How to Include React Variable in a String
- Include React Variable in a String Using String Concatenation
- Include React Variable in a String Using Template Literals
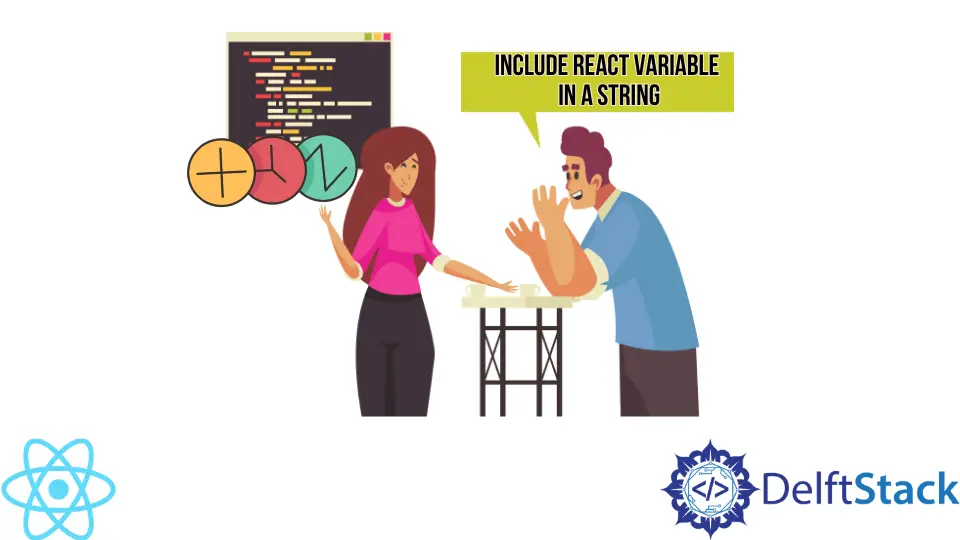
Today, React is probably the best library for building fast web applications with dynamic features. React uses a templating language JSX, similar to HTML; however, it has many advantages, like allowing us to execute JavaScript expressions as long as they’re wrapped with curly braces.
Include React Variable in a String Using String Concatenation
It is possible to generate the string using simple concatenation dynamically. You can concatenate multiple strings using the simple +
operator or the concat()
method.
For example, imagine you want to generate a dynamic className
string. Let’s imagine an example of how to generate a dynamic className
value using string concatenation:
export default function App() {
const [type, setType] = useState("large");
return (
<div className={type + "Box"}>
<h1>Hello CodeSandbox</h1>
<h2>Start editing to see some magic happen!</h2>
</div>
);
}
We initiate the type
state variable to a large
string. In JSX, we set the className
attribute of the <div>
element by concatenating the string value of type
variable (large
) and Box
string.
If we look at the source HTML, we will see that the class
of the container is largeBox
, as it should be.
We can use the setType
function to change the value of the type
state variable. The string value of className
will change as well.
This allows you to dynamically change the className
value of the container, enabling you to customize the component’s appearance.
You can use the same principle to concatenate more than two strings. If you need spaces between words, make sure to add them manually:
<h1>{firstWord + " " + secondWord}</h1>
Note: To execute JavaScript expressions within JSX, we must wrap them with curly braces.
A live demo of this example is available on CodeSandbox, so you can check out how concatenation works yourself.
Include React Variable in a String Using Template Literals
Many front-end prefer to use more elegant template literals to feature variables in a string. They look like normal strings; the only difference is that instead of using single or double quotes, we use backticks (``) to mark template literals.
They also allow you to embed expressions within the string, using the dollar sign and curly braces. This feature was introduced in ES6, so it’s a relatively new addition to JavaScript.
Let’s look at the first example again, but this time we use template literals to include a variable in the string:
export default function App() {
const [type, setType] = useState("large");
return (
<div className={`${type}Box`}>
<h1>Hello CodeSandbox</h1>
<h2>Start editing to see some magic happen!</h2>
</div>
);
}
In this case, the type
variable will be substituted with its string value. Ultimately, we will still have a largeBox
string value for the className
attribute.
Template literals are often more readable than simple concatenation. For example, putting spaces between words is much easier when you have many variables in the string.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn