Where Are Python Packages Installed
-
Use the
pip
Command to List the Packages Installed -
Use the
conda
Command to List the Locally Installed Packages -
Use the
python
Command to List the Packages Installed -
Use the
distutils.sysconfig
Module to List the Packages Installed -
Use the
sysconfig
Module to List the Packages Installed
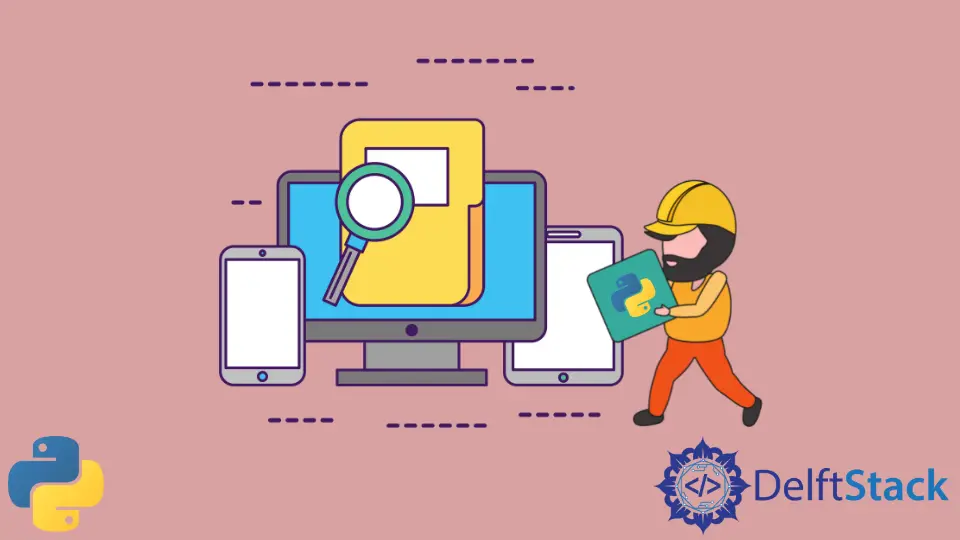
A package in Python can be defined as a directory that contains Python files. These files are usually Python Modules.
As the program grows larger and gets more complex, similar modules are positioned in a package, which helps in making the program easier to manage and have better readability. This approach is often called Modular Programming, and packages help in achieving it.
The file __init__.py
must be contained inside the directory in order for Python to consider it as a Package. This file usually has the initialization code for the package, but it can be left empty.
This tutorial will discuss different methods to find the directories in which python packages are installed.
Use the pip
Command to List the Packages Installed
In Python, the packages can be installed both globally and locally.
A package, when installed globally, is available to all the users in the system. The same package, when installed locally, would only be available to the user that manually installed it.
By default, the pip
command installs the packages globally.
The following code uses the pip
command to list the packages installed globally.
# we can also use "pip list command"
pip freeze
Although, by default, the pip
command installs packages globally, the packages that have been manually installed locally can also be seen using this command.
The following code uses the pip
command to list the packages installed locally.
# we can also use "pip list --user"
pip freeze --user
Use the conda
Command to List the Locally Installed Packages
This method works only for programmers working on Anaconda IDE. It is possible to list the locally installed package in a conda
environment. To execute this, we just have to write a single line of code in the Anaconda prompt.
The following code uses conda
to list the packages installed locally.
conda list
Use the python
Command to List the Packages Installed
The python
command can be used to find the package-site directories.
Global Site Packages
The global site packages are found to be listed in sys.path
.
The following code uses the python
command to list the globally installed packages.
python -m site
The site
module can also be used along with the python command to get a better and more concise list of packages. This method uses the getsitepackages()
from the site
module.
The following code uses the python
command along with the site
module to list the globally installed packages.
python -c 'import site; print(site.getsitepackages())'
Note that the getsitepackages()
function is not available with virtualenv.
Locally Installed Packages
The local packages are installed in the per-user
site-packages directory (PEP 370).
The following code uses the python
command to list the locally installed packages.
python -m site --user-site
Use the distutils.sysconfig
Module to List the Packages Installed
The distutils
package can be utilized to provide functions for installing and building additional modules into a Python installation. In this case, it can be used to list the packages as well.
The following code uses the distutils.sysconfig
to list the globally installed packages.
python -c "from distutils.sysconfig import get_python_lib; print(get_python_lib())"
The only drawback is that it points us to the directory of the dist-packages
or the packages automatically installed by the Operating System.
Use the sysconfig
Module to List the Packages Installed
In Python 3 and above, the sysconfig
module is available to use for listing the packages installed.
The sysconfig
module isn’t to be mistaken with the distutils.sysconfig
submodule that was mentioned above. The latter is an altogether different module and it’s deficient in the get_paths
function that will be used here.
The following code uses the sysconfig
module to list the installed packages.
python -c "import sysconfig; print(sysconfig.get_path('purelib'))"
We use the purelib
path here, which is where the standard Python packages are installed, with the help of tools like pip
.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn