extends in React JS
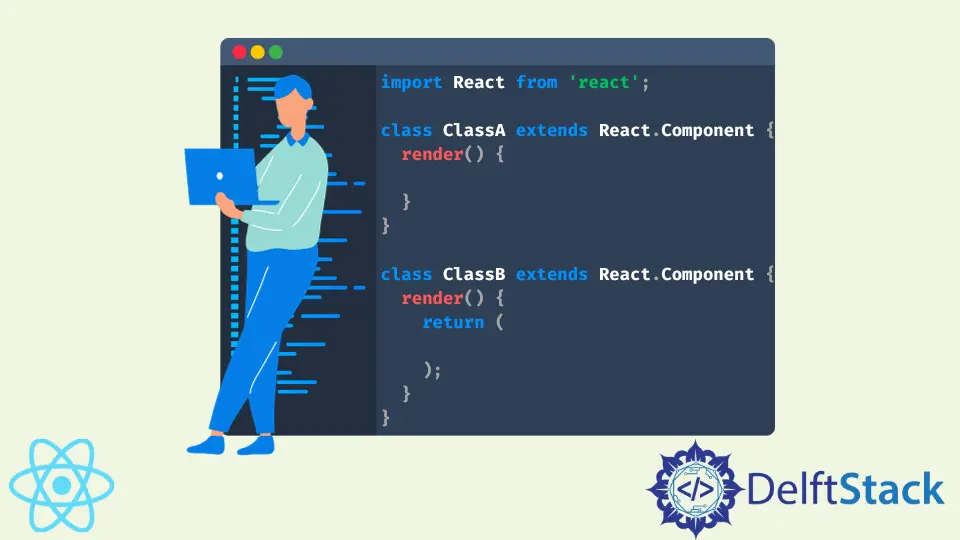
Inheritance is an essential concept for object oriented architecture. In React JS, we mainly use inheritance to get access to React.Component
to build the UI.
For example, we mostly use class ClassA extends React.Component
, meaning that the ClassA
has access to the elements of React.Component
.
This article will discuss the extends
keyword in React JS. We will also look at an example with an explanation to make the topic easier.
Use the extends
Keyword in React JS
In our example below, we will discuss a simple example regarding inheritance in React JS. First, take a look at the below example code.
import React from 'react';
class ClassA extends React.Component {
render() {
return <h2>I am from ClassA<
/h2>;
}
}
class ClassB extends React.Component {
render() {
return (
<div>
<h1>I am from ClassB</h1>
<ClassA />< /div>
);
}
}
export default ClassB;
In the example above, the keyword extends
is used to access the components of React.Component
.
For the class-to-class reference, we use the general syntax below:
<ClassName />
This will access the default export from the class. If you have more elements inside the class, you can import them like the following:
import {element1, element2, ...} from './YourClass';
And then you can easily access them as shown below:
<element1 />
Now, after you are done with all the files, you will get the output below in your browser,
Let’s see another example regarding the inheritance of React components. Here we illustrate how we can extend components directly from another class.
import React from 'react';
class Class_Parent extends React.Component {
constructor(props) {
super(props);
this.Parent_Class_Function = this.Parent_Class_Function.bind(this);
}
// Function of Parent Class.
Parent_Class_Function() {
console.log('This is a parent class method.');
}
render() {
return false;
}
}
export default class Class_Child extends Class_Parent {
constructor() {
super();
}
render() {
this.Parent_Class_Function();
return false;
}
}
The above example demonstrated how to extend the components from another class in React JS.
In the function above, we created a class named Class_Parent
with a function Parent_Class_Function()
. After that, we generated another class that extends the Class_Parent
through the following line:
export default class Class_Child extends Class_Parent
Now we can easily access the parent function Parent_Class_Function()
.
Now, when you run the above example, you will get the below output in your web console:
This is a parent class method.
This tutorial uses the react-select
package, so you need to install this package before running the application. You can easily install this using npm
.
The example codes shared in this article are written in React JS project. To run a React project, you must have the latest Node JS version installed on your system.
If your system doesn’t contain Node JS, install it first.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn