How to Export Data Frame to CSV in R
- Using the write.csv() Function
- Using the write.table() Function
- Using the readr Package
- Conclusion
- FAQ
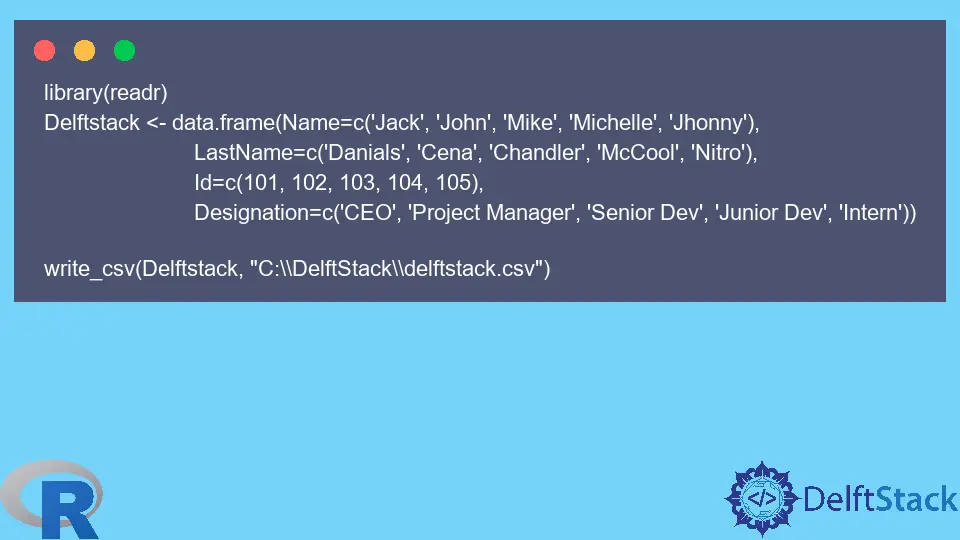
Exporting data frames to CSV in R is a fundamental skill for anyone working with data analysis. Whether you’re a data scientist, statistician, or just someone who enjoys playing with data, knowing how to effectively export your data sets is crucial.
In this tutorial, we will walk you through the steps to export a data frame to a CSV file using R. We will cover various methods, ensuring you have the right tools and knowledge to handle your data efficiently. By the end of this article, you will be equipped to save your data frames in a widely-used format, making it easy to share and analyze your data further. Let’s dive in!
Using the write.csv() Function
One of the simplest ways to export a data frame to CSV in R is by using the write.csv()
function. This built-in function allows you to specify the data frame you want to export, the file path where you want to save it, and several other options to customize your output.
Here’s how it works:
# Create a sample data frame
data <- data.frame(Name = c("Alice", "Bob", "Charlie"),
Age = c(25, 30, 35),
City = c("New York", "Los Angeles", "Chicago"))
# Export the data frame to a CSV file
write.csv(data, file = "output.csv", row.names = FALSE)
Output:
Name,Age,City
Alice,25,New York
Bob,30,Los Angeles
Charlie,35,Chicago
In this code, we first create a sample data frame named data
with three columns: Name, Age, and City. The write.csv()
function is then called to save this data frame to a file named output.csv
. The argument row.names = FALSE
is included to prevent R from writing row numbers into the CSV file, which is often unnecessary for data analysis. This method is straightforward and effective for most use cases.
Using the write.table() Function
Another versatile function to export data frames in R is write.table()
. This function provides more options for customization, allowing you to define delimiters and other formatting options.
Here’s an example:
# Create a sample data frame
data <- data.frame(Name = c("Alice", "Bob", "Charlie"),
Age = c(25, 30, 35),
City = c("New York", "Los Angeles", "Chicago"))
# Export the data frame to a CSV file using write.table
write.table(data, file = "output_table.csv", sep = ",", row.names = FALSE, col.names = TRUE)
Output:
Name,Age,City
Alice,25,New York
Bob,30,Los Angeles
Charlie,35,Chicago
In this example, we again create a sample data frame and use write.table()
to save it as output_table.csv
. The sep
argument specifies that we want to use a comma as the delimiter, which is standard for CSV files. We also set row.names = FALSE
to exclude row names and col.names = TRUE
to ensure that column headers are included in the output. This method is particularly useful if you need to export data in formats other than CSV by simply changing the delimiter.
Using the readr Package
For those who prefer a tidyverse approach, the readr
package offers a function called write_csv()
that is optimized for performance and ease of use. This package is part of the tidyverse collection, which is widely used in the R community for data manipulation and visualization.
Here’s how to use it:
# Load the readr package
library(readr)
# Create a sample data frame
data <- data.frame(Name = c("Alice", "Bob", "Charlie"),
Age = c(25, 30, 35),
City = c("New York", "Los Angeles", "Chicago"))
# Export the data frame to a CSV file using write_csv
write_csv(data, "output_readr.csv")
Output:
Name,Age,City
Alice,25,New York
Bob,30,Los Angeles
Charlie,35,Chicago
In this example, we first load the readr
package. The write_csv()
function is then used to export our data frame to a file named output_readr.csv
. One of the advantages of using write_csv()
is that it automatically handles the row names and column headers for you, making it a cleaner option for those who prefer simplicity. Additionally, the readr
package is optimized for speed, making it suitable for larger datasets.
Conclusion
Exporting a data frame to CSV in R is a straightforward process that can be accomplished using various methods. Whether you choose the built-in write.csv()
and write.table()
functions or opt for the tidyverse’s write_csv()
, each method has its strengths. Knowing how to effectively export your data sets allows you to share your findings with others or conduct further analysis using different tools. With these techniques at your disposal, you can confidently manage your data export tasks in R.
FAQ
-
How do I specify a different file path when exporting a CSV in R?
You can specify a different file path by providing the full path in thefile
argument of the write functions, such aswrite.csv(data, file = "C:/path/to/your/directory/output.csv")
. -
Can I export a data frame with row names in R?
Yes, you can include row names by settingrow.names = TRUE
in thewrite.csv()
orwrite.table()
functions. -
What is the difference between write.csv() and write.table()?
write.csv()
is a specialized version ofwrite.table()
with default settings for comma-separated values, whilewrite.table()
allows for more flexibility with delimiters and formatting. -
Is it necessary to install additional packages to use write_csv()?
Yes, you need to install thereadr
package from the tidyverse to use thewrite_csv()
function. -
How can I check if my CSV file was created successfully?
You can check the directory where you saved the file and open it using a text editor or spreadsheet software to verify its contents.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook