preloading screen.
First, we will access the index.html
file inside the public
folder of our project
folder and create the loading screen effect there. Our image has a link, which we will utilize and then create stylings.
See the code below.
Code Snippet- index.html
:
<!DOCTYPE html>
<html lang="en">
<head>
<title>React App</title>
<style>
.preloader {
display: flex;
justify-content: center;
}
.rotate {
animation: rotation 9s infinite linear;
}
.loader-hide {
display: none;
}
@keyframes rotation {
from {
transform: rotate(0deg);
}
to {
transform: rotate(359deg);
}
}
</style>
</head>
<body>
<div class="preloader">
<img src="https://previews.123rf.com/images/nolimit46/nolimit462006/nolimit46200600489/148500740-modern-glowing-preloader-and-progress-loading-circle-bar-3d-render-background.jpg" class="rotate" width="100" height="100" />
</div>
<div id="root"></div>
</body>
</html>
Then in the App.js
, we apply the useEffect
to control the loading effects, with the CSS’s support inside the index.html
.
Code Snippet- App.js
:
import React, { useEffect } from "react";
import "./App.css";
const loader = document.querySelector(".preloader");
const showLoader = () => loader.classList.remove("preloader");
const addClass = () => loader.classList.add("loader-hide");
const App = () => {
useEffect(() => {
showLoader();
addClass();
}, []);
return (
<div style={{ display: "flex", justifyContent: "center" }}>
<h2>App react</h2>
</div>
);
};
export default App;
Output:
Use the react-loader-spinner
Dependency to Load Screen While DOM Is Rendering Using React
The React Loader dependency is a suitable example for creating beautiful preloading screens in react. It offers a wide range of loading effects that website visitors will love before they enter a website.
Inside the project
folder we have created, we will install the dependency with:
npm install react-loader-spinner
Then create a folder named components
and two files, Loading.js
and Clock.js
. Loading.js
will contain codes for the loading screen effect, and Clock.js
will display the main page content.
Then we go into the App.js
file to set things:
Code Snippet- App.js
:
import React, {useState, useEffect} from 'react';
import Loading from './components/Loading';
import Clock from './components/Clock';
function App() {
const [isLoading, setIsLoading] = useState(true);
useEffect(() => {
setTimeout(() => {
setIsLoading(false);
}, 2500);
})
return (
<div>
{isLoading===true?
<Loading/>:
<Clock/>
}
</div>
);
}
export default App;
We are using the useState
hook to control the state of the Loading
component, and then with useEffect
, we tell React how long we want the loading screen to linger before the main page is rendered from the DOM.
Then in the Loading.js
file, where we want to set the loading screen, we import the kind of effect we want from the loader spinner dependency and the CSS setting for the dependency. We then copy and paste the settings for that particular effect from this site:
https://www.npmjs.com/package/react-loader-spinner
This should look like the following code.
Code Snippet- Loading.js
:
import React from 'react'
import "react-loader-spinner/dist/loader/css/react-spinner-loader.css"
import { Puff } from 'react-loader-spinner'
const Loading = () => {
return (
<div align="center">
<Puff
height="100"
width="100"
color='grey'
ariaLabel='loading'
/>
</div>
)
}
export default Loading
Finally, we set the main content of the page inside the Clock.js
like this:
Code Snippet-Clock.js
:
import React from 'react'
const Clock = () => {
return (
<div align='center'>
<h1>CLOCK</h1>
</div>
)
}
export default Clock
Output:
As we have set inside the App.js
file, once the loading screen reaches its lifecycle, the Clock.js
file contents are displayed.
Conclusion
Who does not want to avoid building a boring website with nothing to wow the visitors? Creating a loading screen is one way to shorten the waiting time, where visitors stare at blank screens until the website loads.
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn