How to Increase the Memory for R Processes
- Understanding Memory Management in R
- Increase Memory Manually in R
-
Increase Memory Using the
memory.limit()
Method in R -
Use
gc()
for Garbage Collection in R - Use Command-Line Options to Increase the Memory for R Processes
- Adjust System Environment Variables
- Conclusion
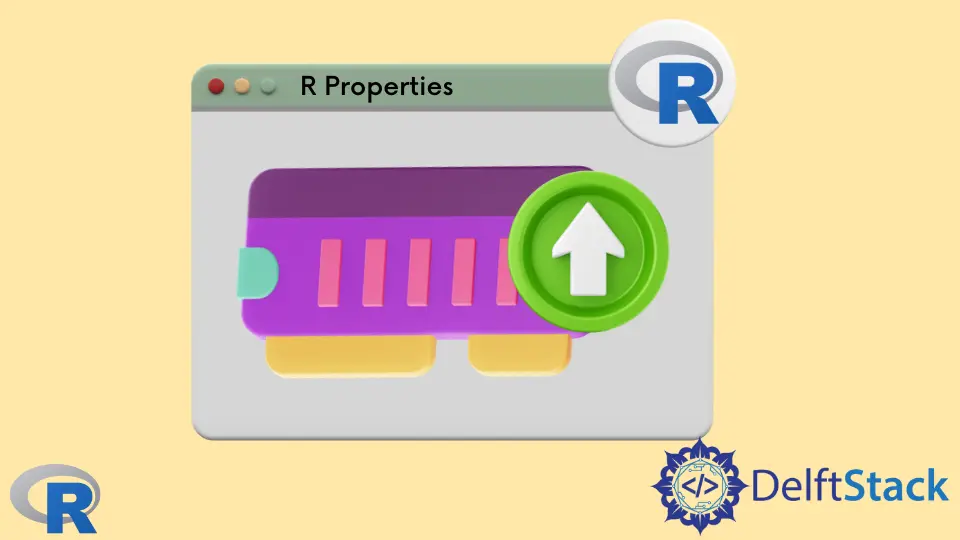
Memory constraints often pose challenges when working with data-intensive tasks in R, and sometimes, R users get the out-of-memory error. However, there are several methods to increase the memory available to R processes, ensuring smoother execution of memory-demanding operations.
Let’s explore various approaches along with code examples to illustrate each method’s implementation. This tutorial demonstrates how to increase memory in R.
There are two methods to increase the memory in R; one is manual, and the other is by using a method.
Understanding Memory Management in R
R is a powerful programming language widely used for statistical computing and graphics. However, when dealing with large datasets or complex computations, you might encounter memory limitations that can hinder the execution of R scripts or cause them to crash.
Increasing memory for R processes is a crucial step to handle such situations effectively.
Before delving into increasing memory, it’s essential to comprehend how R manages memory. R operates within a memory limit that you can modify to suit your needs.
R has two primary memory limits:
- Physical Memory Limit: This is the actual RAM available on your machine.
- Virtual Memory Limit: This limit is set within R and determines how much RAM R can use from the available physical memory.
Increase Memory Manually in R
Windows users mostly get out-of-memory errors. We can increase the memory of R from the properties of the R program or app.
Here’s how you can manually increase memory allocation for R processes on a Windows operating system:
-
Right-click on the R program icon from the desktop or the program directory and go to
Properties
. -
Go to the
Shortcut
tab and find theTarget
field. -
Add the following line into the
Target
field.--max-mem-size=1000M
-
The code above will allocate 1000 MB of memory to the R program; the maximum can be the memory up to the memory of R.
-
Finally, click
OK
, and you are good to go. -
There might be an error that R cannot allocate the vector of length. Then add the following line to the
Target
field and clickOK
.--max-vsize=1000M
Increase Memory Using the memory.limit()
Method in R
The memory.limit()
function in R allows users to query and set the maximum amount of memory available to R processes.
By default, R allocates a certain amount of memory, which might not be sufficient for memory-intensive operations. Increasing this limit can prevent crashes and allow larger datasets or more complex analyses to run smoothly.
However, it’s important to note that this function is specific to Windows and might not be available or might behave differently on other operating systems.
Syntax of memory.limit()
Querying Current Memory Limit:
memory.limit()
This command, with no arguments, returns the current memory limit in megabytes (MB) that R is allowed to use on the system. It simply displays the current memory allocation.
Setting New Memory Limit:
memory.limit(size)
The memory.limit()
function, when used with an argument size
, sets the maximum amount of memory that R can use to the specified size
in megabytes. Replace size
with the desired amount of memory to be allocated in MB.
Example:
memory.limit(size = 1000)
# or
memory.limit(1000)
The code above will allocate the 1000 MB of memory to the R program.
Using memory.limit()
With Administrative Privileges
In Windows environments, administrative privileges might be required to increase the memory limit beyond the default maximum.
# Setting R Memory Limit with Administrative Privileges
if (Sys.info()["sysname"] == "Windows") {
# Run R as administrator and execute the following command:
system("powershell -Command 'Start-Process Rgui -Verb runAs'")
memory.limit(size = 8000) # Set memory limit to 8000 MB (8 GB)
}
This code checks the operating system, and if it’s Windows, it suggests running R as an administrator to set the memory limit beyond the default maximum.
Using memory.limit()
in RStudio
RStudio is an integrated development environment (IDE) specifically designed for R programming. It provides a user-friendly interface with various tools and features tailored to support R users in writing, debugging, and executing R code efficiently.
In RStudio, memory limits can be adjusted using the memory.limit()
function within the R console.
# Adjusting R Memory Limit in RStudio
memory.limit(size = 8000) # Set memory limit to 8000 MB (8 GB)
Executing this code within the RStudio console increases the memory limit for R processes.
Use gc()
for Garbage Collection in R
Garbage collection in R is a process that automatically identifies and removes unreferenced or unused objects from the memory, freeing up space for new data or computations.
As an interpreted language, R manages memory dynamically, creating objects and allocating memory as needed during program execution. However, this dynamic memory management can lead to memory fragmentation and inefficiencies.
The process of garbage collection in R involves identifying objects that are no longer referenced or accessible by the program. When an object is created in R, it occupies memory space.
If no references are pointing to that object (i.e., no variables or data structures using that object), it becomes eligible for garbage collection.
R’s garbage collector periodically scans the memory to find and remove these unreferenced objects, reclaiming the memory they were occupying. The garbage collection process helps prevent memory leaks and keeps memory usage efficient by freeing up space that can be utilized for new objects or computations.
Users can also manually trigger garbage collection in R using the gc()
function. Invoking gc()
prompts an immediate garbage collection cycle, attempting to free up memory by removing unused objects.
However, it’s important to note that calling gc()
explicitly is typically not necessary, as R’s garbage collector automatically manages memory.
Here’s an example of using the gc()
function in R:
# Generate some data and perform operations
data <- runif(10^7) # Create a large dataset
result <- sum(data) # Perform some computation
# Perform garbage collection explicitly
gc() # Trigger garbage collection
Routinely performing garbage collection using the gc()
function can help free up memory space by removing unused objects. The gc()
function triggers the garbage collection process in R, releasing memory occupied by unreferenced objects.
While garbage collection helps manage memory usage, excessive or frequent use of gc()
can impact performance as it momentarily pauses program execution to clean up memory.
R’s automatic garbage collection is generally efficient, so manual intervention is seldom required unless working with exceptionally large datasets or encountering specific memory-related issues.
Use Command-Line Options to Increase the Memory for R Processes
Command-line options are parameters passed to the R executable when launching R from the terminal or command prompt. These options modify the behavior of the R process, including memory allocation.
Methods to Increase Memory Using Command-Line Options
When launching R, command-line options can be utilized to specify memory limits:
-
--max-mem-size
Option: This option sets the maximum amount of memory R can utilize in bytes.R --max-mem-size=8000000000
This command allocates approximately 8 gigabytes of memory (8,000,000,000 bytes) to the R process.
-
--max-vsize
Option: It specifies the maximum address space size that R can use.R --max-vsize=8000000000
This command sets the maximum virtual memory size to approximately 8 gigabytes for the R process.
-
Combining Options: Both
--max-mem-size
and--max-vsize
options can be used together to enhance memory allocation.
R --max-mem-size=8000000000 --max-vsize=8000000000
This command allocates both physical and virtual memory to the specified limit.
-
Using Environment Variables: Environment variables like
R_MAX_MEM_SIZE
andR_MAX_VSIZE
can also be utilized to set memory limits.export R_MAX_MEM_SIZE=8000000000 export R_MAX_VSIZE=8000000000 R
Setting these environment variables before launching R ensures the R process operates within the specified memory limits.
-
Windows-Specific Options: On Windows, different command-line options might be used to set memory limits.
R --max-mem-size=8000000000
Windows users can use similar options to increase memory for R processes but with a slight variation in syntax compared to Unix-based systems.
Adjust System Environment Variables
System environment variables are settings that define the behavior of various processes and applications running on an operating system. These variables are accessible globally and can influence the behavior and resource allocation of programs like R.
Methods to Adjust System Environment Variables
-
R_MAX_MEM_SIZE
Variable:R_MAX_MEM_SIZE
defines the maximum amount of memory (in bytes) R can utilize.export R_MAX_MEM_SIZE=8000000000
Setting
R_MAX_MEM_SIZE
to approximately 8 gigabytes allocates this amount of memory for R processes. -
R_MAX_VSIZE
Variable:R_MAX_VSIZE
determines the maximum address space size that R can use.export R_MAX_VSIZE=8000000000
Adjusting
R_MAX_VSIZE
to 8 gigabytes sets the maximum virtual memory size for R processes. -
Setting Variables in Startup Scripts: Edit system startup scripts (e.g.,
.bashrc
,.bash_profile
) to automatically set these variables on system startup.echo 'export R_MAX_MEM_SIZE=8000000000' >> ~/.bashrc echo 'export R_MAX_VSIZE=8000000000' >> ~/.bashrc source ~/.bashrc
Appending these commands to startup scripts ensures that the variables are set every time the system boots up.
-
Windows Environment Variable Configuration: Adjust environment variables via the Control Panel on Windows systems.
- Navigate to
Control Panel
>System and Security
>System
>Advanced system settings
. - Click on
"Environment Variables"
and add new variables (R_MAX_MEM_SIZE
,R_MAX_VSIZE
) with appropriate values.
- Navigate to
-
Effect on R Processes: After configuring these variables, launching R processes will allow them to utilize the allocated memory limits.
-
Combining Linux command with R Execution: The
ulimit
command in Linux allows users to set various resource limits for processes, including memory limits. Whileulimit
primarily focuses on the user-level resource limits, it can indirectly impact the memory available to R processes.Launching R processes while applying the memory limit set by
ulimit
.ulimit -v 8000000 && R
This command combines setting the memory limit using
ulimit
with launching R, ensuring that the R process adheres to the specified memory constraints.
Conclusion
In handling memory constraints within R, it’s vital to optimize memory usage, especially when working with extensive datasets and memory-demanding operations. This guide detailed multiple methods to increase memory for R processes, spanning manual adjustments, command-line options, system environment variables, and Linux-specific ulimit
commands.
These approaches enable users to efficiently allocate memory and prevent out-of-memory errors, enhancing the execution of complex analyses and computational tasks in the R programming language.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook