How to Get Cookie Using Python Selenium
- Install Prerequisite Libraries
- Get Named Cookies Using Selenium in Python
- Get All Cookies Using Selenium in Python
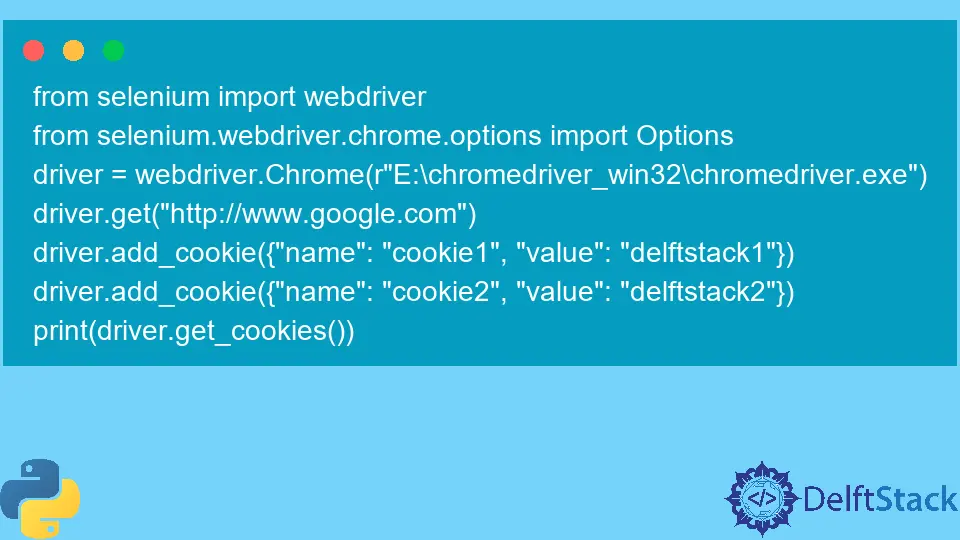
Selenium is a famous testing framework for web applications. We write scripts that take control of the web browser and perform specific actions.
This article will go through a step-by-step procedure to set up Selenium on Linux, get cookies, and format them in an HTTP request.
Install Prerequisite Libraries
To use the plotting of Selenium, we first need to install the Selenium and browser driver, using ChromeDriver
. We can easily achieve this by running the command below in the terminal of choice:
Install ChromeDriver
ChromeDriver
is another executable that Selenium WebDriver
uses to interact with Chrome. To automate tasks on the Chrome web browser, we also need to install ChromeDriver
.
Based on the version of the Chrome browser, we need to select a compatible driver for it. Following are the steps to install and configure the ChromeDriver
:
-
Click on this link. Download
ChromeDriver
according to your Chrome browser’s version and the operating system’s type. -
If you want to find the version of your Chrome browser, click on the three dots on the top right corner of Chrome, click on
Help
, and selectAbout Google Chrome
. You can see the Chrome version in theAbout
section. -
Extract the zip file and run the Chrome driver.
Install Selenium
To install Selenium, we use the following command.
pip install selenium
It’s also important to ensure we work with the correct Python version. In this article, we are using version 3.10.4
.
We can check the currently installed Python version by running this command in the terminal:
python --version
And now we are all set to go!
Get Named Cookies Using Selenium in Python
We imported the required libraries in the following code and specified the path to the Chrome driver. Make sure to change the path accordingly when using this code.
Then we navigated to the URL (http://www.google.com in our case) and added the cookie with our defined name and value for the current browser’s context. To get the cookie, we used the get_cookie()
method and passed the cookie name as an argument to get its value.
Example Code:
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
driver = webdriver.Chrome(r"E:\chromedriver_win32\chromedriver.exe")
driver.get("http://www.google.com")
driver.add_cookie({"name": "my_cookie", "value": "delftstack"})
print(driver.get_cookie("my_cookie"))
Output:
{'domain': 'www.google.com', 'httpOnly': False, 'name': 'my_cookie', 'path': '/', 'secure': True, 'value': 'delftstack'}
Get All Cookies Using Selenium in Python
We used the get_cookies()
method to get all cookies without specifying any argument. In this case, it returns serialized cookie data for the current browsing context.
However, it returns an error if the browser is no longer available.
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
driver = webdriver.Chrome(r"E:\chromedriver_win32\chromedriver.exe")
driver.get("http://www.google.com")
driver.add_cookie({"name": "cookie1", "value": "delftstack1"})
driver.add_cookie({"name": "cookie2", "value": "delftstack2"})
print(driver.get_cookies())
Output:
[{'domain': 'www.google.com', 'httpOnly': False, 'name': 'cookie2', 'path': '/', 'secure': True, 'value': 'delftstack2'}, {'domain': 'www.google.com', 'httpOnly': False, 'name': 'cookie1', 'path': '/', 'secure': True, 'value': 'delftstack1'}, {'domain': '.google.com', 'expiry': 1683449080, 'httpOnly': True, 'name': 'NID', 'path': '/', 'sameSite': 'None', 'secure': True, 'value': '511=jG4Loar3RnUPlWlCqLgy0_91KhWEVmdnIfcppZaWR_KThFAtcD8JMCOYG_Yf1TrevFkG5Y9WOpfx9HV6MFamlRvchElWP46TiH-DCCf_bnto4-HOGTZpDN5cSCRI2NHZahNbhgPu4oMDM2My4MK3kQc7y_7N8ShOIAqDe0j_irs'}, {'domain': '.google.com', 'expiry': 1683189877, 'httpOnly': True, 'name': 'AEC', 'path': '/', 'sameSite': 'Lax', 'secure': True, 'value': 'AakniGPzQVs6uZH9iaixodFHhyrFSOk2Ji7p3ATkJSmOOlngCml58E6Khg'}, {'domain': '.google.com', 'expiry': 1670229880, 'httpOnly': False, 'name': '1P_JAR', 'path': '/', 'sameSite': 'None', 'secure': True, 'value': '2022-11-05-08'}]
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn