Difference Between Multiprocessing and Threading in Python
- Difference Between Threads and Multiprocessing in Python
-
Use the
multiprocessing
Module to Perform Multiprocessing in Python -
Use the
threading
Module to Perform Multithreading in Python
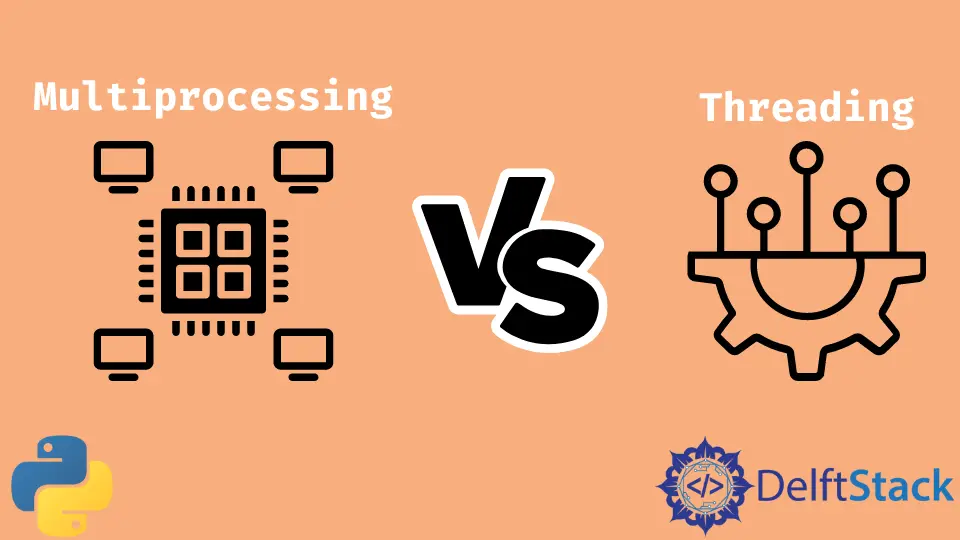
Multiprocessing and threading are techniques that can speed up the execution of your code by breaking down your program into smaller tasks.
This tutorial will demonstrate multiprocessing vs. threading in Python.
Difference Between Threads and Multiprocessing in Python
Threads are a small compilation of instructions to control the execution flow, and a process can be divided into several threads to improve efficiency.
In Python, we use the threading
module to perform multithreading. A process is generally divided into several threads to perform smaller tasks.
The Global Interpreter Locker (GIL) ensures that the threads do not run parallelly in Python and execute one after another concurrently.
On the other hand, multiprocessing is a technique where processes run across multiple CPU cores. This method achieves parallelism by running several processes simultaneously.
These processes may be divided into several threads, and every child process has its own memory space. Both techniques allow the code to run concurrently, and each method has its advantages and disadvantages.
We will now demonstrate two simple programs for multiprocessing and threading. Let us start with multiprocessing.
Use the multiprocessing
Module to Perform Multiprocessing in Python
Multiprocessing runs several processes at once. Every process has its own space and runs without interfering with each other.
It is a little straightforward and takes full advantage of the different cores of the CPU. However, spawning multiple processes takes time and a lot of memory.
It is generally used for CPU-bound tasks. To perform multiprocessing in Python, we use the multiprocessing
module. See the code below.
import multiprocessing
def fun():
print("Sample Process")
for i in range(5):
p = multiprocessing.Process(target=fun)
p.start()
Output:
Sample Process
Sample Process
Sample Process
Sample Process
Sample Process
The multiprocessing.Process
constructor creates a Process
class object in the above example. The target
parameter is used to provide the target function which needs to execute when the process starts.
We can also specify some arguments using the args
parameter in the multiprocessing.Process
constructor. The start()
method will start the process.
The join()
method can be used to stop the execution of the program till a process stops executing.
Use the threading
Module to Perform Multithreading in Python
Threads do not require much memory, and the data is shared between multiple threads. They also require very few system calls and are a great option for I/O applications or if the program is network-bound.
Threading makes the application more responsive but can be complicated to execute. Also, if a single thread crashes, the entire program may get affected.
Race conditions may exist in Python; one or more threads try to access the same resource. Similarly, we use the threading
module to perform multithreading in Python.
See the code below.
import threading
def fun():
print("Sample Thread")
for i in range(5):
p = threading.Thread(target=fun)
p.start()
Output:
Sample Thread
Sample Thread
Sample Thread
Sample Thread
Sample Thread
The threading.Thread
constructor instantiates an object of the Thread
class. We specify the ’ Target ’ function like the Process
object.
The start()
function starts the thread. We can also use the join()
method with threads. Additionally, we can use a Lock
object to prevent the race condition in Python.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn