How to Split a String on New Line in Python
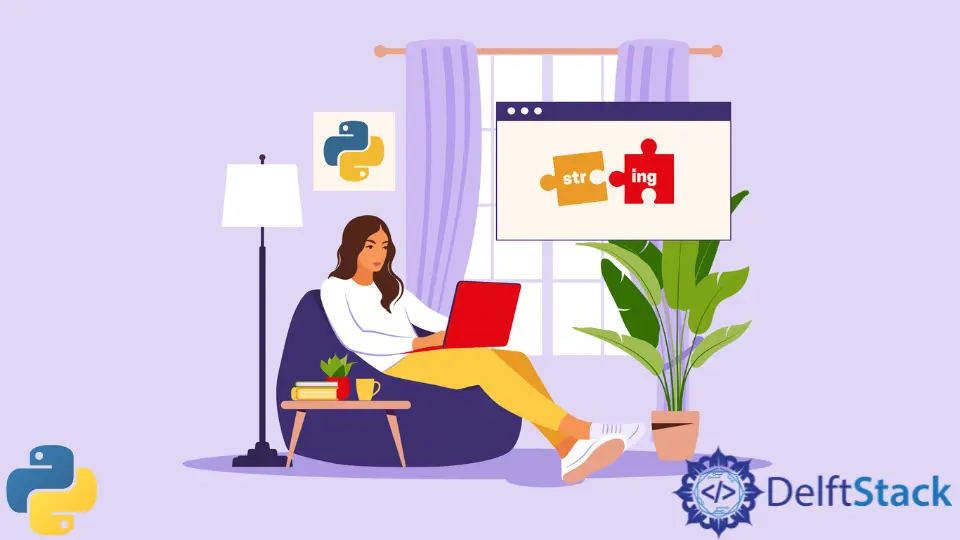
Most of the time while working with the strings, we usually face a situation where we want to break a big string into separate into lines. In this article, we will learn how to split the big string into smaller pieces of text and also how we can divide the big string into separate lines in Python
. A string split is a method that further divides or splits the words of the string into smaller pieces.
By working with Strings in other programming languages we came to know about concatenation (combining the small pieces of strings) and String split is just the opposite concept of it. If you want to perform the split operation on any string, Python provides you with various built-in functions, but one of them is called split()
.
The python split()
method is used to break the string into smaller chunks or we can say, the split()
method splits a string into a list of characters. It breaks the string at line boundaries and returns a list of characters with index, it also gets one argument called a separator. A separator in a code is nothing but a character or just a symbol. If there is no separator is given, then it will split the mentioned string and whitespace will be used by default.
The syntax for splitting any string in Python is following:
variable_name = "String value"
variable_name.split()
Split a String on New Lines in Python
There are various ways to split the string into smaller words or onto new lines. We will see each method with examples below:
If you want to split the whole string using a new line separator then you have to pass the \n
. The string has the next line separator \n
characters too in the string as shown below, instead of a multi-line string with triple quotes.
sentence = "Hi\nHow are you\n doing Where\n are you"
char = sentence.split("\n")
print(char)
Output:
['Hi', 'How are you', ' doing Where', ' are you']
If you want to split the whole string using using the splitlines()
function, it will break the lines by line boundaries. If you want the line breaks are included then you need to pass True
to the splitlines()
function:
sentence = "First Line\n\nThird Line\r Forth Line \r\n"
char = sentence.splitlines()
print(char)
Output:
['First Line', '', 'Third Line', ' Forth Line ']
sentence = "First Line\n\nThird Line\r Forth Line \r\n"
char = sentence.splitlines()
print(char)
Output:
['First Line\n', '\n', 'Third Line\r', ' Forth Line \r\n']
Abdul is a software engineer with an architect background and a passion for full-stack web development with eight years of professional experience in analysis, design, development, implementation, performance tuning, and implementation of business applications.
LinkedIn