super vs super(props) in React
-
Difference Between
super
andsuper(props)
in React -
Use the
super()
Function in React -
Use the
super(props)
Function in React
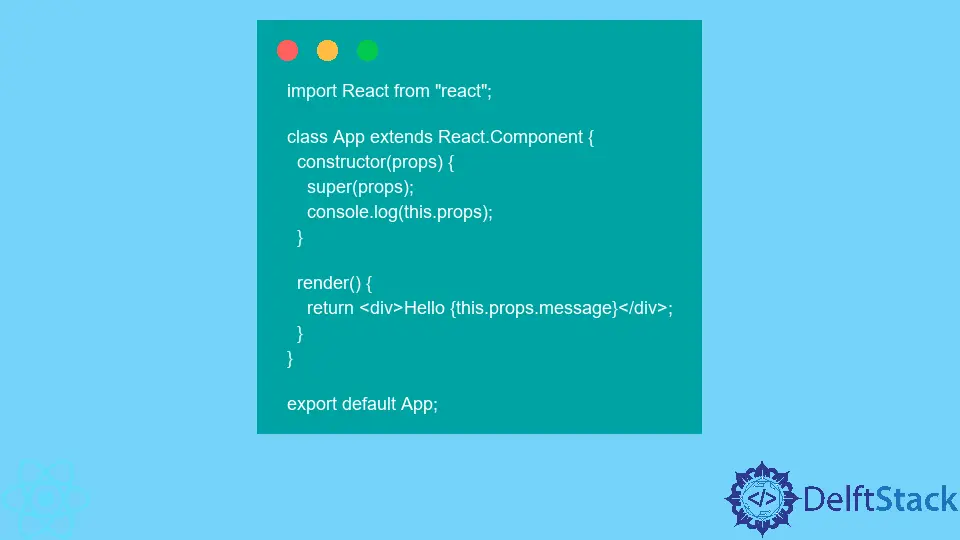
We will introduce super()
and super(props)
in React and explain the difference between both of them using examples.
Difference Between super
and super(props)
in React
We often need to use super()
and super(props)
when building a web app in React, but some developers do not know the difference between them or when to use which one.
Before going into detail about the differences between them, we will first understand what super()
and props
are.
We use the super()
function when we need to access some variables of the parent class. It is used to call the constructor of its parent class to access variables.
On the other hand, props
is a special keyword in React used for passing data from one component to another. The data that props contain is read-only.
Because of that, the data that comes from a parent cannot be changed in a child component.
Now, let’s have an example to understand super()
and super(props)
. Let’s create a new application using the following command.
# react
npx create-react-app my-app
After creating our new application in React, we will go to our application directory using this command.
# react
cd my-app
Run the app to check if all dependencies are installed correctly.
# react
npm start
Use the super()
Function in React
We will create a simple component that will demonstrate the super()
function. In App.js
, we will extend the class App
with React.Component
.
Inside our class, we will define a constructor with props
. Inside our constructor, we will call super()
and log props
and this.props
in the console to check what output we get for both.
So, our code in App.js
will look like below.
import React from "react";
class App extends React.Component {
constructor(props) {
super();
console.log(this.props);
console.log(props);
}
render() {
return <div>Hello {this.props.message}</div>;
}
}
export default App;
Output:
As shown above, when we are not using props in super()
and console.log(this.props)
, we will get an undefined message because we are using this.props
inside the constructor. But when we console.log(props)
, it gives us a proper message meaning that it is defined.
Use the super(props)
Function in React
Let’s look at the super(props)
example. Let’s create a new application using the following command.
# react
npx create-react-app my-app
After creating our new application in React, we will go to our application directory using this command.
# react
cd my-app
Run the app to check if all dependencies are installed correctly.
# react
npm start
We will create a simple component that will demonstrate the use of the super(props)
function. In App.js
, we will extend the class App
with React.Component
.
Inside our class, we will define a constructor with props
. Inside our constructor, we will call super(props)
and log this.props
in the console to check what output we get.
So, our code in App.js
will look like below.
import React from "react";
class App extends React.Component {
constructor(props) {
super(props);
console.log(this.props);
}
render() {
return <div>Hello {this.props.message}</div>;
}
}
export default App;
Output:
As shown above, if we want to use this
in the constructor, we must pass it to super. So if we want to use this.props
inside the constructor, we need to pass the props
to super()
.
But if we don’t want to use it, we can only use super()
. And from the above example, we also learned that inside the render function, it doesn’t matter if we have passed props
to super()
or not because this.props
are available in render function whether we pass props to super()
or not.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn