How to Implement Horizontal Scroll of Cards in React
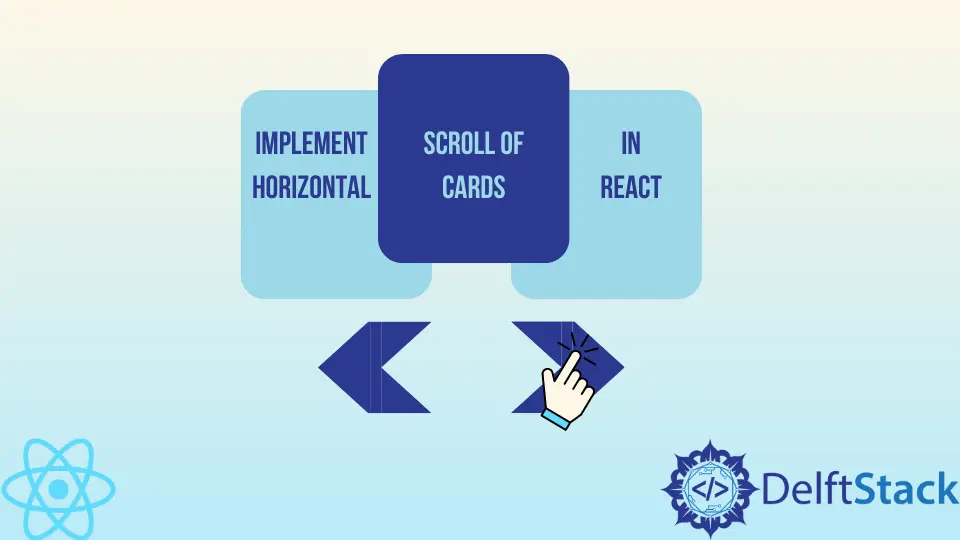
It’s technically possible to build anything with just Vanilla JavaScript. Frameworks like React add a structure to the core JavaScript language, which helps you easily build web applications.
React also has a large community of developers who create custom components and share them with everyone.
Developers in React community have created many packages which allow you to add almost all features you might need in a React app. You can use these custom components without understanding how they work.
This article will discuss creating a carousel of horizontally scrollable cards in React.
React Horizontal Scroll Cards
You can create a custom React component for horizontally scrollable cards. However, it will take too much time and might not work as well as ready-made packages.
In this article, we will use the package called react-spring-3d-carousel
.
We must import the custom Carousel
component from the react-spring-3d-carousel
package. Thanks to this component, implementing horizontal scroll cards is very easy.
All you have to do is provide a list of DOM elements displayed in the carousel.
Let’s look at an example.
import "./styles.css";
import React, { Component } from "react";
import Carousel from "react-spring-3d-carousel";
import SampleCard from "./card";
import { config } from "react-spring";
class ExampleCarousel extends Component {
constructor(props) {
super(props);
this.state = {
changeSlide: ""
};
}
slides = [
{
key: 1,
content: <SampleCard />
},
{
key: 2,
content: <SampleCard />
},
{
key: 3,
content: <SampleCard />
},
{
key: 4,
content: <SampleCard />
},
{
key: 5,
content: <SampleCard />
},
{
key: 6,
content: <SampleCard />
},
{
key: 7,
content: <SampleCard />
},
{
key: 8,
content: <SampleCard />
}
].map((slide, index) => {
return { ...slide, onClick: () => this.setState({ changeSlide: index }) };
});
render() {
return (
<div style={{ width: "40%", height: "500px", margin: "0 auto" }}>
<Carousel
slides={this.slides}
changeSlide={this.state.changeSlide}
offsetRadius="10"
animationConfig={config.gentle}
showNavigation
/>
</div>
);
}
}
export default ExampleCarousel;
Here, we have a list (array) of cards. Each object in the list has two properties: key
and content
.
Values of the key
property need to be unique. The value of the content
property will be displayed in the carousel.
Then we apply the .map()
method to set the onClick()
event handler for each card in the carousel. The .map()
method generates a new array of objects, which we will store in the slides
variable.
We will reference this list to display a carousel in JSX.
<Carousel
slides={this.slides}
changeSlide={this.state.changeSlide}
animationConfig={config.gentle}
showNavigation
/>
First, we set the slides
attribute to the array of objects. We also set the changeSlide
to the current index of the card currently in focus.
We also set the animationConfig
attribute to a smooth transition. This is only possible because we imported the config
module from the react-spring
package.
The List of Cards in the Scroll
Let us look at the list of cards stored in the slides
property.
slides = [
{
key: 1,
content: <SampleCard />
},
{
key: 2,
content: <SampleCard />
},
{
key: 3,
content: <SampleCard />
},
{
key: 4,
content: <SampleCard />
},
{
key: 5,
content: <SampleCard />
},
{
key: 6,
content: <SampleCard />
},
{
key: 7,
content: <SampleCard />
},
{
key: 8,
content: <SampleCard />
}
].map((slide, index) => {
return { ...slide, onClick: () => this.setState({ changeSlide: index }) };
});
Each object in the slides list has a content
property, which has a value of the <SampleCard />
component. The carousel will create a horizontal scroll of content that we specify here.
For example, you can specify a square <div>
element, and the carousel will display them.
You can also set different components for each object’s content
property in the array, and the carousel will display different cards. In this case, we set a <SampleCard />
component to all the objects in the array.
We can also pass props
to the component to customize its behavior, functionality, and appearance. Read official React documentation to learn about possible use cases for props
.
Let’s look at the code of the <SampleCard>
component.
const imageUrl =
'https://play-lh.googleusercontent.com/yPtnkXQAn6yEahOurxuYZL576FDXWn3CqewVcEWJsXlega_nSiavBvmaXwfTGktGlQ';
export default class SampleCard extends React.Component {
render = () => {
return (
<div>
<img src={imageUrl} height='150' width='150'></img>
<h1>Card title</h1>
</div>
);
};
}
If you open this live demo on CodeSandbox, you’ll see each a horizontal scroll of these cards. The structure of these cards is fairly simple - we have an image and an <h1>
text.
The image is just an example of how a card image would look. You can replace the imageUrl
variable with a link to any other image, and you will see the changes.
Add elements and styles to the main <div>
container returned by the SampleCard
component to create a more complex card.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn