How to Remove Whitespace From a String in Python
- Remove Whitespace at the Beginning of a String in Python
- Remove Whitespace at the End of a String in Python
- Remove Whitespaces at Both the Beginning and the End of a String in Python
- Remove All Whitespaces From a String in Python
- Only Remove the Duplicated Whitespaces of a String in Python
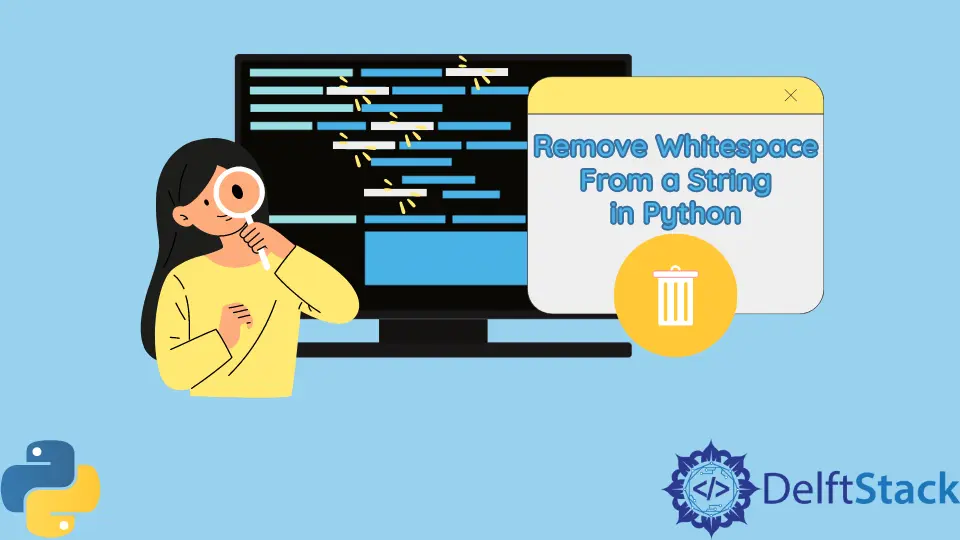
This article shows you below how to remove the whitespaces from a string in Python. It could be mainly categorized into two different approaches; one is Python str
methods, like str.split()
and str.replace()
; the other is Python regular expression method.
We will use the string " Demo Example "
as the string to be processed in the following examples.
Remove Whitespace at the Beginning of a String in Python
str.lstrip()
Method to Strip Whitespace at the Beginning
>>> demo = " Demo Example "
>>> demo.lstrip()
"Demo Example "
Here, str.lstrip()
method removes the leading characters specified in the method argument. If there is no argument given, it simply removes the leading whitespaces from the Python string.
Python Regex Method - re.sub
to Remove Whitespace in Python String
>>> import re
>>> demo = " Demo Example "
>>> re.sub(r"^\s+", "", demo)
"Demo Example "
^
forces regex to only find the matching string at its beginning, and \s
means to match all different kinds of whitespaces like whitespace, tab, return, etc. or in other words, it is equal to the collection of these special characters [ \t\n\r\f\v]
. +
indicates it should match whitespaces as many as possible.
You could refer to this Python regular expression tutorial to learn more about regex.
Remove Whitespace at the End of a String in Python
str.rstrip()
Method to Strip Python String Whitespaces
In contrast to str.lstrip()
that strips the characters at the beginning of the string, str.rstrip()
strips the characters at the end.
>>> demo = " Demo Example "
>>> demo.lstrip()
" Demo Example"
Python Regex Method - re.sub
to Trim Python String Whitespaces
Similarly, you should use an expression that matches the whitespaces at the end of the string.
>>> import re
>>> demo = " Demo Example "
>>> re.sub(r"\s+$", "", demo)
" Demo Example"
Remove Whitespaces at Both the Beginning and the End of a String in Python
str.strip()
Method to Remove Whitespaces From Python String
str.strip()
is the combination of str.lstrip()
and str.rstrip()
to remove whitespaces at the beginning and the end of the string.
>>> demo = " Demo Example "
>>> demo.strip()
"Demo Example"
Python Regex sub()
Method
>>> import re
>>> demo = " Demo Example "
>>> re.sub(r"^\s+|\s+$", "", demo)
"Demo Example"
Remove All Whitespaces From a String in Python
Python String Replace Method str.replace()
It is not necessary to check the position of the white space. Therefore, you could use str.replace()
method to replace all the whitespaces with the empty string.
>>> demo = " Demo Example "
>>> demo.replace(" ", "")
'DemoExample'
Python String Regex Replace sub()
Method
The regex expression could only be \s+
to match the whitespaces.
>>> import re
>>> demo = " Demo Example "
>>> re.sub(r"\s+", "", demo)
"DemoExample"
Only Remove the Duplicated Whitespaces of a String in Python
Python String Split Method str.split()
>>> demo = " Demo Example "
>>> " ".join(demo.split())
'Demo Example'
str.split()
returns a list of the sub-string in the string, using whitespaces as the delimiter string.
Python Regex Split Method re.split()
>>> import re
>>> demo = " Demo Example "
>>> " ".join(re.split(r"\s+", demo)
" Demo Example "
re.split()
and str.split()
are different where re.split()
will have an empty string at the beginning or the end of the list if the string has whitespaces in these positions, but str.split()
doesn’t include any empty string in its result.Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook