How to Sort a Set in Python
-
Use the
sorted()
Function to Sort a Set in Python -
Use the
sort()
Method to Sort a Set in Python - Conclusion
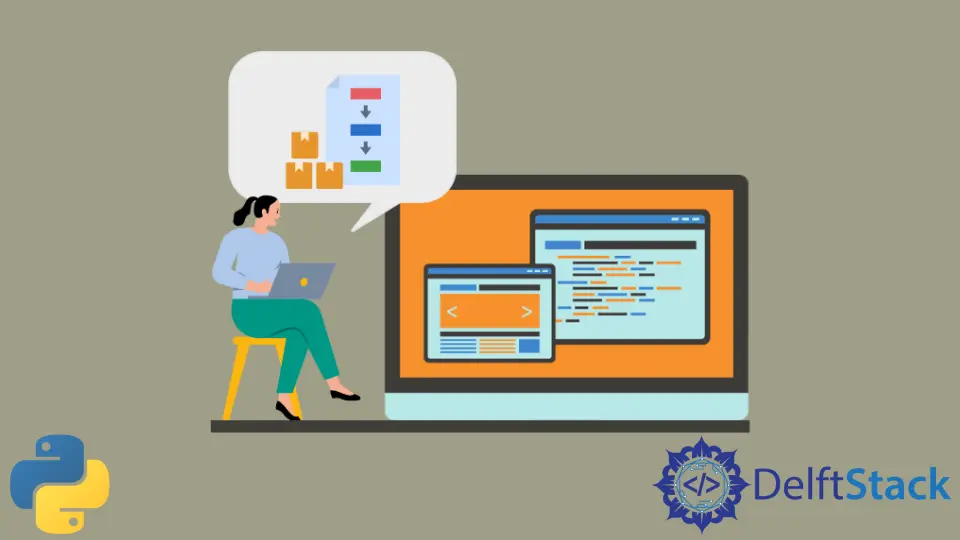
Sets are a fundamental data type in Python, characterized by their unique and unordered nature. These collections contain no duplicate elements and are enclosed within curly braces.
They offer a versatile way to work with unique values, such as integers, strings, or any other hashable objects.
The most distinctive feature of sets is their lack of ordering. Unlike lists or tuples, sets do not maintain any specific sequence or index for their elements, which means that when you iterate through a set, you can’t predict the order in which elements will appear.
This property is a direct consequence of sets being implemented as hash tables.
While sets themselves are inherently unordered, when you print the contents of a set, you might notice that the elements are displayed in a sorted manner. However, it’s essential to understand that this sorted presentation is merely a side effect of how Python internally manages the elements within the set.
Here’s an example of printing a set:
s = {5, 2, 7, 1, 8}
print(s)
Output:
{1, 2, 5, 7, 8}
The order in which the elements appear here is not guaranteed to be the same every time you print the set. It depends on the internal hashing and indexing mechanism used by Python to store and display set elements.
Use the sorted()
Function to Sort a Set in Python
The sorted()
function is a versatile tool for sorting data in Python. It works with various iterable data structures, including sets, lists, tuples, and more.
Its basic syntax is as follows:
sorted(iterable, key=None, reverse=False)
Let’s break down its parameters:
iterable
: The iterable you want to sort, such as a set, list, tuple, or string.key
(optional): A function that defines the criteria for sorting. If not specified, the default sorting order is ascending.reverse
(optional): If set toTrue
, the iterable is sorted in descending order; otherwise, it’s sorted in ascending order (default).
Sorting Sets in Ascending Order
To sort a set of values in ascending order, use the sorted()
function like this:
original_set = {5, 3, 1, 4, 2}
sorted_set = sorted(original_set)
print(sorted_set)
Output:
[1, 2, 3, 4, 5]
The original_set
remains unaltered, and the sorted values are stored in sorted_set
.
Custom Sorting With the key
Parameter
The key
parameter allows you to define a custom sorting criterion. For example, to sort a set of strings in reverse order, you can use a lambda function with key
:
original_set = {"apple", "banana", "cherry", "date"}
sorted_set = sorted(original_set, key=lambda x: x[::-1])
print(sorted_set)
Output:
['banana', 'apple', 'date', 'cherry']
This sorts the strings based on their reversed order, thanks to the custom sorting key.
Sorting in Descending Order
If you need to sort in descending order, set the reverse
parameter to `True:
original_set = {5, 3, 1, 4, 2}
sorted_set = sorted(original_set, reverse=True)
print(sorted_set)
Output:
[5, 4, 3, 2, 1]
The sorted_set
now contains the values in descending order.
As you can see, by changing the reverse
parameter, you have the flexibility to adapt the sorting process to your specific needs. This capability enhances your control over how data is presented or processed in your programs.
Sorting Complex Data
Python’s sorted()
function isn’t limited to simple values. You can sort sets containing more complex data structures or objects by specifying a custom sorting key.
Consider a scenario where you have a list of custom objects, and you want to sort them based on a specific attribute. Here’s how it’s done:
class CustomObject:
def __init__(self, name, value):
self.name = name
self.value = value
objects = [CustomObject("A", 3), CustomObject("B", 1), CustomObject("C", 2)]
sorted_objects = sorted(objects, key=lambda obj: obj.value)
for obj in sorted_objects:
print(obj.name, obj.value)
Output:
B 1
C 2
A 3
In this example, we’ve defined a CustomObject
class with two attributes: name
and value
. We have a list of CustomObject
instances, and we want to sort them based on the value
attribute.
We achieve this by using the key
parameter with a lambda function that extracts the value
attribute for sorting.
By customizing the key
function, you have full control over the sorting process. For example, you can sort by timestamps, alphabetical order, or any other attribute that’s relevant to your data.
Use the sort()
Method to Sort a Set in Python
The sort()
method is available for lists, not sets, in Python. It arranges the elements of a list in ascending or descending order in place.
Unlike the sorted()
function, which creates a new sorted list, the sort()
method modifies the original list in place.
Here is the basic syntax of the sort()
method:
list.sort(key=None, reverse=False)
Let’s examine its parameters:
key
(optional): A function that defines the sorting criteria. If not provided, the default sorting order is ascending.reverse
(optional): If set toTrue
, the list is sorted in descending order; otherwise, it’s sorted in ascending order (default).
Sorting a Set in Ascending Order
Sets in Python are inherently unordered collections of unique elements. Unlike lists, they don’t have an index, and they lack the sort()
method that lists have.
Therefore, you cannot use the sort()
method to sort a set directly.
However, if you want to sort the elements of a set and create a sorted list from it, you can do so by converting the set to a list and then using the sort()
method on the list. Here’s how you can achieve this:
my_set = {5, 1, 3, 2, 4}
my_list = list(my_set)
my_list.sort()
print(my_list)
Output:
[1, 2, 3, 4, 5]
In this example, we first convert the set my_set
to a list using the list()
constructor. Then, we use the sort()
method on the list to sort its elements in ascending order.
Remember that the resulting list will no longer maintain the uniqueness property of the set, as lists can contain duplicates. If you need to maintain uniqueness while sorting, you can convert the sorted list back to a set:
sorted_set = set(my_list)
print(sorted_set)
This will give you a sorted set without duplicates.
Sorting a Set in Descending Order
If you want to sort a set in descending order, you can convert the set to a list and then use the sort()
method with the reverse=True
parameter to sort the list in descending order. Here’s how you can do it:
my_set = {5, 1, 3, 2, 4}
my_list = list(my_set)
my_list.sort(reverse=True)
print(my_list)
Output:
[5, 4, 3, 2, 1]
In this example, we first convert the set my_set
to a list using the list()
constructor. Then, we use the sort()
method with reverse=True
to sort the list in descending order.
Sorting a Set With the key
Parameter
To use the key
parameter to customize the sorting of a set, you’ll need to convert the set to a list first, then sort the list with the specified key function.
Here’s an example of how you can sort a set in descending order based on a custom sorting key:
my_set = {5, 1, 3, 2, 4}
my_list = list(my_set)
def custom_key(x):
return -x
my_list.sort(key=custom_key)
print(my_list)
In this example, we first convert the set my_set
to a list using the list()
constructor. Then, we define a custom sorting key function called custom_key
.
The key function takes an element x
and returns a value that determines the sort order. To achieve descending order, we return the negative of the element’s value.
Finally, we use the sort()
method with the key
parameter to sort the list using the custom key function, resulting in a sorted list in descending order based on the custom key.
Sorting a Set With the key
and reverse
Parameters
If you want to use both the key
and reverse
parameters when sorting a set, you can follow the same approach as before by first converting the set to a list and then sorting the list with both parameters. Here’s an example of how to do it:
my_set = {5, 1, 3, 2, 4}
my_list = list(my_set)
def custom_key(x):
return -x
my_list.sort(key=custom_key, reverse=True)
print(my_list)
Output:
[1, 2, 3, 4, 5]
In this example, we first convert the set my_set
to a list using the list()
constructor. Then, we define a custom sorting key function, custom_key
, and set it to return the negative value of the element to achieve descending order.
Next, we use the sort()
method with both the key
parameter (using the custom key function) and the reverse
parameter set to True
to sort the list in descending order based on the custom key. The result is a sorted list with both the custom sorting key and in descending order.
Conclusion
To exert more control over the sorting of sets, Python provides two main methods: the sorted()
function and the sort()
method.
The sorted()
function is a versatile tool for sorting various iterable data structures, including sets, in ascending or descending order. It also enables you to create custom sorting criteria using the key
parameter, offering flexibility in how data is presented and processed in your programs.
The sort()
method, on the other hand, is specifically available for lists, not sets. To sort a set using the sort()
method, you must first convert the set to a list.
This method allows you to sort the list in ascending or descending order, as well as apply custom sorting criteria using the key
parameter.
Choosing between the sorted()
function and the sort
method depends on the data structure you’re working with and your specific sorting requirements.