How to Render an Array of Data in React
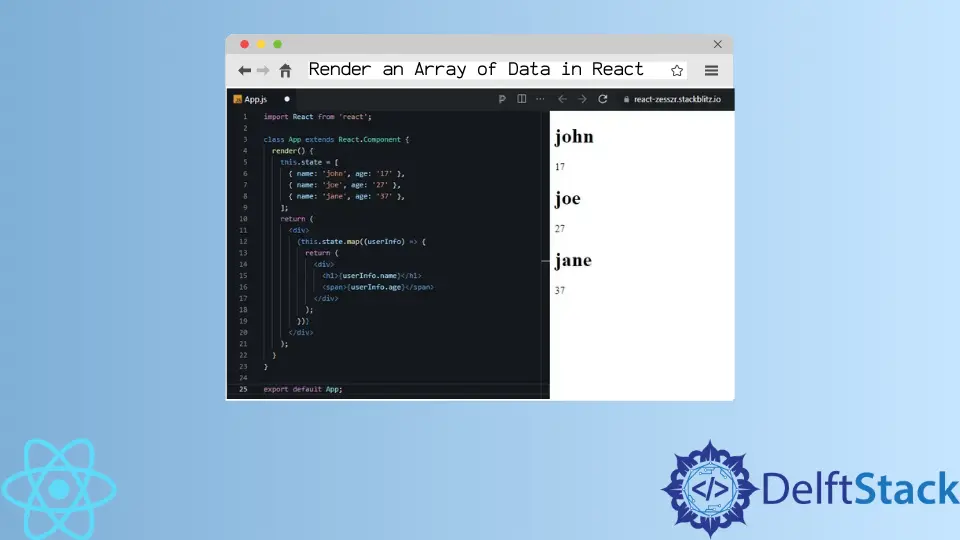
This tutorial will discuss rendering items in an array using the Array.map()
function in React.
Use the Array.map()
Function to Render an Array of Data in React
There are instances where we need to display items in a list on our web page. This is where the array.map()
function becomes useful when we use the React framework to create websites.
Amazingly, this React function works well for rendering a small and large list of items. Hence, we will look at two examples that utilize the array.map()
rendering capability as we advance.
Example 1:
To begin using Visual Studio Code, we open the terminal and create a new react app using npx create-react-app itemslist
. After this is done, we then head to the app.js
file in the src
folder.
In the app.js
, we write the following code:
import './App.css';
import React from 'react';
import logo from './logo.svg';
class App extends React.Component {
render() {
this.state = [
{name: 'john', age: '17'},
{name: 'joe', age: '27'},
{name: 'jane', age: '37'},
];
return (
<div>
{this.state.map((userInfo) => {
return (
<div><h1>{userInfo.name} <
/h1>
<span>{userInfo.age}</span >
</div>
);
})}
</div>);
}
}
Output:
Breaking down the code above, the userInfo
stores all the data in the this.state
array. Then, to render the names in the array, we type in userInfo.name
, and to display the age, we code in userInfo.age
.
Example 2:
This example will take a more complex approach to become more versatile when rendering lists of items in an array.
After we have created the react app, we then navigate to the App.js
and write the code below:
import './App.css'
import React, {Component} from 'react';
import NameList from './components/NameList'
class App extends Component {
render() {
return (
<div className='App'>
<NameList />
</div>
)
})
}
export default App;
In the above code snippet, observe that we imported the NameList
component into the App.js
and added it to our render array.
Now, we will create the NameList
component under the src > components
folder and name it NameList.js
.
After that is done, we navigate it and write some code.
import React from 'react'
import Person from './Person'
function NameList() {
const persons = [
{
id: 1,
name: 'Bruce',
age: 30,
skill: 'React'
},
{
id: 2,
name: 'Clark',
age: 25,
skill: 'Angular'
},
{
id: 3,
name: 'Diana',
age: 28,
skill: 'Vue'
}
]
const personList = persons.map(person =>
<Person person={
person} />)
return <div>{personList}</div>
}
export default NameList
Everything should work this way, but we can still make the coding more sophisticated. We will create another file called person.js
in the Components folder.
Then we will pass the JSX in the NameList.js
into the Person.js
. That means we will remove everything inside the <h2></h2>
and put it in the Person.js
.
We will pass the props inside the NameList.js
, it will look like this:
const personList = persons.map(person ==> <Person person={
person} />)
return <div>{personList}</div>
Then the Person.js
will look like this:
import React from 'react'
function Person({person}) {
return (
<div><h2>I am{person.name}.I am{person.age} years old.I know{
person.skill} < /h2>
</div >)
}
export default Person
Output:
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn