How to Make Random IP Address Generator in Python
-
Use the
Faker
Library to Generate a Random IP Address as a String in Python -
Use the
random
Module to Generate a Random IP Address as a String in Python -
Use the
ipaddress
Module to Generate a Random IP Address as a String in Python -
Use the
socket
Module to Generate a Random IP Address as a String in Python - Conclusion
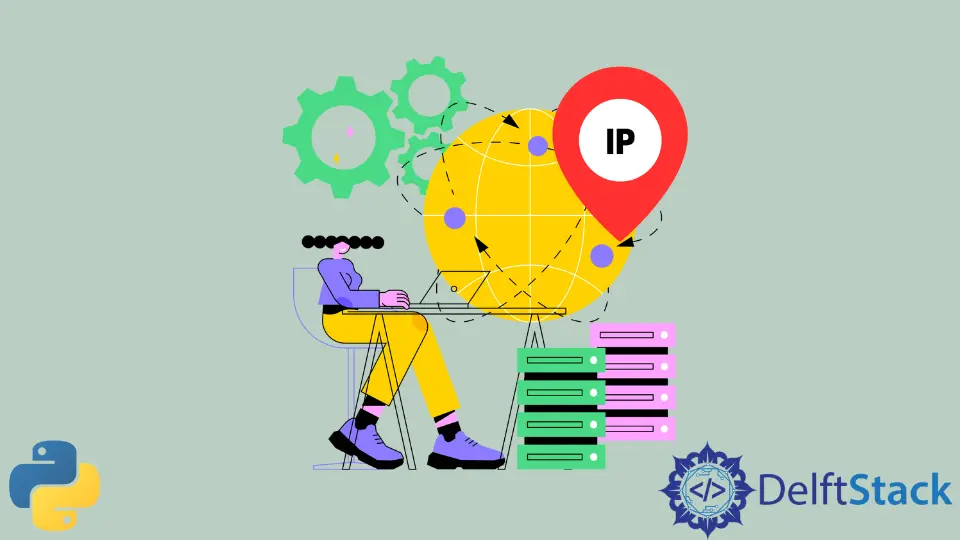
Generating random IP addresses in string format is a common requirement in various programming tasks, such as network testing, simulation, or populating databases with dummy data.
In this article, we will explore different methods to achieve this using Python. We’ll cover four methods: using the Faker
library, the random
module, the ipaddress
module, and the socket
module for IPv4 and IPv6 addresses.
Use the Faker
Library to Generate a Random IP Address as a String in Python
Faker
is a Python library that allows you to generate a wide range of fake data, such as names, addresses, email addresses, and much more. It’s useful for testing purposes, populating databases with sample data, and creating realistic simulations.
Before we can start generating fake IP addresses, we need to install the Faker
library. To do this, you can use pip
, Python’s package installer:
pip install faker
After installing this library, we can now start generating random IP addresses. To do this, we’ll create a Python script that utilizes the Faker
library to produce an IP address as a string.
Here’s a step-by-step guide to achieve this:
-
Import the
Faker
library. -
Create an instance of the
Faker
class. -
Use the
ipv4()
oripv6()
method to generate a random IPv4 or IPv6 address, respectively.
Once the Faker
library is installed, we can import it into our Python script and use its functionality to generate random IP addresses.
Use the ipv4()
Method to Generate Random IPv4 Address
The ipv4()
method generates a random IPv4 address in the format xxx.xxx.xxx.xxx
, where each xxx
is a number between 0 and 255. It ensures that the generated IP addresses are syntactically correct and follow the IPv4 address format.
Here’s how you can use the Faker
library to generate random IPv4 addresses in Python:
from faker import Faker
fake = Faker()
def generate_random_ip():
return fake.ipv4()
random_ip = generate_random_ip()
print("Random IP Address: ", random_ip)
Running the above code will produce output similar to the following:
Random IP Address: 193.123.56.78
In the above code, we imported the Faker
class from the faker
module. Then, we created an instance of the Faker
class, which allows us to use its various methods to generate fake data.
Following this, we defined a function generate_random_ip()
that generates a random IP address using the ipv4()
method provided by Faker
. We then called the generate_random_ip()
function to generate a random IP address and store it in the variable random_ip
.
Finally, we printed the randomly generated IP address. Note that each time you run the script, a different random IP address will be generated.
If you need more control over the generated IP addresses, you can also use the Faker
library’s localization and formatting features.
For example, you can specify a specific network range or format:
from faker import Faker
fake = Faker()
def generate_random_ip():
return fake.ipv4(network=True, address_class="b")
custom_ip = generate_random_ip()
print("Custom IP Address: ", custom_ip)
In this example, we’ve generated a random IP address within a specific Class B network range. You can customize the IP address by specifying the network range and class according to your requirements.
Use the ipv6()
Method to Generate Random IPv6 Address
We can use the Faker
library to generate random IPv6 addresses as well. This library provides a method called ipv6()
to generate random IPv6 addresses.
Here’s how we can modify the previous example to generate a random IPv6 address:
from faker import Faker
fake = Faker()
def generate_random_ipv6():
return fake.ipv6()
random_ipv6 = generate_random_ipv6()
print("Random IPv6 Address: ", random_ipv6)
Output:
Random IPv6 Address: f0d7:7e1e:7a39:32f1:c4aa:1e80:2287:1311
In this updated code, we defined a new function, generate_random_ipv6()
, that uses the ipv6()
method from Faker
to generate a random IPv6 address. We then called this function to generate a random IPv6 address and printed it.
Use the random
Module to Generate a Random IP Address as a String in Python
The random
module is a built-in Python module, so there’s no need to install it separately. To begin using the random
module, you simply need to import it.
import random
The random
module provides various functions for generating random values, and we will leverage these functions to create random IP addresses.
Recall that IP addresses consist of four numerical values separated by periods (e.g., 192.168.1.1
). Each part, known as an octet, ranges from 0 to 255.
To generate a random IP address, we’ll generate random numbers for each octet.
Here’s a step-by-step guide on how to generate a random IP address as a string:
-
Create a list to store the four octets of the IP address.
-
Use a loop to generate a random number between 0 and 255 for each octet.
-
Convert each octet to a string.
-
Join the four octets using periods to form the IP address string.
Here’s the Python code to achieve this:
import random
def generate_random_ip():
octets = []
for _ in range(4):
octet = str(random.randint(0, 255))
octets.append(octet)
ip_address = ".".join(octets)
return ip_address
random_ip = generate_random_ip()
print("Random IP Address:", random_ip)
Output:
Random IP Address: 194.211.219.245
This code defines a function called generate_random_ip()
that creates a random IP address string. It initiates an empty list named octets
to store the four octets of the IP address.
Through a loop iterating four times, it generates random octets within the valid range of 0 to 255 using the random.randint(0, 255)
function from the random
module. Then, each octet is converted to a string and added to the octets
list.
The octets are then joined using periods, forming a complete IP address string. The function returns this random IP address string.
Finally, upon calling the function, the script prints the message Random IP Address:
followed by the generated IP address string.
If you want to generate random IP addresses within a specific range, you can modify the code accordingly.
For example, if you want to generate IP addresses only in the private IP address range (e.g., 192.168.x.x
), you can restrict the range of the first octet:
import random
def generate_random_private_ip():
first_octet = random.randint(
192, 223
) # Private IP addresses range from 192.0.0.0 to 223.255.255.255
octets = [str(first_octet)]
for _ in range(3):
octet = str(random.randint(0, 255))
octets.append(octet)
ip_address = ".".join(octets)
return ip_address
random_private_ip = generate_random_private_ip()
print("Random Private IP Address:", random_private_ip)
Output:
Random Private IP Address: 201.112.99.129
This code generates IP addresses within the private address space, ensuring that the first octet falls within the range 192 to 223.
Use the ipaddress
Module to Generate a Random IP Address as a String in Python
The ipaddress
module in Python provides classes to work with IP addresses and networks, making it easy to manipulate and represent IP addresses in your Python programs. It allows you to create, manipulate, and perform operations on IPv4 and IPv6 addresses and networks.
To generate a random IP address as a string, we’ll follow these steps:
-
Import the
ipaddress
module. -
Use the
ip_address
function to create a random IP address. -
Convert the IP address object to a string using the
str()
function.
Let’s go through the code step by step:
import ipaddress
import random
def generate_random_ip():
random_ip = ipaddress.IPv4Address(random.randint(0, 2 ** 32 - 1))
return str(random_ip)
if __name__ == "__main__":
random_ip_address = generate_random_ip()
print("Random IP address:", random_ip_address)
Output:
Random IP address: 104.186.94.215
In this code snippet, we first imported the necessary modules: ipaddress
for working with IP addresses and random
to generate a random number. We then defined a function generate_random_ip()
that generates a random IPv4 address.
Inside the function, we used random.randint()
to generate a random 32-bit integer and then created an IPv4Address
object using the ip_address()
function from the ipaddress
module.
Finally, we converted the IP address object to a string using str()
and returned the random IP address.
In the main block, we called the generate_random_ip()
function and printed the resulting random IP address. You’ll see the output displaying a random IP address each time you run the script.
Now, generating a random IPv6 address follows a similar approach to generating a random IPv4 address. Here’s how you can modify the code to generate a random IPv6 address as a string:
import ipaddress
import random
def generate_random_ipv6():
random_ipv6 = ipaddress.IPv6Address(random.randint(0, 2 ** 128 - 1))
return str(random_ipv6)
if __name__ == "__main__":
random_ipv6_address = generate_random_ipv6()
print("Random IPv6 address:", random_ipv6_address)
Output:
Random IPv6 address: 54e9:df42:fd1a:6380:1312:e866:2f7a:4a03
In this modified code, we used the IPv6Address
class from the ipaddress
module to generate a random IPv6 address. The random.randint()
function generates a random 128-bit integer, which is then used to create the IPv6 address.
Finally, the resulting IPv6 address is converted to a string using the str()
function.
This code will display a random IPv6 address each time you run the script.
Use the socket
Module to Generate a Random IP Address as a String in Python
Python’s socket
module provides low-level access to networking functionalities. We can utilize this module to generate random IP addresses.
Here’s a step-by-step approach:
-
First, we need to import the
socket
module to access its functionalities. -
We’ll generate four random numbers (each representing an octet) using the
random.randint()
function, with each number ranging from 0 to 255, inclusive. -
Concatenate the four random octets using periods to create a valid IP address in dotted-decimal notation.
-
Display the generated IP address or use it for your specific application or simulation.
Let’s implement this in Python:
import socket
import random
def generate_random_ip():
ip_octets = [str(random.randint(0, 255)) for _ in range(4)]
# Concatenate the octets to form the IP address
ip_address = ".".join(ip_octets)
return ip_address
random_ip = generate_random_ip()
print("Random IP Address:", random_ip)
Output:
Random IP Address: 101.131.185.15
In this example, we started by importing the socket
and random
modules. Then, we defined a function, generate_random_ip()
, that generates four random octets for the IP address using a list comprehension.
The random.randint()
function is used to generate random values for each octet. The octets are then concatenated using the join()
method to form a valid IP address.
Let’s have another example code on generating an IPv4 address using the random
, socket
, and struct
modules in Python.
import random
import socket
import struct
ip = socket.inet_ntoa(struct.pack(">I", random.randrange(1, 0xFFFFFFFF)))
print(ip)
Output:
101.131.185.15
First, the random.randrange(1, 0xffffffff)
function generates a random 32-bit unsigned integer between 1 and the maximum value of a 32-bit unsigned integer (0xffffffff
in hexadecimal). This value is then packed into a binary format using struct.pack('>I', random.randrange(1, 0xffffffff))
, specifying big-endian byte order ('>I'
).
The socket.inet_ntoa()
function is then employed to convert this packed binary representation into a string format representing an IPv4 address in dotted-decimal notation.
Finally, the resulting random IP address is printed to the console using print(ip)
.
Conclusion
Generating random IP addresses in Python is made easy using the Faker
library, the random
module, the ipaddress
module, or the socket
module. Whether you need IPv4 or IPv6 addresses, these methods allow you to efficiently generate random IP addresses for your applications.
Use these techniques for various purposes, including testing, simulation, and populating databases with dummy data. Select the method that suits your needs and integrate it into your projects to generate the desired IP addresses.