Props and Children in React
-
What Is
props.children
in React -
Passing
props.children
in React JSX -
Use
children
Attribute to Pass Down Children in React -
Use
React.createElement()
to Pass Down Children in React
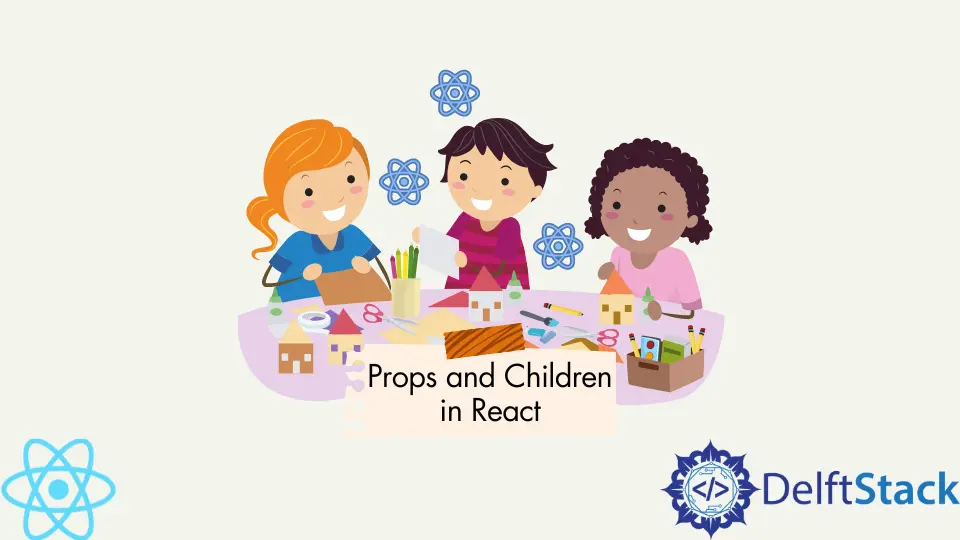
This article will explore what props.children
is and why it is useful for customizing components.
What Is props.children
in React
Like any other component-based library, React facilitates code reusability. First and foremost, this is achieved by re-using the components, but there’s more.
Since the framework follows the declarative approach, it’s easy to include components and nest them.
React features the tools and framework you need to build generic components and customize them using the composition model.
To customize your components using the composition model, you’ll have to access the children
property of your props
object. By default, every component has this property.
To put it in simple words, props.children
of children components can be used to access the nested value between the opening and closing tags when this child component is invoked. Let’s look at a simple example.
export default function App() {
return (
<div className="App">
<Child>
<h1>Hello, world</h1>
</Child>
</div>
);
}
function Child(props) {
return <div>{props.children}</div>;
}
In this example, it might seem like we are displaying content directly in the App
component, but we’re passing the content between the opening and closing tags of the <Child>
component as props
. Later we reference the <h1>
element in our Child
component.
You can check out the live code sample on CodeSandbox.
Please note that you must invoke a component with opening and closing tags for the props.children
feature to work.
In some cases, React developers write self-closing tags: <Child />
. This is incompatible with props.children
, which needs opening and closing tags to work.
Passing props.children
in React JSX
This feature of React is particularly useful when you need to dynamically generate content and pass it into the child component, which could be just a styled container. A good example of this use case is the message box in any messenger app.
We passed just one <h1>
element from the parent to the child component through props
in the example. You aren’t limited in the volume of content you want to pass down to a child component.
Anything you put between the child component’s opening and closing JSX tags can be rendered in the child component through props.children
.
If you have a lot of content to pass down as children
, you may need to pass them into multiple holes in the child component. React gives developers the freedom to create custom prop attributes on the child component.
Let’s look at an example.
export default function App() {
return (
<div className="App">
<Child
greeting={<h1>Hello, world</h1>}
goodbye={<h1>Goodbye, world</h1>}
>
</Child>
</div>
);
}
function Child(props) {
return <div>
<div className="top">{props.greeting}</div>
<div className="bottom">{props.goodbye}</div>
</div>;
}
In this case, instead of passing the <h1>
element between the opening and closing tags, we create custom attributes greeting
and goodbye
and pass the elements as props.
In the Child
component, we have two separate <div>
elements that display values from each attribute.
The key takeaway from this example is that you can pass down HTML elements like <h1>
as props, as well as custom components. For instance, Vue has a similar concept called slots
.
However, in React, you aren’t limited to what value you can pass down as props.
Use children
Attribute to Pass Down Children in React
The most common way to pass down children
values in React is to put them between the opening and closing tags of the component. Alternatively, you can use a children
value on the component and set its value.
Use React.createElement()
to Pass Down Children in React
JSX allows us to define and invoke React components declaratively, but all JSX code is ultimately compiled to React.createElement()
calls.
If you don’t use JSX to develop your web app, you can directly use the React.createElement()
method. The first two arguments are type
and props
.
After that, any subsequent argument will be passed down to the created element as children and can be referenced by accessing props.children
value.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn