ReactDOM Render in React
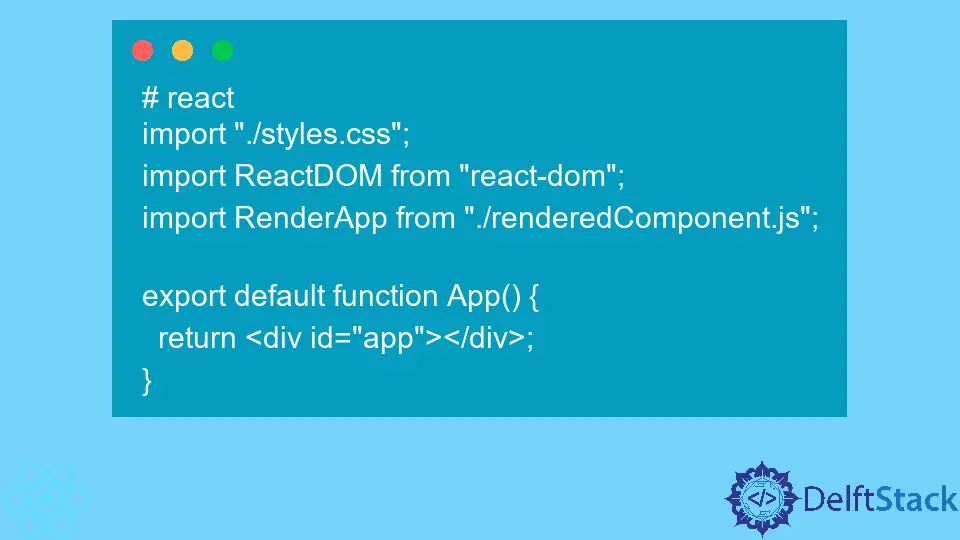
We will introduce what reactDOM.render
is and use it to render React components in React applications.
Use ReactDOM.render
to Render a Component in React
First, we will discuss the term render
in React. The term render
refers to sharing code between React components using a prop.
The value of that prop will be a function. React has two types of renders, namely render
and ReactDOM.render()
.
ReactDOM.render()
controls the content of the container nodes we pass in. We can replace the content of any DOM element using the ReactDom.render()
. It renders our components to the DOM while the component’s render returns the element that makes up the component.
Let’s have an example to understand how to render any component using ReactDom.render()
.
Let’s create a new React application and use ReactDom.render()
to render a component. We will run the command below to create a new app in React.
# react
npx create-react-app my-app
After creating our new application in React, we will go to our application directory using this command.
# react
cd my-app
Now, let’s run our app to check if all dependencies are installed correctly.
# react
npm start
Now, let’s create a new component, renderedComponent.js
, in our application, in which we will return an HTML element.
# react
export default function RenderApp() {
return <h1>I am Husnain</h1>;
}
Once we have created our component, we will go to App.js
and import ReactDOM
and the component we just created RenderApp
. And return a div
with an id app
.
# react
import "./styles.css";
import ReactDOM from "react-dom";
import RenderApp from "./renderedComponent.js";
export default function App() {
return <div id="app"></div>;
}
Now, using ReactDom.render
, we will render the component RenderApp
.
# react
ReactDOM.render(<RenderApp />, document.getElementById("app"));
Output:
In the above example, we have successfully rendered a React component using ReactDom.render()
. Using these simple steps, we can easily render any React component in another component.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn