Python での defaultdict の使用
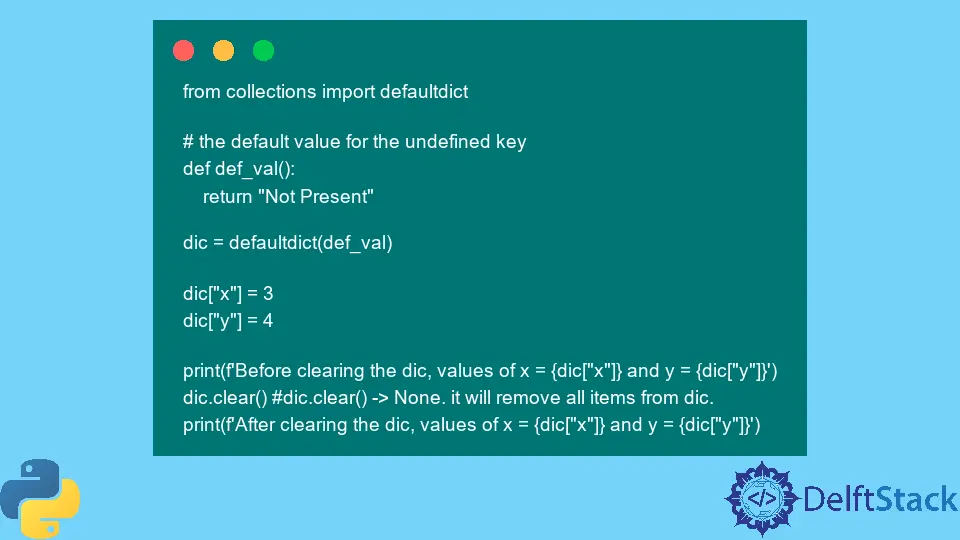
今日の記事では、defaultdict
コンテナーについて説明し、コード例を使用してその使用法を示します。
Python のdefaultdict
とdict
defaultdict
は collections モジュールに属する辞書のような コンテナ です。 これはディクショナリのサブクラスです。 したがって、それは dictionary
のすべての機能を備えています。 ただし、defaultdict
の唯一の目的は、KeyError
を処理することです。
# return true if the defaultdict is a subclass of dict (dictionary)
from collections import defaultdict
print(issubclass(defaultdict, dict))
出力:
True
ユーザーが dictionary
のエントリを検索し、検索されたエントリが存在しないとします。 通常の辞書では、エントリが存在しないことを意味する KeyError
が発生します。 この問題を克服するために、Python 開発者は defaultdict
の概念を提示しました。
コード例:
# normal dictionary
ord_dict = {"x": 3, "y": 4}
ord_dict["z"] # --> KeyError
出力:
KeyError: 'z'
通常の辞書は不明な keys
を処理できず、上記のコードで示されているように、未知の key
を検索すると KeyError
がスローされます。
一方、defaultdict
モジュールは Python 辞書と同様に機能します。 それでも、高度で便利で使いやすい機能を備えており、ユーザーが辞書で未定義のキーを検索してもエラーは発生しません。
しかし代わりに、辞書にエントリを作成し、key
に対してデフォルト値を返します。 この概念を理解するために、以下の実用的な部分を見てみましょう。
コード例:
from collections import defaultdict
# the default value for the undefined key
def def_val():
return "The searched Key Not Present"
dic = defaultdict(def_val)
dic["x"] = 3
dic["y"] = 4
# search 'z' in the dictionary 'dic'
print(dic["z"]) # instead of a KeyError, it has returned the default value
print(dic.items())
出力:
The searched Key Not Present
dict_items([('x', 3), ('y', 4), ('z', 'The searched Key Not Present')])
defaultdict
は、ディクショナリで定義されていないキーでアクセスしようとするアイテムを作成します。
そのような default
アイテムを作成すると、defaultdict
のコンストラクターに渡す関数オブジェクトが呼び出されます。より正確には、オブジェクトは、型オブジェクトと関数を含む callable
オブジェクトである必要があります。
上記の例では、ディクショナリ内の未定義のキーに対して、文字列 検索されたキーが存在しません
を返す def_val
関数を使用して、デフォルト アイテムが作成されます。
Python の defaultdict
default_factory
は、__missing__()
メソッドによって使用される defaultdict
コンストラクターへの 最初の引数 であり、コンストラクターの引数が欠落している場合、default_factory
は None
として初期化されます。 KeyError
が発生します。
また、default_factory
が None
以外で初期化されている場合、上記の例に見られるように、検索された key
に value
として割り当てられます。
Python の defaultdict
の便利な関数
dictionary
と defaultdict
の機能は山ほどあります。 ご存知のように、defaultdict
は dictionary
のすべての機能にアクセスできます。 ただし、これらは defaultdict
に固有の最も便利な関数の一部です。
Python の defaultdict.clear()
コード例:
from collections import defaultdict
# the default value for the undefined key
def def_val():
return "Not Present"
dic = defaultdict(def_val)
dic["x"] = 3
dic["y"] = 4
print(f'Before clearing the dic, values of x = {dic["x"]} and y = {dic["y"]}')
dic.clear() # dic.clear() -> None. it will remove all items from dic.
print(f'After clearing the dic, values of x = {dic["x"]} and y = {dic["y"]}')
出力:
Before clearing the dic, values of x = 3 and y = 4
After clearing the dic, values of x = Not Present and y = Not Present
上記の例でわかるように、辞書 dic
には x=3
と y=4
の 2つのデータのペアがあります。 ただし、clear()
関数を使用した後、データは削除され、x
と y
の値はもう存在しません。そのため、x
と y に対して
Not presentを取得しています。
.
Python の defaultdict.copy()
コード例:
from collections import defaultdict
# the default value for the undefined key
def def_val():
return "Not Present"
dic = defaultdict(def_val)
dic["x"] = 3
dic["y"] = 4
dic_copy = dic.copy() # dic.copy will give you a shallow copy of dic.
print(f"dic = {dic.items()}")
print(f"dic_copy = {dic_copy.items()}")
出力:
dic = dict_items([('x', 3), ('y', 4)])
dic_copy = dict_items([('x', 3), ('y', 4)])
defaultdict.copy()
関数は、辞書の浅いコピーを別の変数にコピーするために使用され、それに応じて使用できます。
Python の defaultdict.default_factory()
コード例:
from collections import defaultdict
# the default value for the undefined key
def def_val():
return "Not present"
dic = defaultdict(def_val)
dic["x"] = 3
dic["y"] = 4
print(f"The value of z = {dic['Z']}")
print(
dic.default_factory()
) # default_factory returns the default value for defaultdict.
出力:
The value of z = Not present
Not present
default_factory()
関数は、定義されたクラスの属性にデフォルト値を提供するために使用されます。通常、default_factory
の値は関数によって返される値です。
Python の defaultdict.get(key, default value)
コード例:
from collections import defaultdict
# the default value for the undefined key
def def_val():
return "Not present"
dic = defaultdict(def_val)
dic["x"] = 3
dic["y"] = 4
# search the value of Z in the dictionary dic; if it exists, return the value; otherwise, display the message
print(dic.get("Z", "Value doesn't exist")) # default value is None
出力:
Value doesn't exist
defaultdict.get()
関数は 2つの引数を取ります。最初はキーで、2 番目は値が存在しない場合のキーに対するデフォルト値です。
ただし、2 番目の引数はオプションです。 したがって、任意のメッセージまたは値を指定できます。 それ以外の場合は、デフォルト値として None
が表示されます。
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn