How to Wrap Text in Python
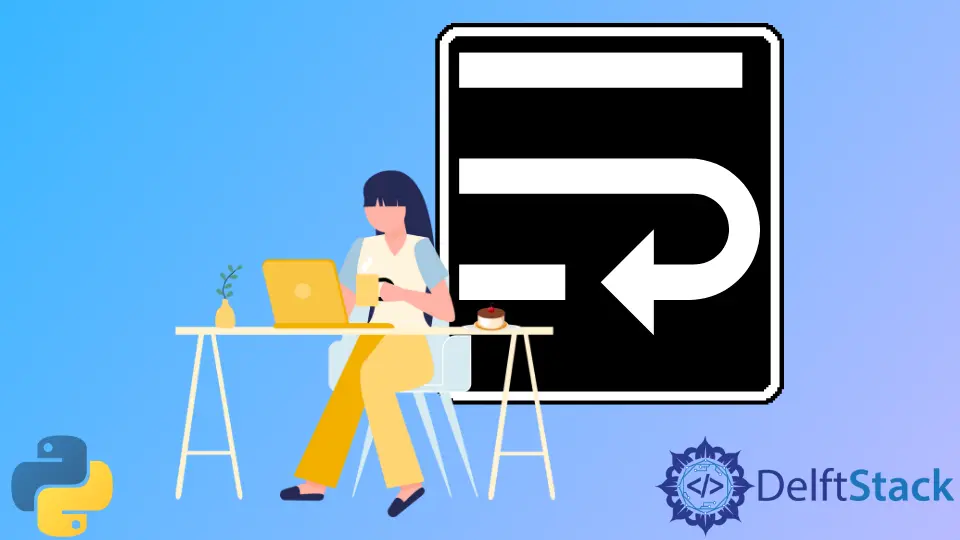
We will introduce how we can wrap text in python. Python has a built-in module, textwrap
, that can help us achieve this functionality.
This tutorial will go through different examples using the textwrap
module.
Text Wrap in Python
Many situations require us to wrap the strings to improve visibility, readability, or other reasons. We may need to wrap the text for certain screen size so the text doesn’t overflow beyond the size of the screen.
If we wrap the text based on the number of characters per line, it can break the words and make it sometimes unreadable and decreases user experience. The textwrap
module provides different methods that can be used to wrap long text.
Use the wrap()
Method in Python
One commonly used method is wrap(content, width=length)
. Content is the text we need to wrap, and width represents the number of characters per line.
The default number of characters is 70
. After wrapping the text, we will use the join()
method to join the lines into a single string.
Example Code:
# python
import textwrap
content = "Lorem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry's standard dummy text ever since the 1500s, when an unknown printer took a galley of type and scrambled it to make a type specimen book. It has survived not only five centuries, but also the leap into electronic typesetting, remaining essentially unchanged. It was popularised in the 1960s with the release of Letraset sheets containing Lorem Ipsum passages, and more recently with desktop publishing software like Aldus PageMaker including versions of Lorem Ipsum."
wrappedText = textwrap.wrap(content)
print("\n".join(wrappedText))
Output:
Our text was easily wrapped without breaking the words from the above example.
Now let’s go through another example in which we will discuss what to do if we want to display only a certain number of lines from a string or a text paragraph.
We will print the same text paragraph, but we will limit the number of characters per line to increase the number of lines and will display only 10 lines and place a placeholder in place of the remaining lines, as shown below.
Example Code:
# python
import textwrap
content = "Lorem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry's standard dummy text ever since the 1500s, when an unknown printer took a galley of type and scrambled it to make a type specimen book. It has survived not only five centuries, but also the leap into electronic typesetting, remaining essentially unchanged. It was popularised in the 1960s with the release of Letraset sheets containing Lorem Ipsum passages, and more recently with desktop publishing software like Aldus PageMaker including versions of Lorem Ipsum."
# Without Limiting Number of Lines
wrappedText = textwrap.wrap(content, width=40)
print("Number of Lines : {}\n".format(len(wrappedText)))
print("\n".join(wrappedText))
# With Limiting Number of Lines
wrappedText = textwrap.wrap(
content, width=40, max_lines=10, placeholder="more content....."
)
print("Number of Lines : {}\n".format(len(wrappedText)))
print("\n".join(wrappedText))
Output for without limiting the number of lines:
Output for limiting the number of lines:
We can not only limit the number of characters per line but also limit the number of lines to display, and we can also place a placeholder in place of the remaining lines.
Use the fill()
Method in Python
The wrap()
method returns a list of lines instead of returning the whole paragraph with wrapped text.
But for this purpose, Python provides another method called fill()
, which functions the same as the wrap()
method but returns a single string combining each line instead of returning a list of lines.
Example Code:
# python
import textwrap
content = "Lorem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry's standard dummy text ever since the 1500s, when an unknown printer took a galley of type and scrambled it to make a type specimen book. It has survived not only five centuries, but also the leap into electronic typesetting, remaining essentially unchanged. It was popularised in the 1960s with the release of Letraset sheets containing Lorem Ipsum passages, and more recently with desktop publishing software like Aldus PageMaker including versions of Lorem Ipsum."
wrappedText = textwrap.fill(content)
print(wrappedText)
print("\n")
wrappedText = textwrap.fill(content, width=40)
print("\n")
print(wrappedText)
wrappedText = textwrap.fill(
content, width=40, max_lines=12, placeholder=" [..More Content]"
)
print("\n")
print(wrappedText)
Output:
This tutorial discussed the wrap()
method from the textwrap
module and its parameters. We also discussed the fill()
method and its parameters.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn