How to Use of defaultdict in Python
-
defaultdict
vsdict
in Python -
the
defaultdict
in Python -
Useful Functions of the
defaultdict
in Python
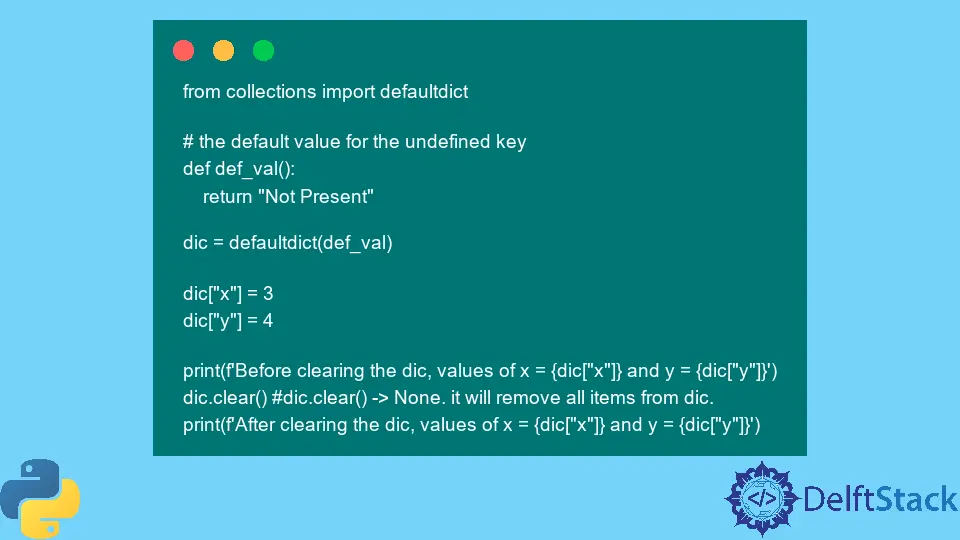
Today’s article discusses the defaultdict
container and demonstrates its use using code examples.
defaultdict
vs dict
in Python
The defaultdict
is a container like a dictionary that belongs to the collections module. It’s a sub-class of the dictionary; hence it has all the functionalities of dictionary
. However, the sole purpose of defaultdict
is to handle the KeyError
.
# return true if the defaultdict is a subclass of dict (dictionary)
from collections import defaultdict
print(issubclass(defaultdict, dict))
Output:
True
Let’s say a user searches an entry in the dictionary
, and the searched entry does not exist. The ordinary dictionaries will arise a KeyError
, which means the entry does not exist. To overcome this issue, Python developers presented the concept of defaultdict
.
Code Example:
# normal dictionary
ord_dict = {"x": 3, "y": 4}
ord_dict["z"] # --> KeyError
Output:
KeyError: 'z'
The ordinary dictionary can’t handle unknown keys
, and it throws KeyError
when we search for an unknown key
, as demonstrated in the above code.
On the other hand, the defaultdict
module works similarly to Python dictionaries. Still, it has advanced, helpful and user-friendly features and doesn’t throw an error when a user searches for an undefined key in the dictionary.
But instead, it creates an entry in the dictionary and returns a default value against the key
. To understand this concept, let’s look at the practical part below.
Code Example:
from collections import defaultdict
# the default value for the undefined key
def def_val():
return "The searched Key Not Present"
dic = defaultdict(def_val)
dic["x"] = 3
dic["y"] = 4
# search 'z' in the dictionary 'dic'
print(dic["z"]) # instead of a KeyError, it has returned the default value
print(dic.items())
Output:
The searched Key Not Present
dict_items([('x', 3), ('y', 4), ('z', 'The searched Key Not Present')])
The defaultdict
creates any items we try to access with the key, which is undefined in the dictionary.
And create such a default
item, it calls the function object that we pass to the constructor of the defaultdict
, and to be more precise, the object should be a callable
object that includes type objects and functions.
In the above example, the default items are created with the def_val
function that returns a string The searched Key Not Present
against the undefined key in the dictionary.
the defaultdict
in Python
The default_factory
is the first argument to the defaultdict
constructor which is used by the __missing__()
method, and if the argument of the constructor is missing the default_factory
will be initialized as None
which will arise the KeyError
.
And if the default_factory
is initialized with something other than None
, it will be assigned as a value
to a searched key
as seen in an example above.
Useful Functions of the defaultdict
in Python
There are tons of functions of dictionary
and defaultdict
. As we know, the defaultdict
has access to all the functions of dictionary
; however, these are some of the most useful functions specific to defaultdict
.
defaultdict.clear()
in Python
Code Example:
from collections import defaultdict
# the default value for the undefined key
def def_val():
return "Not Present"
dic = defaultdict(def_val)
dic["x"] = 3
dic["y"] = 4
print(f'Before clearing the dic, values of x = {dic["x"]} and y = {dic["y"]}')
dic.clear() # dic.clear() -> None. it will remove all items from dic.
print(f'After clearing the dic, values of x = {dic["x"]} and y = {dic["y"]}')
Output:
Before clearing the dic, values of x = 3 and y = 4
After clearing the dic, values of x = Not Present and y = Not Present
As we see in the above example, we have two pairs of data in the dictionary dic
where x=3
and y=4
. However, after using the clear()
function, data has been removed, and the values of x
and y
do not exist anymore, and that’s why we are getting Not present
against x
and y
.
defaultdict.copy()
in Python
Code Example:
from collections import defaultdict
# the default value for the undefined key
def def_val():
return "Not Present"
dic = defaultdict(def_val)
dic["x"] = 3
dic["y"] = 4
dic_copy = dic.copy() # dic.copy will give you a shallow copy of dic.
print(f"dic = {dic.items()}")
print(f"dic_copy = {dic_copy.items()}")
Output:
dic = dict_items([('x', 3), ('y', 4)])
dic_copy = dict_items([('x', 3), ('y', 4)])
The defaultdict.copy()
function is used to copy a shallow copy of the dictionary into another variable which we can use accordingly.
defaultdict.default_factory()
in Python
Code Example:
from collections import defaultdict
# the default value for the undefined key
def def_val():
return "Not present"
dic = defaultdict(def_val)
dic["x"] = 3
dic["y"] = 4
print(f"The value of z = {dic['Z']}")
print(
dic.default_factory()
) # default_factory returns the default value for defaultdict.
Output:
The value of z = Not present
Not present
The default_factory()
function is used to provide default values to the attributes of a defined class, and generally, the values of the default_factory
are the values returned by a function.
defaultdict.get(key, default value)
in Python
Code Example:
from collections import defaultdict
# the default value for the undefined key
def def_val():
return "Not present"
dic = defaultdict(def_val)
dic["x"] = 3
dic["y"] = 4
# search the value of Z in the dictionary dic; if it exists, return the value; otherwise, display the message
print(dic.get("Z", "Value doesn't exist")) # default value is None
Output:
Value doesn't exist
The defaultdict.get()
function takes two arguments first is the key, and the second is the default value against the key in case the value doesn’t exist.
But the second argument is optional. So we can specify any message or value; otherwise, it will display None
as a default value.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn