The if Statement in React
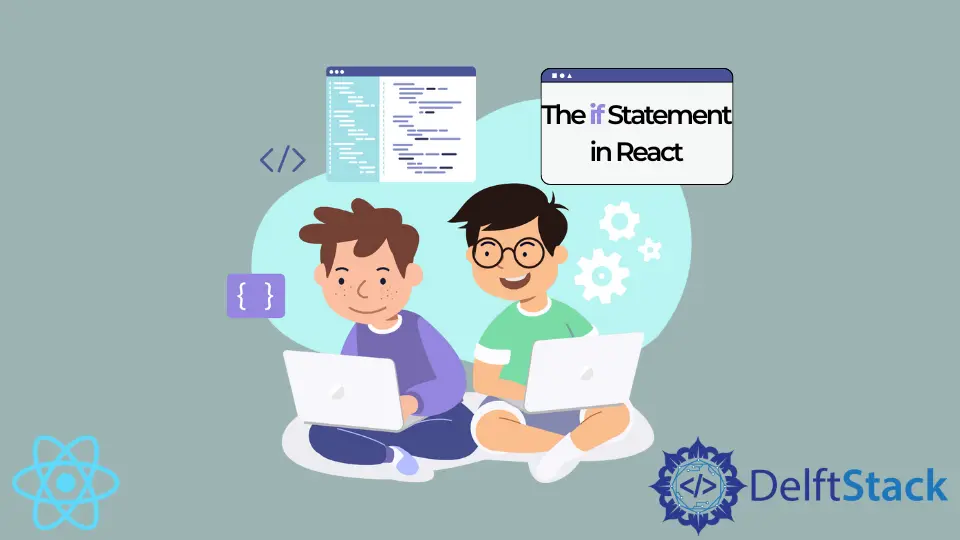
We will introduce the if
statement in react and how to use it in the react render
functions.
if Statement in React
When we build a react application, we may often need to display or hide some content based on a certain condition. Conditional rendering in react works the same way as the conditions work in JavaScript.
First, we will create a new file called UserGreetings.Js
; within the file, let’s create a class component. Now let’s remove the named export
.
In JSX
, we will return Welcome User!
. Our UserGreetings.Js
file will look like below.
# react
import React, {Component} from 'react';
class UserGreetings extends Component {
render() {
return (
<div>
Welcome User!
</div>
);
}
}
export default UserGreetings;
Now we will import UserGreetings
in the App.js
file.
# react
import UserGreetings from "./UserGreetings"
Now we will include the UserGreetings
component.
# react
<UserGreetings />
So, our code in App.js
will look like below.
# react
import "./styles.css";
import UserGreetings from "./UserGreetings"
export default function App() {
return (
<div className="App">
<UserGreetings />
</div>
);
}
Output:
Now, let’s go back to the UserGreetings.js
file and make changes by adding a constructor and within the constructor call super
and then defining the state
. Now let’s create one state property called LoggedIn
and initialize it to false
.
Now in the JSX
, let’s add another message that says Welcome Guest!
. Our code in UserGreetings.js
will look like below.
# react
import React, { Component } from "react";
class UserGreetings extends Component {
constructor(props) {
super(props);
this.state = {
LoggedIn: false
};
}
render() {
return (
<div>
<div>Welcome User!</div>
<div>Welcome Guest!</div>
</div>
);
}
}
export default UserGreetings;
Now, let’s create a if
statement. If LoggedIn
is true
, it should display Welcome User!
and if LoggedIn
is false
, it should display Welcome Guest!
.
# react
if(this.state.LoggedIn){
return(
<div>Welcome User!</div>
)
}
and else
condition if LoggedIn
is false.
# react
else{
return(
<div>Welcome Guest!</div>
)
}
So, our UserGreetings.js
file will look like below.
# react
import React, { Component } from "react";
class UserGreetings extends Component {
constructor(props) {
super(props);
this.state = {
LoggedIn: false
};
}
render() {
if(this.state.LoggedIn){
return(
<div>Welcome User!</div>
)
}
else{
return(
<div>Welcome Guest!</div>
)
}
}
}
export default UserGreetings;
Output:
So, As you can see in the result, it works fine. It has returned Welcome Guest
because LoggedIn
is set to false
.
Now let’s set it to true
.
# react
import React, { Component } from "react";
class UserGreetings extends Component {
constructor(props) {
super(props);
this.state = {
LoggedIn: true
};
}
render() {
if(this.state.LoggedIn){
return(
<div>Welcome User!</div>
)
}
else{
return(
<div>Welcome Guest!</div>
)
}
}
}
export default UserGreetings;
Output:
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn