How to Export Default in React
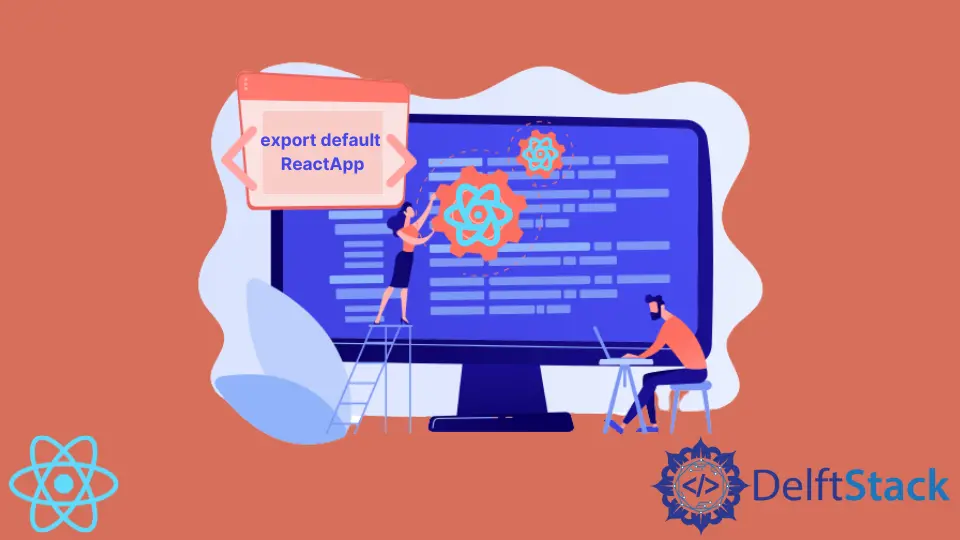
We will introduce types of export
in React and what they do.
Types of Exports in React
Export like export default ReactApp;
and import like import logo from './logo.svg'
is part of the ES6 Modules syste.
There are two kinds of exports in ES6
: named exports and default exports.
Named Exports in React
The exports which are exported using the name of the function are known as named exports such as export Function ExFunc(){}
.
Named modules can be imported using import { ExFunc } from 'module';
. The import name should be the same as the name of the export, like in this example ExFunc
.
We can have multiple named exports
in one module.
Default Exports in React
Default export
is used to export a single class, primitive, or function from a module. There are different ways to use default export
.
Usually, the default export
is written after the function, like the example below.
# react
function App() {
return (
<div className="App">
<header className="App-header">
<img src={logo} className="App-logo" alt="logo" />
</header>
</div>
);
}
export default App;
But it can also be written like below.
# react
export default class ReactApp extends React.Component {
render() {
return <p>Exported Using Default Export</p>;
}
}
And for functions, it can be written as.
# react
export default function ReactApp() {
return <p>Exported Using Default Export</p>
}
Once we have used a default export
, we don’t necessarily need to import it as ReactApp
; we can give it any name.
# react
import Y from './ReactApp';
Y
is the name that will be given locally to the variable assigned to contain the value, and it doesn’t have to be named the origin export.
One important thing to remember while using default export
is that there can only be one default export
, unlike named export
. A module can have both named export
and a default export
, and they can be imported together.
# react
import Y, { ExFunc1, ExFunc2, etc... } from 'module';
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn