How to Reverse Order Using Slicing in Python
- Understanding Slicing in Python
- Reversing a List with Slicing
- Reversing a String with Slicing
- Reversing a Tuple with Slicing
- Conclusion
- FAQ
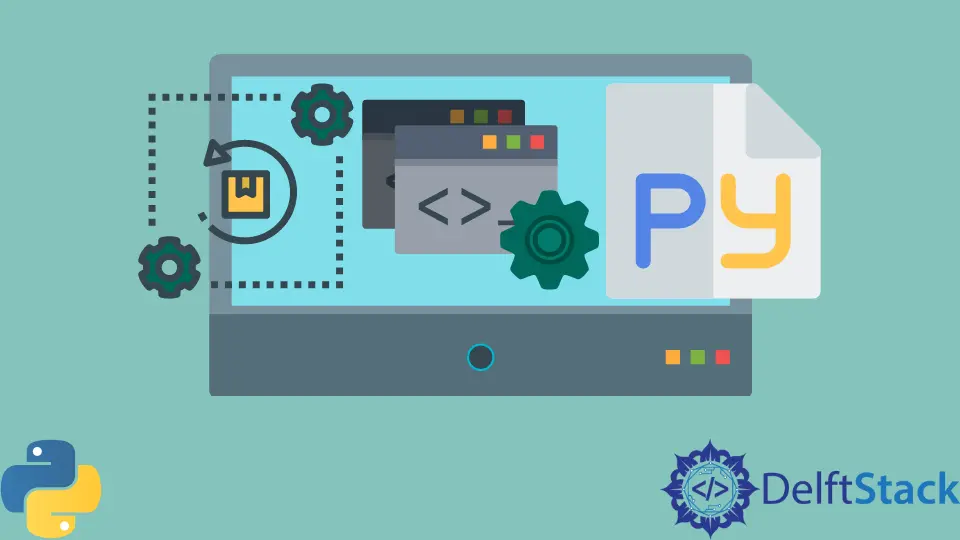
In the world of programming, there are various techniques to manipulate data efficiently. One such technique in Python is slicing, which allows you to access parts of sequences like lists, strings, and tuples. A particularly interesting application of slicing is reversing the order of these sequences. Whether you need to reverse a list of items or a string of text, Python’s slicing feature makes it simple and elegant.
This tutorial will walk you through the different methods of reversing order using slicing in Python, providing clear examples and explanations along the way. By the end of this article, you’ll have a solid understanding of how to reverse sequences effectively in your Python projects.
Understanding Slicing in Python
Before diving into reversing sequences, it’s essential to grasp the basics of slicing in Python. Slicing allows you to extract a portion of a sequence by specifying a start and end index, along with an optional step. The syntax for slicing is:
sequence[start:end:step]
- start: The starting index of the slice (inclusive).
- end: The ending index of the slice (exclusive).
- step: The interval between each index in the slice.
If you omit the start and end, Python defaults to the entire sequence. The step can also be negative, which is particularly useful for reversing sequences.
Reversing a List with Slicing
One of the most common use cases for reversing is with lists. Python makes this incredibly easy with its slicing feature. Here’s how you can reverse a list:
my_list = [1, 2, 3, 4, 5]
reversed_list = my_list[::-1]
Output:
[5, 4, 3, 2, 1]
In this example, we create a list called my_list
containing integers from 1 to 5. By using the slicing syntax [::-1]
, we effectively tell Python to start from the end of the list and move backward to the beginning. The -1
indicates that we want to step backwards through the list. The result is a new list, reversed_list
, that contains the elements in reverse order.
Reversing a list using slicing is not only concise but also efficient. It creates a new list rather than modifying the original one, which is a crucial aspect of functional programming. This method preserves the original list, allowing you to use it later if needed.
Reversing a String with Slicing
Strings in Python are immutable, meaning they cannot be changed after they are created. However, you can create a reversed version of a string using the same slicing technique. Here’s an example:
my_string = "Hello, World!"
reversed_string = my_string[::-1]
Output:
!dlroW ,olleH
In this code snippet, we have a string called my_string
. By applying the slicing method [::-1]
, we generate a new string reversed_string
that contains the characters of my_string
in reverse order. Just like with lists, this approach is straightforward and efficient, allowing you to easily reverse any string in Python.
Reversing strings can be particularly useful in various applications, such as checking for palindromes or simply formatting text in a specific way. The ability to reverse strings with slicing showcases Python’s flexibility and ease of use.
Reversing a Tuple with Slicing
Tuples are another common data structure in Python, similar to lists but immutable. You can also reverse a tuple using slicing. Here’s how you can do it:
my_tuple = (10, 20, 30, 40, 50)
reversed_tuple = my_tuple[::-1]
Output:
(50, 40, 30, 20, 10)
In this example, we define a tuple called my_tuple
containing integers. By applying the slicing method [::-1]
, we create a new tuple reversed_tuple
that contains the elements in reverse order. The immutability of tuples means that the original my_tuple
remains unchanged, while the new reversed_tuple
holds the reversed elements.
Reversing tuples can be useful in scenarios where you need to maintain the integrity of the original data while still requiring a reversed version for processing. This method is just as efficient and straightforward as reversing lists and strings.
Conclusion
Reversing sequences using slicing in Python is a powerful and straightforward technique that can enhance your programming skills. Whether you’re working with lists, strings, or tuples, Python’s slicing feature allows you to reverse the order of elements with minimal code and maximum efficiency. By mastering this skill, you’ll be better equipped to handle various data manipulation tasks in your Python projects. Remember, the key takeaway is that slicing provides a clean and effective way to achieve your goals without altering the original data structures.
FAQ
-
What is slicing in Python?
Slicing in Python is a method used to extract portions of sequences like lists, strings, and tuples by specifying start and end indices along with an optional step. -
Can I reverse a list without using slicing?
Yes, you can reverse a list using thereverse()
method or thereversed()
function, but slicing is often more concise.
-
Is reversing a string using slicing efficient?
Yes, reversing a string with slicing is efficient and creates a new string without modifying the original. -
Can I reverse a list in place?
Yes, you can reverse a list in place using thereverse()
method, which modifies the original list instead of creating a new one. -
Are tuples mutable in Python?
No, tuples are immutable, meaning once they are created, their elements cannot be changed. However, you can create a reversed version using slicing.