setState and prevState in React
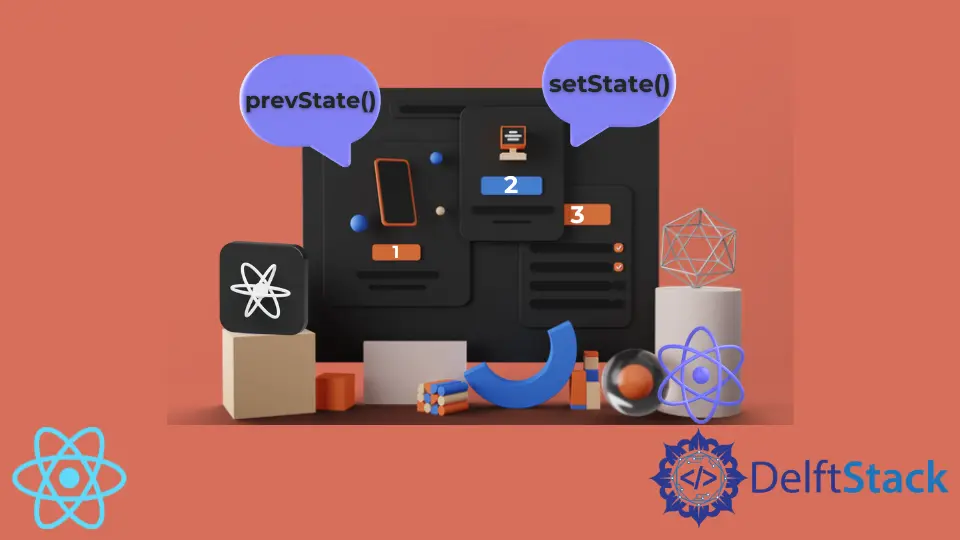
We will introduce setState
and prevState
and use them in React.
setState
and prevState
in React
setState()
and prevState()
are useState
hooks that are used to change state in a React class component.
setState()
indicates that this component and its children components are changed and need to be re-rendered with the updated state. setState
is the primary method used to update the UI in response to event handlers and server responses.
prevState()
is the same as the setState
but the only difference between them is that if we want to change the state of a component based on the previous state of that component, we use this.setState()
, which provides us the prevState
.
Let’s check an example of a counter app. That can increment, decrement, reset numbers.
First of all, we will create a new file, Counter.js
. In Counter.js
we will import React from "react";
and create a function called Counter()
.
In Counter()
we will initialize our constants initialNum
, num
, and setNum
# react
import React from "react";
function Counter() {
const initialNum = 0;
const [num, setNum] = React.useState(initialNum);
}
We will return the HTML with buttons and a counter display. So, Counter.js
will look like below.
# react
import React from "react";
function Counter() {
const initialNum = 0;
const [num, setNum] = React.useState(initialNum);
};
return (
<div>
<p>Counter: {num}</p>
<button onClick={() => setNum(initialNum)}>Reset</button>
<button onClick={() => setNum(num + 1)}>Increment</button>
<button onClick={() => setNum(num - 1)}>Decrement</button>
</div>
);
}
export default Counter;
Let’s include Counter.js
in App.js
and add its component. App.js
will look like below.
# react
import "./styles.css";
import Counter from "./Counter.js";
export default function App() {
return (
<div className="App">
<Counter />
</div>
);
}
Now, let’s test our counter.
Output:
Let’s add another button that will increment the counter by five.
# react
<button onClick={IncrementByFive}>Increment By 5</button>
Let’s create a function IncrementByFive
.
# react
const IncrementByFive = () => {
for (let i = 0; i < 5; i++) {
setNum(num + 1);
}
};
This function will loop for five times to increment it by 5.
Now, let’s test our counter app.
Output:
When we click on the Increment by 5
button, it only increments the counter by 1.
It is because in setNum(num + 1);
there are multiple setNum
calls, so React grouped all these calls and updates the state only in the last call of setNum
, and because in the last call setNum
is not yet updated and still have the same value, so it only increments it by 1.
That’s when prevState
comes in handy and solves our problems like a champ. Now, let’s rewrite our counter app using prevState
.
Basically, instead of passing in a value of the new state variable, we pass in a function that has access to the old state value. So, now we will change IncrementByFive
function by changing setNum(num + 1);
to setNum(prevNum => prevNum + 1);
.
So, our function will look like below.
# react
const IncrementByFive = () => {
for (let i = 0; i < 5; i++) {
setNum(prevNum => prevNum + 1);
}
};
Now, let’s test our counter app.
Output:
In the above result, when we click on the Increment by 5
, it increments it by 5.
Let’s correct our other buttons as well using prevState
.
Counter.js
will look like below.
# react
import React from "react";
function Counter() {
const initialNum = 0;
const [num, setNum] = React.useState(initialNum);
const IncrementByFive = () => {
for (let i = 0; i < 5; i++) {
setNum((prevNum) => prevNum + 1);
}
};
return (
<div>
<p>Counter: {num}</p>
<button onClick={() => setNum(initialNum)}>Reset</button>
<button onClick={() => setNum((prevNum) => prevNum + 1)}>
Increment
</button>
<button onClick={() => setNum((prevNum) => prevNum - 1)}>
Decrement
</button>
<button onClick={IncrementByFive}>Increment By 5</button>
</div>
);
}
export default Counter;
So, in this guide, we learned about the issues which can be resolved using prevState
, and we also built a basic counter app in React using setState
and prevState
.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn