How to Display JSON in React
- Understanding JSON and Its Role in React
- Method 1: Fetching JSON Data from an API
- Method 2: Displaying Local JSON Files
- Method 3: Handling Nested JSON Data
- Conclusion
- FAQ
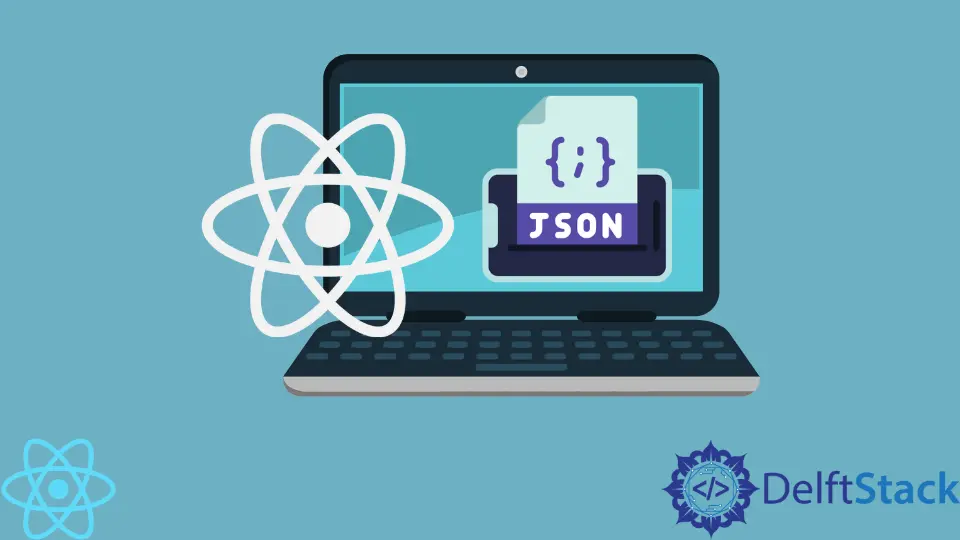
Displaying JSON-formatted data in a React application is a common task that many developers encounter. Whether you’re fetching data from an API or working with local files, understanding how to effectively render this data can significantly enhance your application’s functionality and user experience.
In this article, we will explore various methods to display JSON in React, providing clear examples and explanations. By the end of this guide, you’ll be equipped with the knowledge to seamlessly integrate JSON data into your React components, making your application more dynamic and responsive.
Understanding JSON and Its Role in React
JSON, or JavaScript Object Notation, is a lightweight data interchange format that is easy for humans to read and write. It is also easy for machines to parse and generate. In the context of React, JSON is often used to represent data fetched from APIs or to structure data that your application needs to display. React’s component-based architecture makes it simple to render this data dynamically, allowing for a rich user experience.
Method 1: Fetching JSON Data from an API
One of the most common ways to display JSON in React is by fetching it from an API. This method involves using the fetch
function to retrieve data and then storing it in the component’s state. Here’s how you can do it:
import React, { useEffect, useState } from 'react';
const App = () => {
const [data, setData] = useState([]);
useEffect(() => {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(json => setData(json))
.catch(error => console.error('Error fetching data:', error));
}, []);
return (
<div>
<h1>Data from API</h1>
<ul>
{data.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
</div>
);
};
export default App;
Output:
Data from API
- Item 1
- Item 2
- Item 3
In this example, we use the useEffect
hook to fetch data when the component mounts. The fetch
function retrieves data from the specified API endpoint. Once the data is fetched and converted to JSON, it is stored in the component’s state using the setData
function. The data is then rendered as a list of items. This approach is efficient and leverages React’s capabilities to manage state and lifecycle events.
Method 2: Displaying Local JSON Files
Sometimes, you might want to display data from a local JSON file instead of fetching it from an external API. This can be especially useful during development or when working with static data. Here’s how to do it:
import React, { useEffect, useState } from 'react';
import data from './data.json';
const App = () => {
const [items, setItems] = useState([]);
useEffect(() => {
setItems(data);
}, []);
return (
<div>
<h1>Local JSON Data</h1>
<ul>
{items.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
</div>
);
};
export default App;
Output:
Local JSON Data
- Item A
- Item B
- Item C
In this example, we import a local JSON file named data.json
. The useEffect
hook is used to set the items from the JSON file into the component’s state. This method is straightforward and allows for quick access to static data without the need for an API call. It is particularly useful in scenarios where the data does not change frequently.
Method 3: Handling Nested JSON Data
When working with JSON, you may encounter nested structures. Displaying nested data in React requires a slightly different approach. Let’s take a look at how to handle this situation:
import React, { useEffect, useState } from 'react';
const App = () => {
const [data, setData] = useState([]);
useEffect(() => {
fetch('https://api.example.com/nested-data')
.then(response => response.json())
.then(json => setData(json))
.catch(error => console.error('Error fetching data:', error));
}, []);
return (
<div>
<h1>Nested JSON Data</h1>
{data.map(item => (
<div key={item.id}>
<h2>{item.title}</h2>
<ul>
{item.details.map(detail => (
<li key={detail.id}>{detail.description}</li>
))}
</ul>
</div>
))}
</div>
);
};
export default App;
Output:
Nested JSON Data
Title 1
- Detail A
- Detail B
Title 2
- Detail C
- Detail D
In this example, we fetch nested JSON data from an API. Each item has a title and an array of details. We first map over the main data to render each title, and then we map over the details array to display the corresponding descriptions. This method demonstrates how to effectively navigate and display nested JSON structures in React, enhancing the way data is presented to users.
Conclusion
Displaying JSON data in React is a fundamental skill that can significantly enhance your application’s interactivity and user experience. Whether fetching data from an API, using local JSON files, or handling nested structures, React provides flexible methods to manage and render this data effectively. By mastering these techniques, you can create dynamic applications that provide users with up-to-date information in an engaging manner.
FAQ
-
What is JSON?
JSON stands for JavaScript Object Notation, a lightweight format for data interchange that is easy to read and write. -
How do I fetch JSON data in React?
You can use thefetch
function within theuseEffect
hook to retrieve JSON data from an API. -
Can I display local JSON files in React?
Yes, you can import local JSON files and use them in your components, similar to how you would fetch data from an API. -
How do I handle nested JSON data in React?
You can map over the main data and then further map over nested arrays to display the information correctly. -
What are the benefits of using JSON in React?
JSON is easy to work with, lightweight, and integrates seamlessly with JavaScript, making it ideal for dynamic web applications.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn