How to Check Variable Data Types in R
- Check Variable Data Types in R
-
Use the
typeof()
Function to Check the Data Type of a Variable in R -
Use the
class()
Function to Check the Data Type of a Variable in R -
Use the
sapply()
Function to Check the Data Type of a Variable in R -
Use the
str()
Function to Check the Data Type of Every Variable in a Data Frame in R - Check if a Variable Is a Specific Data Type in R
- Conclusion
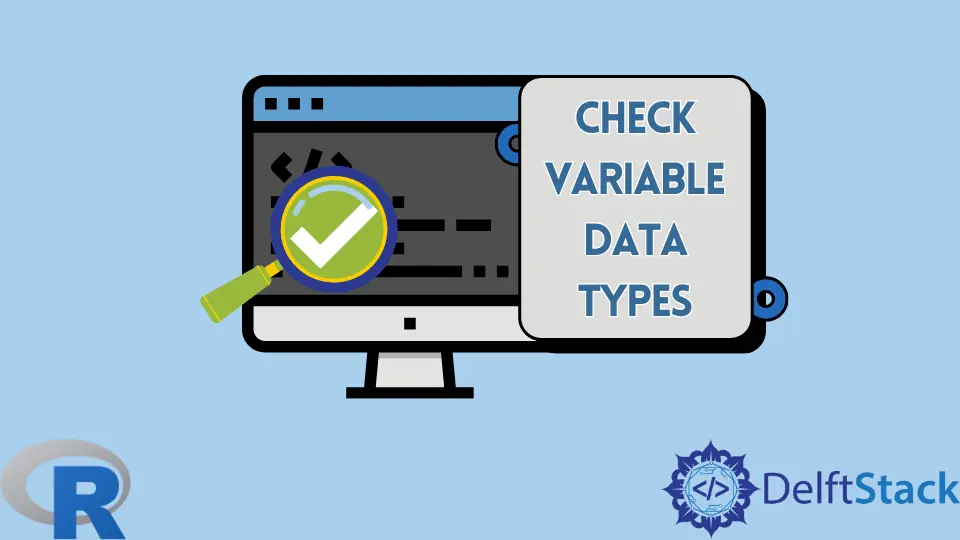
This tutorial will demonstrate how to check the data type of variables in R.
Check Variable Data Types in R
Checking variable data types in R involves determining the type of data stored in a particular variable. Knowing the data type is essential for various reasons, such as ensuring compatibility with operations and functions, identifying potential issues, and guiding data manipulation.
R provides various functions and methods to inspect the data type of a variable. Let’s discuss the methods in the following section.
Use the typeof()
Function to Check the Data Type of a Variable in R
In R, the typeof()
function is used to determine the type of an R object, which includes variables. The typeof()
function returns a string indicating the fundamental data type of the object.
Basic Syntax:
typeof(x)
In the syntax, the x
is the object (variable or value) for which you want to determine the data type. The typeof()
function returns a string representing the fundamental data type of the object x
.
Code:
Demo <- c(
"Delftstack1", "Delftstack2", "Delftstack3", "Delftstack4", "Delftstack5", "Delftstack6"
)
print("The output for typeof method:")
typeof(Demo)
In the code, we first create a vector named Demo
containing six character elements. Subsequently, we use the typeof()
function to check the data type of the entire vector.
The print()
statement is used to display a descriptive message, indicating that we are about to showcase the output for the typeof
method.
Output:
[1] "The output for typeof method:"
[1] "character"
The output reveals that the data type of the entire Demo
vector is a "character"
. Thus, using the typeof()
function on a vector of character elements accurately identifies and confirms their data type, which, in this case, is character
.
We can use the typeof()
function to check the data type of various R objects, including numeric values, characters, logical values, and more.
Use the class()
Function to Check the Data Type of a Variable in R
The class()
function is used to determine the class or classes of an object, which includes variables. The class()
function returns a character vector of class names that represent the type or types of the object.
Basic Syntax:
class(x)
In the syntax, the x
is the object (variable or value) for which you want to determine the class or classes. The class()
function returns a character vector of class names that represent the type or types of the object.
Code:
Demo <- c(
"Delftstack1", "Delftstack2", "Delftstack3", "Delftstack4", "Delftstack5", "Delftstack6"
)
print("The output for class method:")
class(Demo)
In this code, we initialize a vector named Demo
containing six character elements. To ascertain the class or classes of the elements within the vector, we utilize the class()
function.
The print()
statement is employed to announce that we are about to display the output for the class
method.
Output:
[1] "The output for class method:"
[1] "character"
The output indicates that the class of the entire Demo
vector is "character"
.
Similar to typeof()
, we can use the class()
function to check the data type of various R objects, including numeric values, characters, factors, and more. The difference between the two functions is that the typeof()
is more concerned with the underlying representation of data, while class()
is related to the broader object-oriented structure and inheritance of R objects.
Use the sapply()
Function to Check the Data Type of a Variable in R
In R, you can use the sapply()
function to apply another function, such as typeof()
or class()
, to each element of a vector or each column of a data frame. This allows you to check the data type of each element concisely.
Basic Syntax:
output_typeof <- sapply(Demo, typeof)
In the syntax, we use the sapply()
function to apply the typeof()
function to each element of the Demo
vector, storing the results in output_typeof
.
Code:
Demo <- c("Delftstack1", "Delftstack2", "Delftstack3")
output_typeof <- sapply(Demo, typeof)
print("The output for typeof method:")
print(output_typeof)
In this code, we create a vector named Demo
containing three character elements. Employing the sapply()
function, we apply the typeof()
function to each element within the Demo
vector.
The results are stored in a new vector named output_typeof
. Subsequently, we use the print()
statement to convey that we are showcasing the output for the typeof
method.
Output:
[1] "The output for typeof method:"
Delftstack1 Delftstack2 Delftstack3
"character" "character" "character"
The output displayed in each element in the Demo
vector is of the "character"
data type.
In this way, sapply()
provides a convenient way to check the data type or class of each element in a vector or each column in a data frame.
Use the str()
Function to Check the Data Type of Every Variable in a Data Frame in R
The str()
function is used to display the structure of an R object, providing a concise and informative summary. When applied to a data frame, str()
not only shows the data type of every variable but also displays additional details such as the length, class, and a preview of the data.
Basic Syntax:
str(object)
In the syntax, the object
is the R object (e.g., a data frame, list, or vector) for which you want to display the structure. The str()
function provides a concise and informative summary of the internal structure of the object.
Code:
Delftstack <- data.frame(
Name = c("Jack", "John", "Mike", "Michelle", "Jhonny"),
LastName = c("Danials", "Cena", "Chandler", "McCool", "Nitro"),
Id = c(101, 102, 103, 104, 105),
Designation = c("CEO", "Project Manager", "Senior Dev", "Junior Dev", "Intern")
)
Delftstack
str(Delftstack)
In this code, we create a data frame named Delftstack
with four columns: Name
, LastName
, Id
, and Designation
. Each column contains values corresponding to the names, last names, IDs, and designations of individuals.
The Delftstack
data frame is then displayed to the console. Following that, we use the str()
function to provide a structured summary of the data frame’s internal structure, including the data types, lengths, and classes of each variable.
Output:
Name LastName Id Designation
1 Jack Danials 101 CEO
2 John Cena 102 Project Manager
3 Mike Chandler 103 Senior Dev
4 Michelle McCool 104 Junior Dev
5 Jhonny Nitro 105 Intern
'data.frame': 5 obs. of 4 variables:
$ Name : chr "Jack" "John" "Mike" "Michelle" ...
$ LastName : chr "Danials" "Cena" "Chandler" "McCool" ...
$ Id : num 101 102 103 104 105
$ Designation: chr "CEO" "Project Manager" "Senior Dev" "Junior Dev" ...
The output shows a concise overview, indicating that the data frame has five observations and four variables. It further details the data types and classes of each variable within the data frame, aiding in a quick understanding of its structure.
Using str()
is a convenient way to get an overview of the structure and data types of all variables in a data frame.
Check if a Variable Is a Specific Data Type in R
There are a few methods to check if a variable is of a specific type, one method for each data type.
The methods used to check if a variable is of a specific data type are based on the use of functions such as is.numeric()
, is.complex()
, is.character()
, is.logical()
, is.factor()
, and is.integer()
. These functions return a logical value (TRUE
or FALSE
) indicating whether the specified variable belongs to the corresponding data type.
Let’s try an example to show each method.
Code 1:
a <- 3
b <- 5.3
c <- "Delftstack"
d <- TRUE
e <- factor(c("A", "B", "C", "D"))
i <- as.integer(a)
print(is.numeric(a))
print(is.complex(b))
print(is.character(c))
print(is.logical(d))
print(is.factor(e))
print(is.integer(i))
The code above creates a series of variables for each type and checks if it has the corresponding data type.
Output:
[1] TRUE
[1] FALSE
[1] TRUE
[1] TRUE
[1] TRUE
[1] TRUE
The output shows that variable b <- 5.3
is not a complex type variable; all the other variables have the same data type as the method.
We can also use these methods to check the data types for the columns of a data frame.
Code 2:
Delftstack <- data.frame(
Name = c("Jack", "John", "Mike", "Michelle", "Jhonny"),
LastName = c("Danials", "Cena", "Chandler", "McCool", "Nitro"),
Id = c(101, 102, 103, 104, 105),
Designation = c("CEO", "Project Manager", "Senior Dev", "Junior Dev", "Intern")
)
is.character(Delftstack$Name)
is.complex(Delftstack$LastName)
is.numeric(Delftstack$Id)
The code above checks if a column of the data frame is a specific type of data or not.
Output:
[1] TRUE
[1] FALSE
[1] TRUE
The output shows that the variable LastName=c('Danials', 'Cena', 'Chandler', 'McCool', 'Nitro')
in the data frame is not a complex type variable. All the other variables have the same data type as the method.
Conclusion
In conclusion, we explored various methods to check variable data types in R, which is crucial for ensuring compatibility, identifying issues, and guiding data manipulation.
We introduced functions like typeof()
and class()
to inspect fundamental data types and classes of objects, respectively. The sapply()
function was demonstrated as a versatile tool to apply type-checking functions to elements efficiently.
Additionally, we used str()
to provide detailed summaries of data frame structures, including data types, lengths, and classes. The examples illustrated the application of functions like is.numeric()
, is.complex()
, and is.character()
for specific type checking, both for individual variables and data frame columns.
By leveraging these methods, we gain valuable insights into data types, facilitating effective data analysis and manipulation in the R programming language.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook