How to Find Index of an Element in a R Vector
-
Using the
which()
Function -
Using the
match()
Function -
Using the
which()
Function with a Condition - Using the for Loop
- Conclusion
- FAQ
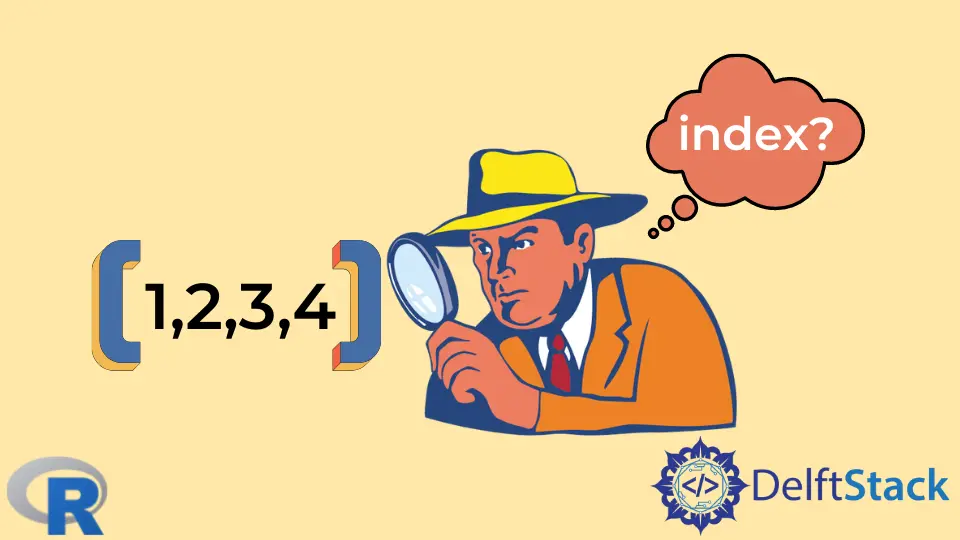
Finding the index of an element within a vector in R is a fundamental task that can come in handy for data analysis and manipulation. Whether you’re working with datasets, performing statistical analyses, or simply exploring data, knowing how to efficiently locate elements in a vector is crucial.
In this tutorial, we will explore various methods to find the index of an element in an R vector, complete with clear examples and explanations. By the end of this article, you will have a solid understanding of how to retrieve indices in R, making your data handling smoother and more effective. Let’s dive in!
Using the which()
Function
One of the most straightforward methods to find the index of an element in an R vector is by using the which()
function. This function returns the indices of the logical object that are TRUE. It’s particularly useful when you want to locate specific values within your vector.
Here’s how you can use the which()
function:
my_vector <- c(10, 20, 30, 40, 50)
element_to_find <- 30
index <- which(my_vector == element_to_find)
index
Output:
[1] 3
In this example, we create a vector named my_vector
containing five numeric elements. We then specify the element we want to find, which is 30
. By using the which()
function, we can easily find out that the index of 30
in my_vector
is 3
. This method is efficient and concise, making it a popular choice among R users.
Using the match()
Function
Another effective way to find the index of an element in an R vector is by utilizing the match()
function. This function returns the position of the first match of its first argument in its second argument, which can be quite handy for vector comparisons.
Here’s how to use match()
:
my_vector <- c("apple", "banana", "cherry", "date")
element_to_find <- "banana"
index <- match(element_to_find, my_vector)
index
Output:
[1] 2
In this scenario, we have a character vector my_vector
with fruit names. We want to find the index of "banana"
. By employing the match()
function, we discover that "banana"
is located at index 2
in the vector. This method is particularly useful when working with character data or when you want to find the position of a specific item in a list.
Using the which()
Function with a Condition
Sometimes, you may want to find the index of an element based on a condition rather than an exact match. The which()
function can also be used in combination with logical conditions to achieve this.
Here’s an example:
my_vector <- c(5, 15, 25, 35, 45)
condition <- my_vector > 20
indices <- which(condition)
indices
Output:
[1] 3 4 5
In this case, we are looking for indices of elements in my_vector
that are greater than 20
. By creating a logical condition (my_vector > 20
), we can then pass it to the which()
function. The output shows that elements greater than 20
are located at indices 3
, 4
, and 5
. This method allows for more flexibility when searching through vectors, especially when dealing with larger datasets.
Using the for Loop
If you prefer a more manual approach, you can also use a for
loop to iterate through the vector and find the index of an element. This method is less common but can be useful for educational purposes or when you want to implement custom logic.
Here’s how you can do it:
my_vector <- c(100, 200, 300, 400, 500)
element_to_find <- 300
index <- -1
for (i in 1:length(my_vector)) {
if (my_vector[i] == element_to_find) {
index <- i
break
}
}
index
Output:
[1] 3
In this example, we define a vector my_vector
containing five elements. We then loop through each element using a for
loop. If we find a match with element_to_find
, we store the index and break out of the loop. The output indicates that 300
is found at index 3
. While this method is more verbose and less efficient than the previous ones, it demonstrates how you can implement your own logic to find indices.
Conclusion
Finding the index of an element in an R vector is a vital skill for anyone working with data in R. Whether you choose to use built-in functions like which()
and match()
, or prefer a more hands-on approach with loops, understanding these methods will enhance your data manipulation capabilities. With practice, you’ll be able to quickly and efficiently locate elements within vectors, making your data analysis tasks much easier. So go ahead and experiment with these techniques in your own R projects!
FAQ
-
How does the which() function work in R?
Thewhich()
function returns the indices of the TRUE values in a logical vector, allowing you to find specific elements based on conditions. -
Can I find multiple indices using match()?
No, thematch()
function only returns the position of the first match. If you need all indices, consider usingwhich()
. -
Is there a way to find indices without using built-in functions?
Yes, you can use afor
loop to manually iterate through the vector and find indices based on your conditions. -
What if the element is not found in the vector?
If the element is not found, bothwhich()
andmatch()
will return an empty result orNA
, indicating that there is no match. -
Are there performance differences between these methods?
Yes, built-in functions likewhich()
andmatch()
are generally more efficient than using loops, especially with larger datasets.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn