String Interpolation in React
- What is String Interpolation?
- Method 1: Using Template Literals
- Method 2: String Interpolation with JSX
- Method 3: Interpolating Strings in Function Calls
- Conclusion
- FAQ
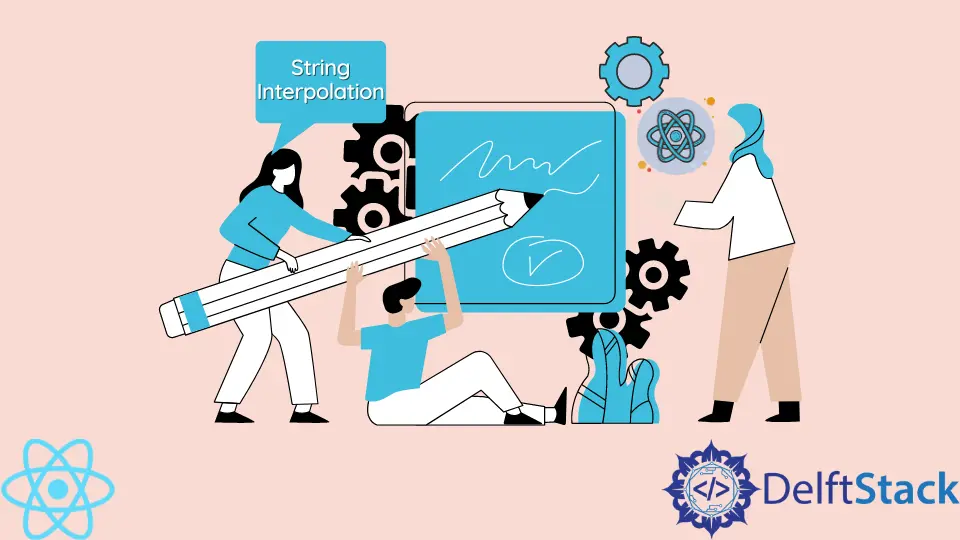
String interpolation is a powerful feature that allows developers to seamlessly integrate dynamic values into strings. In the context of React, a popular JavaScript library for building user interfaces, string interpolation enhances the readability and maintainability of code. Whether you’re working with component properties, state variables, or dynamic content, understanding how to effectively use string interpolation can significantly improve your React applications.
In this article, we will explore various methods of string interpolation in React, providing clear examples and detailed explanations to help you grasp this essential concept.
What is String Interpolation?
String interpolation refers to the process of inserting dynamic values into a string without the need for cumbersome concatenation. In React, this is particularly useful for rendering JSX, where you often need to display variables, expressions, or function results within your components. By leveraging template literals and JSX syntax, developers can create cleaner and more readable code. Let’s delve into some practical methods of achieving string interpolation in React.
Method 1: Using Template Literals
Template literals are a feature of ES6 that allow for embedded expressions in strings. They are defined using backticks (`) and can include placeholders for variables or expressions, which are indicated by the dollar sign and curly braces (${expression}). This method is particularly effective in React for rendering dynamic content.
Here’s a simple example demonstrating how to use template literals in a React component:
import React from 'react';
const UserGreeting = ({ name }) => {
return <h1>{`Hello, ${name}! Welcome back.`}</h1>;
};
export default UserGreeting;
In this example, the UserGreeting
component takes a name
prop and uses a template literal to create a personalized greeting. The backticks allow for the inclusion of the name
variable directly within the string.
Output:
Hello, John! Welcome back.
This approach enhances readability by eliminating the need for traditional string concatenation. Instead of writing something like “Hello, " + name + “! Welcome back.”, template literals make it clear and concise. Additionally, they support multi-line strings, which can be beneficial for formatting larger blocks of text.
Method 2: String Interpolation with JSX
In React, you can also use string interpolation directly within JSX. This method allows you to embed JavaScript expressions inside curly braces within your JSX code. It’s a straightforward way to render dynamic values alongside static content.
Here’s how you can implement this in a React component:
import React from 'react';
const WeatherReport = ({ temperature, city }) => {
return (
<div>
<h2>Weather Report</h2>
<p>{`The current temperature in ${city} is ${temperature}°C.`}</p>
</div>
);
};
export default WeatherReport;
In this example, the WeatherReport
component receives temperature
and city
as props. The string interpolation occurs within the <p>
tag, allowing the dynamic values to be rendered seamlessly.
Output:
The current temperature in London is 22°C.
This method is particularly useful when you want to combine static text with dynamic data, making your components more informative and engaging. Using curly braces to embed expressions also keeps your JSX clean and easy to read.
Method 3: Interpolating Strings in Function Calls
Another effective way to use string interpolation in React is by incorporating it within function calls. This can be useful when you want to format strings or perform calculations before rendering them in your component.
Here’s an example that demonstrates this concept:
import React from 'react';
const formatGreeting = (name) => {
return `Hello, ${name}!`;
};
const Greeting = ({ name }) => {
return <h1>{formatGreeting(name)}</h1>;
};
export default Greeting;
In this example, the formatGreeting
function takes a name
parameter and returns a formatted greeting string. The Greeting
component then calls this function, passing the name
prop to it.
Output:
Hello, Sarah!
By using a function for string interpolation, you can encapsulate complex logic or formatting rules outside of your JSX. This not only keeps your components clean but also promotes reusability and separation of concerns in your code.
Conclusion
String interpolation is an essential skill for any React developer. By utilizing methods like template literals, JSX embedding, and function calls, you can create dynamic, readable, and maintainable code. These techniques not only enhance the user experience but also streamline the development process. As you continue to build more complex applications, mastering string interpolation will undoubtedly elevate your coding capabilities and improve the overall quality of your projects.
FAQ
-
What is string interpolation in React?
String interpolation in React refers to the technique of embedding dynamic values into strings, enhancing readability and maintainability in your components. -
How do I use template literals in React?
Template literals are used by enclosing strings in backticks () and including variables or expressions within
${}` placeholders. -
Can I use string interpolation with JSX?
Yes, you can embed JavaScript expressions directly within JSX using curly braces, allowing for dynamic content rendering alongside static text. -
Is there a way to format strings before rendering in React?
Yes, you can create functions to format strings and call them within your components, keeping your JSX clean and organized. -
Why is string interpolation important in React development?
String interpolation improves code readability, reduces complexity, and enhances user experience by allowing for dynamic and personalized content display.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn