How to Dispatch in React
- What is Dispatch in React
-
Use the
dispatch
Function in React -
Increment and Decrement Using the
dispatch()
Function in React
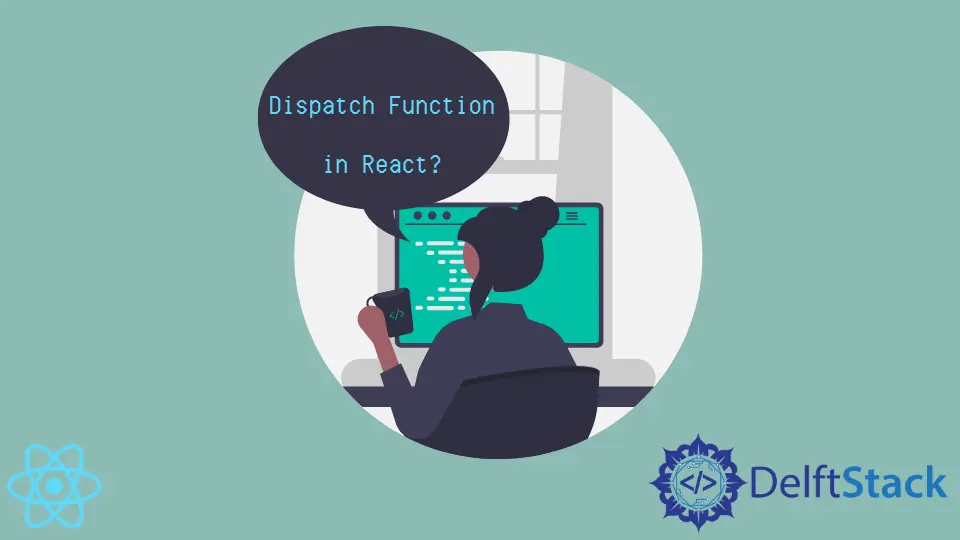
We will introduce dispatch in React and create a counter application using useReducer
and dispatch
to understand dispatch in detail in the example.
What is Dispatch in React
We encounter a dispatch function when learning or using redux or useReducer in our React application. We will understand what dispatch is and how to use it in useReducer
to build a counter application in React.
The dispatch
function relies on functional programming because it requires switching our mental model of managing data in our application and is difficult and complex to understand.
The dispatch()
is used to dispatch actions and trigger state changes to the store. Managing the state in React is one of the main issues we face while developing websites in React.
The useReducer
is used to achieve it. We will understand useReducer
first and then discuss dispatch.
We are quite familiar with useState
, but useReducer
is also the native way of managing the state in React. The useReducer
uses dispatch
instead of setState
.
# react
const [state, setState] = useState(counter);
const [state, dispatch] = useReducer(reducer, counter);
We will go through an example to understand how to use dispatch in our React application. Let’s create a new application by using the following command.
# react
npx create-react-app my-app
After creating our new application in React, we will go to our application directory using this command.
# react
cd my-app
Let’s run our app to check if all dependencies are installed correctly.
# react
npm start
We will import the useReducer
hook in App.js
.
# react
import React, { useReducer } from "react";
Once we have imported useReducer
, we will go into our default function. We will return it in an array, and the useReducer
function will take two different parameters to start.
We will have a reducer, a function that we perform on our state to get a new state, and we will define it before our default function, and it will also have an initial value.
We will use an object as an initial value. We will set a count
equal to 0. This process is the same as setting our state using useState
.
So, our code in App.js
will look like below.
# react
import "./styles.css";
import React, { useReducer } from "react";
function redcuer(){
}
export default function App() {
const [] = useReducer(redcuer, { count: 0 })
return (
<div className="App">
</div>
);
}
The above code shows that we could pass in 0 instead of an object for the initial value in useReducer
. Generally, when we work with reducer and useReducer
, we will use objects instead of actual values because, generally, our state is more complex than a single value.
That’s why we used an object. The return value will be in two parts; the first part will be the state.
So, we will use state, but if we were using a single value as an initial value for useReducer
, we could have gone with the count
. Now, the second part will be a function dispatch
.
Use the dispatch
Function in React
Dispatch is what we call to update our state, and it will call the reducer for our given certain parameters. On the other hand, the reducer will take 2 things as well.
First, it will take the current state, which means the current state of our application. And reducer will also take action.
So whenever we call dispatch, it will take whatever we call dispatch with, and it will send it to the action variable, and our current state will be in a state variable. And the reducer will return our new updated state.
Our code will look like below.
# react
import "./styles.css";
import React, { useReducer } from "react";
function redcuer(state, action){
}
export default function App() {
const [state, dispatch] = useReducer(redcuer, { count: 0 })
return (
<div className="App">
</div>
);
}
Now, let’s test our application by creating a frontend. So, we will create 2 buttons, 1 for increment and the other for decrement.
And in the middle of these buttons, we will display the current state of the count
. These buttons will call to functions increment
and decrement
whenever a user clicks on them, respectively.
In our reducer function, we will increment the count value with 1 and return it. And in the increment
function, we will only call dispatch()
to update the state for the count.
So, our code in App.js
will look like below.
# react
import "./styles.css";
import React, { useReducer } from "react";
function redcuer(state, action){
return { count: state.count + 1 }
}
export default function App() {
const [state, dispatch] = useReducer(redcuer, { count: 0 })
function decrement(){
}
function increment(){
dispatch()
}
return (
<div className="App">
<button onClick={decrement}>-</button>
<span>{state.count}</span>
<button onClick={increment}>+</button>
</div>
);
}
Output:
The above example shows that we easily update the count state using a dispatch()
function. Now we will understand how to have different types of dispatch()
and separate them from one other.
Increment and Decrement Using the dispatch()
Function in React
So, now let’s imagine if we want to use both increment and decrement using dispatch()
, how will we use it? We can define types of dispatch
that can help our reducer function understand what kind of dispatch it is and what our function has to do if a certain dispatch type is activated.
So, in our increment
function, instead of just passing a function dispatch
, we will also define the type of dispatch, that is, increment. Our function will look like below.
# react
function increment(){
dispatch({ type: 'increment' })
}
Once we have passed the dispatch type, we can now use switch
statements in our reducer function to check the type of action and perform the action accordingly. We will have three switch cases in the reducer function, one will be increment, and the other will be the decrement.
And the last one will be the default. It is good to have a default switch; we can either throw an exception or execute a line of code.
We will return the state in the default case. Our reducer function will look like below.
# react
function redcuer(state, action){
switch(action.type){
case 'increment':
return { count: state.count + 1 }
case 'decrement':
return { count: state.count - 1 }
default:
return state;
}
}
We will call the dispatch function in the decrement
function with the decrement type.
# react
function decrement(){
dispatch({ type: 'decrement' })
}
Let’s run our application and check how it works.
Output:
The above example shows that we can easily use dispatch to dispatch actions with certain types and use useReducer
and reducer.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn