How to Import Styles in React
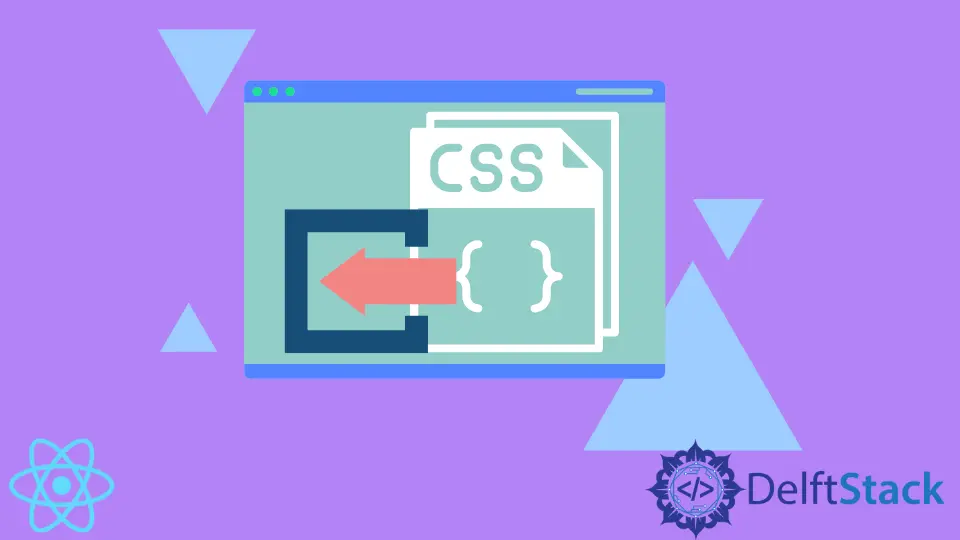
We will introduce how to import styles and write inline CSS in our React application.
Styling is precious to create a strong client experience or end-users in your websites or web applications.
Keeping colors, fonts, buttons, heading sizes, and image styles, sizes, and backgrounds modern and consistent makes the user experience and interface better.
These are all the elements that make a website or web application visually appealing.
We will go through 2 examples of setting styles for our applications by importing a stylesheet or using inline CSS in React.
Import a Stylesheet to Set Styles in React
First, we will create a new application and style it by importing a stylesheet. Let’s create a new application using the command below.
# react
npx create-react-app my-app
After creating our new application in React, we will go to our application directory using this command.
# react
cd my-app
Now, let’s run our app to check if all dependencies are installed correctly.
# react
npm start
In App.js
, we will import a stylesheet.
# react
import "./styles.css";
In return, we will create a template.
# react
<div className="App">
<h1>Welcome Back</h1>
<p>Start editing to see some magic happen!</p>
<button>Save</button>
</div>
Now we will add CSS to style our template.
# react
.App {
font-family: sans-serif;
text-align: center;
}
button{
padding: 5px 10px;
color: white;
background: black;
border: none;
}
Output:
We can easily import stylesheets and style our components by following these simple steps.
Check the code here.
Use the Inline CSS to Set Styles in React
Let’s talk about how to style our components using inline CSS. We will go through an example to understand how to use inline CSS in React.
So, let’s create a new application by using the following command.
# react
npx create-react-app my-app
After creating our new application in React, we will go to our application directory using this command.
# react
cd my-app
Now, let’s run our app to check if all dependencies are installed correctly.
# react
npm start
We need to install a few libraries to help us implement hover effects in our application.
We will run the following command to install react-hook
for hover.
# react
npm i @react-hook/hover
We will run the following command to install dash-ui/styles
.
# react
npm i @dash-ui/styles
Once we have installed our libraries, we need to import useHover
and styles
in the App.js
file.
# react
import useHover from "@react-hook/hover";
import { styles } from "@dash-ui/styles";
Now, we will define our Element
and Hovered
elements inside export default function App()
.
And we will return a div
which will change its class name once it was hovered and had a ref
of Element
, and it will output hovered style if someone hovers onto it or default style if no one is hovering on it.
# react
export default function App() {
const Element = React.useRef(null);
const Hovered = useHover(Element);
return (
<div>
<h1 className={divStyle({ Hovered })} ref={Element}>Styling With Inline CSS</h1>
<button className={hoverStyle({ Hovered })} ref={Element}>Button Style</button>
</div>
);
}
Now, we will define our CSS styles for Hovered
and default
classes. So our code in App.js
will look like below.
# react
import React from "react";
import useHover from "@react-hook/hover";
import { styles } from "@dash-ui/styles";
export default function App() {
const Element = React.useRef(null);
const Hovered = useHover(Element);
return (
<div>
<h1 className={divStyle({ Hovered })} ref={Element}>Styling With Inline CSS</h1>
<button className={hoverStyle({ Hovered })} ref={Element}>Button Style</button>
</div>
);
}
const hoverStyle = styles({
default: `
background: black;
padding: 10px;
color: white;
border: none;
`,
Hovered: `
color: black;
background: white;
border: 1px solid black;
`
});
const divStyle = styles({
default: `
color: black;
`,
Hovered: `
color: blue;
`
});
Output:
Check the code here.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn