How to Loop Through an Array of Objects in React
- Loop Through an Array of Objects in JSX
-
Use
.map()
to Loop Through an Array of Objects in React -
Use
forEach()
to Loop Through an Array of Objects in React -
Use
for
to Loop Through an Array of Objects in React
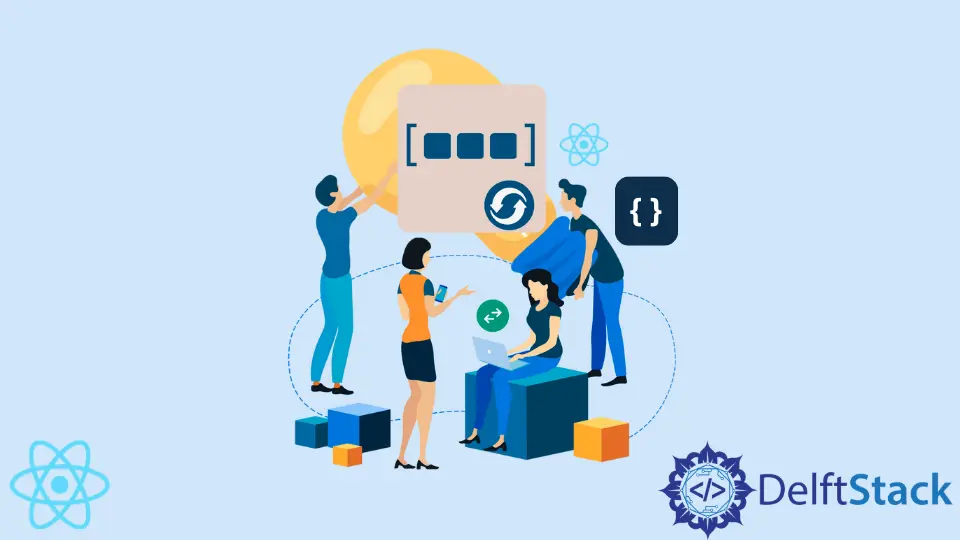
React is a popular front-end framework because it gives developers every feature needed to build fast, powerful web applications.
Loop Through an Array of Objects in JSX
JSX is the default templating language for React. It looks a lot like HTML, but in reality, it is simply a convenient way to write JavaScript code.
Because of this, React developers can use common JavaScript methods within JSX, as long as they use curly braces to mark JavaScript expressions.
Use .map()
to Loop Through an Array of Objects in React
Front-end developers usually need to build beautiful user interfaces to display data received from external sources. Data can be formatted in various ways.
However, if you have data of posts, product listings, or similar items, most likely, it will be formatted as an array of objects. Each object will contain information about the item: its title, main content, meta tags, head picture, and so on.
As a React developer, you can easily loop through an array of objects. You must use the .map()
JavaScript method, which goes over every item in the array and outputs each modified item.
Let’s take a look at an example:
export default function App() {
const postsArray = [
{
title: "How to Learn React",
content:
"React is built on JavaScript, so there is no escaping learning how to code in JavaScript. You need to know the basics of JavaScript and have a good knowledge of HTML and CSS to get started with React. "
},
{
title: "How to Learn Vue",
content:
"First, learn the essentials of Vue 2.0 by going through the main concepts and syntax. Then, build your first single-page app with Vue. "
},
{
title: "How to Learn HTML",
content:
"Codecademy has numerous free programs to provide you with the technical skills you need. ...Learn-HTML.org is a reliable source for everything you need to know about HTML."
}
];
return (
<div className="App">
<h1>Coding Blog</h1>
{postsArray.map((post) => {
return <Post post={post}></Post>;
})}
</div>
);
}
function Post(props) {
return (
<div>
<h1>{props.post.title}</h1>
<p>{props.post.content}</p>
</div>
);
}
Here, we have two components: the parent <App>
component and the child <Post>
component. In the parent component, we define a postsArray
variable to simulate external data you might receive from the API.
Our app is fairly simple: in the main <App>
component, we have a header for the blog. We also use the .map()
method to review each item in the array.
As we mentioned, the .map()
method allows us to output a modified version of each item. In our case, each item is an object that contains post data: its title and content.
We take each object and pass it down as props to the <Post>
component.
We access data received through props in the child component and display it as a header and a paragraph.
If you look at the live demo on CodeSandbox, you will see that our .map()
method does return three <Post>
components. One component for each object in the array.
It is important to look at the syntax of the .map()
function. We start by writing opening and closing curly braces so that we can reference a variable that contains the array.
Then we call the .map()
method on the array and provide its argument – a callback function to go over each item in the array. Finally, when we return JSX or HTML elements, we need to use data in each item of the array (which are objects) to fill the content of each returned element.
Use forEach()
to Loop Through an Array of Objects in React
The forEach()
array method offers an alternative to writing verbose for loops. You can use it in React as well.
forEach()
can not return HTML elements, but you can generate HTML elements and insert them into an array. Then you can reference that array within JSX to display the elements you created.
export default function App() {
const postsArray = [
{
title: "How to Learn React",
content:
"React is built on JavaScript, so there is no escaping learning how to code in JavaScript. You need to know the basics of JavaScript and have a good knowledge of HTML and CSS to get started with React. "
},
{
title: "How to Learn Vue",
content:
"First, learn the essentials of Vue 2.0 by going through the main concepts and syntax. Then, build your first single-page app with Vue. "
},
{
title: "How to Learn HTML",
content:
"Codecademy has numerous free programs to provide you with the technical skills you need. ...Learn-HTML.org is a reliable source for everything you need to know about HTML."
}
];
const posts = []
postsArray.forEach(post => posts.push(<div>
<h1>{post.title}</h1>
<p>{post.content}</p>
</div>)
)
return (
<div className="App">
<h1>Coding Blog</h1>
{posts}
</div>
);
}
Use for
to Loop Through an Array of Objects in React
The for
statement is the primary way to loop through an array of objects in JavaScript. However, you can not use it to render elements within JSX. For this reason, you should define a for
loop outside JSX.
For instance, let’s imagine we have the same array of posts as in the before example.
export default function App() {
const postsArray = [
{
title: "How to Learn React",
content:
"React is built on JavaScript, so there is no escaping learning how to code in JavaScript. You need to know the basics of JavaScript and have a good knowledge of HTML and CSS to get started with React. "
},
{
title: "How to Learn Vue",
content:
"First, learn the essentials of Vue 2.0 by going through the main concepts and syntax. Then, build your first single-page app with Vue. "
},
{
title: "How to Learn HTML",
content:
"Codecademy has numerous free programs to provide you with the technical skills you need. ...Learn-HTML.org is a reliable source for everything you need to know about HTML."
}
];
const posts = []
for (let i = 0; i < postsArray.length; i++){
posts.push(<div>
<h1>{postsArray[i].title}</h1>
<p>{postsArray[i].content}</p>
</div>)
}
return (
<div className="App">
<h1>Coding Blog</h1>
{posts}
</div>
);
}
In this example, we create HTML elements by iterating every item in the postArray
variable. We push HTML elements in the newly created posts
array.
We can not use the for
loop in JSX. Instead, we reference the posts
variable, which contains HTML elements we generated using data from the postArray
state variable.
Use the for...of
Syntax to Loop Through an Array of Objects in React
Developers can replace the for
loop in the previous example with the new, more readable syntax for looping through an array:
const posts = []
for (let post of postsArray){
posts.push(<div><h1>{post.title}</h1></div>)
}
This is a more readable approach. All other parts of the code can remain the same.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn