How to Set Scroll Position in React
- Set Scroll Position in React
-
Use the
scrollTo()
to Set Scroll Position in React -
Use the
scrollIntoView()
to Set Scroll Position in React - Conclusion
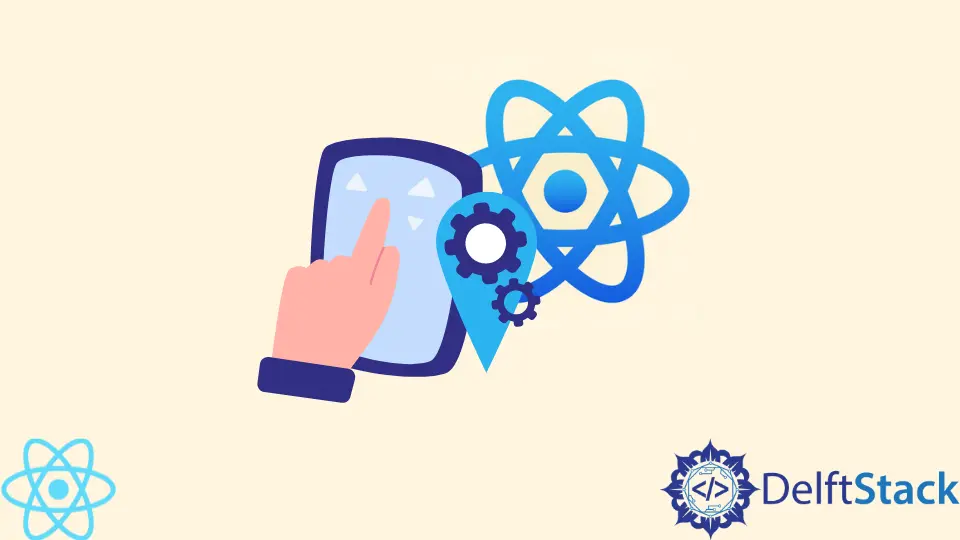
Web applications often present users with extensive content, making manual scrolling cumbersome. Scrolling is a fundamental aspect of web navigation, but it doesn’t have to be a one-size-fits-all experience.
In React, there are various methods to gain precise control over scroll positions, allowing developers to enhance user interaction and create a more tailored user experience.
This article explores the different techniques to set a fixed scroll position in React, providing a more user-friendly interface.
Set Scroll Position in React
In order to control the scroll position in React, it is important to understand the useRef()
hook. It excels at creating references to DOM elements, facilitating direct manipulation and interaction.
Think of it as a way to store a link to an element, similar to how getElementById()
works in regular JavaScript. This reference helps us manipulate and manage the scroll position effectively.
Take a look at the example code below:
import { useRef } from "react";
export default function App() {
const welcome = useRef(null);
const about = useRef(null);
const terms = useRef(null);
return (
<div className="App">
<h1 ref={welcome}>Welcome to Web Application</h1>
<h1 ref={about}>About Us</h1>
<h1 ref={terms}>Terms and Conditions</h1>
</div>
);
}
This React code uses the useRef
hook to create references (welcome
, about
, and terms
) for three heading elements in a web application. These references establish a link between the React component and the HTML headings, allowing for dynamic content updates or programmatic interactions.
This understanding of the useRef
hook is essential to start setting a scroll position in React using various methods.
Use the scrollTo()
to Set Scroll Position in React
The scrollTo()
method of the window
interface is natively available in JavaScript libraries, including React. This approach allows developers to dynamically set the scroll position with accuracy.
Consider the full sample code below:
import { useRef } from "react";
export default function App() {
const welcome = useRef(null);
const about = useRef(null);
const terms = useRef(null);
const setScrollPosition = (ref) => {
window.scrollTo({
top: ref.current.offsetTop,
behavior: "smooth",
});
};
return (
<div className="App">
<h1 ref={welcome}>Welcome to Web Application</h1>
<h1 ref={about}>About Us</h1>
<h1 ref={terms}>Terms and Conditions</h1>
<div className="buttons">
<button onClick={() => setScrollPosition(welcome)}>
Skip to Welcome
</button>
<button onClick={() => setScrollPosition(about)}>
Skip to 'About Us'
</button>
<button onClick={() => setScrollPosition(terms)}>Skip to terms</button>
</div>
</div>
);
}
Open this live CodeSandBox demo to see how the application works.
The React code above employs the useRef
hook to create references (welcome
, about
, and terms
) for three heading elements in a web application. These references serve as connections between the React component and the corresponding HTML elements, enabling dynamic interaction and manipulation.
The setScrollPosition
function is crucial in this setup. It takes a reference (ref
) as its parameter.
When invoked by the buttons, it utilizes the native window.scrollTo()
method to smoothly scroll to the specified element’s top position. The behavior: "smooth"
option ensures a visually pleasing transition during the scroll.
The JSX render method structures the user interface, featuring the three headings and a set of buttons within a div
with the class name buttons
.
Each button, when clicked, triggers the setScrollPosition
function with the respective heading’s reference, offering users a convenient way to navigate directly to specific sections of the webpage.
In summary, this code enhances user experience by providing a straightforward mechanism for users to skip to different sections of the web application with smooth scrolling.
The combination of useRef
and the window.scrollTo()
method allows for precise control over scroll behavior, making the web application more interactive and user-friendly.
Use the scrollIntoView()
to Set Scroll Position in React
The scrollIntoView()
method is a native JavaScript function that seamlessly integrates with React applications.
It is known for its simplicity and efficiency. It offers a precise way to guide users to specific elements, enhancing the overall navigational experience.
This section explores the intricacies of using scrollIntoView()
to set scroll positions in React components.
Consider the full sample code below:
import { useRef } from "react";
export default function App() {
const welcome = useRef(null);
const about = useRef(null);
const terms = useRef(null);
const setScrollPosition = (ref) => {
ref.current.scrollIntoView({ behavior: "smooth" });
};
return (
<div className="App">
<h1 ref={welcome}>Welcome to Web Application</h1>
<h1 ref={about}>About Us</h1>
<h1 ref={terms}>Terms and Conditions</h1>
<div className="buttons">
<button onClick={() => setScrollPosition(welcome)}>
Skip to Welcome
</button>
<button onClick={() => setScrollPosition(about)}>
Skip to 'About Us'
</button>
<button onClick={() => setScrollPosition(terms)}>Skip to terms</button>
</div>
</div>
);
}
Open this live CodeSandBox demo to see how the application works.
The React code above defines a web application component using the useRef
hook, a handy tool for creating references to specific HTML elements.
In this case, three references — welcome
, about
, and terms
— are created for the corresponding heading elements.
The setScrollPosition
function takes a reference as an argument and uses it to scroll smoothly to the associated section. When a button is clicked, it calls this function with the relevant reference, allowing users to skip to specific sections of the page.
The JSX part renders three heading elements with content like "Welcome to Web Application"
, "About Us"
, and "Terms and Conditions"
. Additionally, there’s a set of buttons in a div
, each linked to a particular section through the setScrollPosition
function.
In essence, this code offers a straightforward way to implement smooth scrolling to different sections of a webpage, enhancing user experience and navigation. Users can click buttons to swiftly jump to the welcome message, the About Us
section, or the terms and conditions, thanks to the use of useRef
and the setScrollPosition
function.
Conclusion
In conclusion, this article has explored fundamental techniques to establish a fixed scroll position in React, shedding light on two key methods: scrollTo()
and scrollIntoView()
.
By understanding and implementing these methods, developers now possess versatile tools to precisely control scroll behavior within their React applications. Whether opting for the direct positioning offered by scrollTo()
or the element-focused approach of scrollIntoView()
, both methods empower developers to create a seamless and user-friendly navigation experience.
Mastering these scroll control methods will undoubtedly contribute to the overall effectiveness and responsiveness of any web application.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn