How to Store Data in Session Storage in React
- Understanding Session Storage
- Setting Up a React Application
- Storing Data in Session Storage
- Retrieving Data from Session Storage
- Clearing Data from Session Storage
- Conclusion
- FAQ
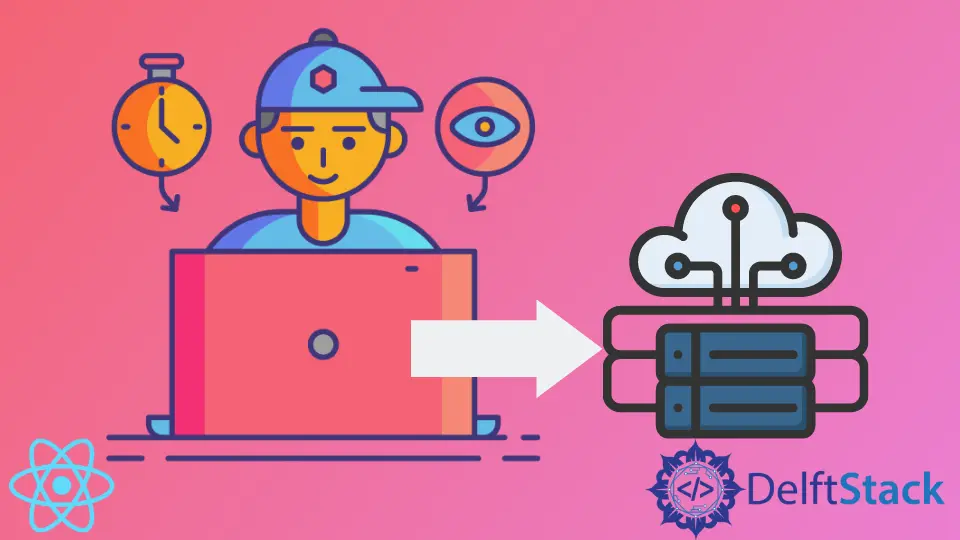
Storing data in session storage is a common practice in web development, especially when working with React applications. Session storage allows developers to store data temporarily for the duration of a page session, making it an excellent choice for scenarios where you need to preserve user data without relying on a backend database.
In this tutorial, we will explore how to effectively use session storage in React to manage state and enhance user experience. We’ll cover essential methods, provide clear code examples, and ensure that you have a solid understanding of this powerful feature. Whether you’re building a small app or a large-scale project, knowing how to manage session storage can significantly improve your application’s functionality.
Understanding Session Storage
Before diving into the implementation, let’s clarify what session storage is. Session storage is a web storage feature that allows you to store data in a browser for the duration of the page session. This means that the data persists as long as the browser tab is open. Once the tab is closed, the data is cleared. This is particularly useful for scenarios where you need to store user preferences, form data, or temporary information that doesn’t require long-term storage.
Session storage is similar to local storage but has a key difference: while local storage persists even after the browser is closed, session storage is limited to the current tab. This makes it ideal for data that doesn’t need to be retained beyond the session.
Setting Up a React Application
To begin, you’ll need a React application. If you don’t have one set up yet, you can create a new project using Create React App. Open your terminal and run the following command:
npx create-react-app session-storage-demo
cd session-storage-demo
npm start
This will create a new React application and start a local development server. Once your setup is complete, you can start implementing session storage.
Storing Data in Session Storage
Now that your React application is ready, let’s look at how to store data in session storage. You can use the sessionStorage
object, which is built into the browser, to achieve this. Here’s a simple example:
import React, { useState } from 'react';
function App() {
const [name, setName] = useState('');
const handleSubmit = (e) => {
e.preventDefault();
sessionStorage.setItem('username', name);
alert('Data stored in session storage!');
};
return (
<div>
<h1>Store Data in Session Storage</h1>
<form onSubmit={handleSubmit}>
<input
type="text"
value={name}
onChange={(e) => setName(e.target.value)}
placeholder="Enter your name"
/>
<button type="submit">Submit</button>
</form>
</div>
);
}
export default App;
In this code, we create a simple form where users can input their name. When the form is submitted, the handleSubmit
function is triggered. This function uses sessionStorage.setItem
to store the name entered by the user under the key “username.” An alert confirms that the data has been stored.
Output:
Data stored in session storage!
The sessionStorage.setItem
method takes two arguments: a key and a value. The key is a string that identifies the data, and the value can be any string. If you need to store objects or arrays, you can convert them to JSON format using JSON.stringify()
before storing them.
Retrieving Data from Session Storage
Once you have stored data in session storage, you may want to retrieve it later. This can be achieved using the sessionStorage.getItem
method. Here’s how to do that:
import React, { useState, useEffect } from 'react';
function App() {
const [name, setName] = useState('');
useEffect(() => {
const storedName = sessionStorage.getItem('username');
if (storedName) {
setName(storedName);
}
}, []);
return (
<div>
<h1>Welcome, {name ? name : 'Guest'}!</h1>
</div>
);
}
export default App;
In this example, we use the useEffect
hook to retrieve the stored name when the component mounts. The sessionStorage.getItem
method is called with the key “username,” and if a value is found, it updates the state variable name
. This allows us to greet the user with their name if it exists in session storage.
Output:
Welcome, [username]!
Using session storage in this way enhances the user experience by personalizing the greeting based on previously entered data. If the user has not entered their name, it defaults to “Guest.”
Clearing Data from Session Storage
Sometimes, you may want to clear specific items or all data from session storage. You can use sessionStorage.removeItem
to remove a specific item, or sessionStorage.clear
to clear all stored data. Here’s an example of how to implement this in your React application:
import React, { useState } from 'react';
function App() {
const [name, setName] = useState('');
const handleClear = () => {
sessionStorage.removeItem('username');
setName('');
alert('Data cleared from session storage!');
};
return (
<div>
<h1>Store Data in Session Storage</h1>
<button onClick={handleClear}>Clear Data</button>
</div>
);
}
export default App;
In this code, we create a button that, when clicked, will remove the stored name from session storage using sessionStorage.removeItem
. It also updates the state variable name
to an empty string, effectively clearing the displayed name.
Output:
Data cleared from session storage!
This method is particularly useful for scenarios where you want to reset user data or allow users to log out of your application.
Conclusion
Storing data in session storage in React is straightforward and can significantly enhance your application’s interactivity. By utilizing the built-in sessionStorage
API, you can easily save, retrieve, and clear data as needed. This tutorial covered the essential methods for managing session storage, including how to store user input, retrieve it for display, and clear it when necessary. With these techniques, you can build more dynamic and user-friendly React applications.
FAQ
-
What is session storage?
Session storage is a web storage feature that allows you to store data temporarily in the browser for the duration of a page session. -
How long does data stay in session storage?
Data in session storage persists as long as the browser tab is open. Once the tab is closed, the data is cleared. -
Can I store objects in session storage?
Yes, you can store objects in session storage by converting them to a JSON string usingJSON.stringify()
. -
How do I clear session storage?
You can clear session storage usingsessionStorage.clear()
to remove all data orsessionStorage.removeItem(key)
to remove a specific item. -
Is session storage secure?
Session storage is not secure for sensitive data, as it can be accessed by any script on the page. Avoid storing sensitive information.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn