How to Read JSON Data From External Files in React
- Read JSON Data From External Files in React
-
Use the
import
Statement to Read JSON Data From External Files in React -
Use the
require()
Function to Read JSON Data From External Files in React
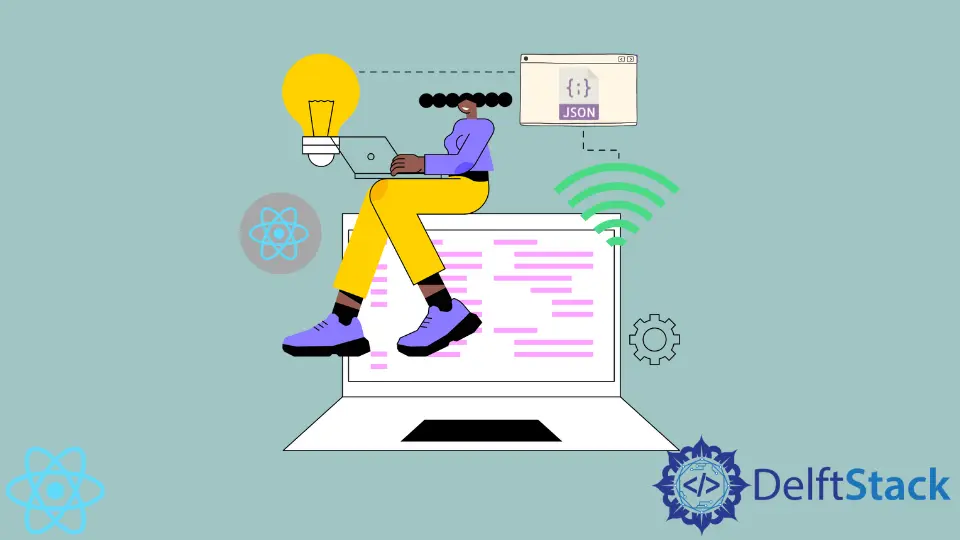
JSON is the most practical format to exchange data on the web. Web applications built in React usually fetch external data in JSON format.
Without external data, applications would not be very useful or dynamic. Usually, the data source is API, but it can also be an external file.
This article will explore how to read data from JSON files in React.
Read JSON Data From External Files in React
It’s fairly easy to access the data from a JSON file in React. In JSX, you can use the .map()
method to go over the array and display a piece of information from every object in the array.
For that, you’re going to need curly braces {}
, which will allow you to use JavaScript expressions to produce elements such as <h1>
.
Let’s explore two ways to do that in React.
Use the import
Statement to Read JSON Data From External Files in React
You can use a simple import
statement to make external JSON data available in your React component.
The import
statement creates a live binding. In other words, if you change any part of the data in the original file, the values in your application will be updated as well.
Example:
import { stockData } from "./data";
export default function App() {
return (
<div className="App">
{stockData.map((data, key) => {
return <h1 key={key}>{data.company}</h1>;
})}
</div>
);
}
We imported the stockData
variable from the data.json
file, containing an object in JSON format.
Then we used the curly braces to call the .map()
method on the stockData
array and displayed values from each item in the array as an <h1>
element.
Check out this live demo on CodeSandbox. Try to change something in the source data, and see if anything changes in your React app.
Use the require()
Function to Read JSON Data From External Files in React
There is another way to read external JSON data in your React components using the require()
function.
Example:
var data = require("./moredata.json");
export default function App() {
return (
<div className="App">
{stockData.map((data, key) => {
return <h1 key={key}>{data.company}</h1>;
})}
<h2>The name is: {data[0].name}</h2>
</div>
);
}
In this case, we read the data from the moredata.json
file and store it in the data
variable.
Then we created an <h2>
element. Between the opening and closing tags, we placed a reference to the data we loaded.
We read the name
property of the first object of the array. As you can see on live demo, it works.
Possible Errors
The recent versions of Webpack support this feature. If you get errors, consider installing an external loader such as json-loader
.
Check it out on npm.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn