Use and in Python
- ASCII Line Feed in Python
- ASCII Carriage Return in Python
- End of Line Sequences in Python
-
Difference Between
\n
and\r
in Screen Output in Python -
Similarity and Difference Between
\n
and\r
in Text Files in Python - Conclusion
- References
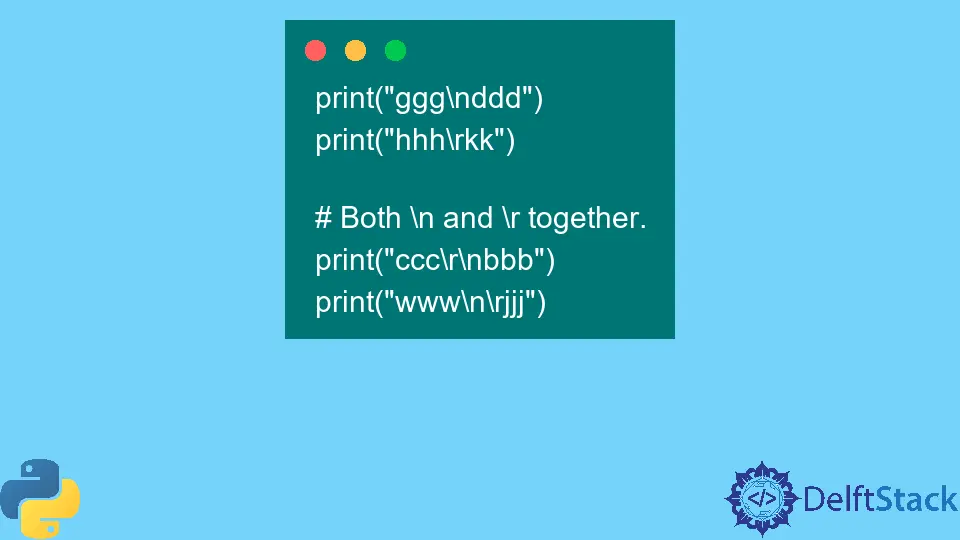
This article will explore the difference between Python’s escape sequences \n
and \r
.
ASCII Line Feed in Python
The escape sequence \n
represents the ASCII line feed (LF).
We can use the escape sequence \n
to create a new line inside a string. The backslash tells Python to treat the following n
as a command, creating a new line.
The cursor gets placed at the beginning of the next line.
ASCII Carriage Return in Python
The escape sequence \r
represents the ASCII carriage return (CR).
\r
behaves differently depending on whether the output is sent to the screen or a text file.
Further, the order matters when \r
is combined with \n
and the output is sent to a text file.
End of Line Sequences in Python
In a text file, Linux uses LF to signal the end of the line. Windows uses CR LF for this purpose.
Older versions of Macintosh used CR.
We need to keep this in mind if we handle Windows text files.
Difference Between \n
and \r
in Screen Output in Python
The following code shows the difference between \n
and \r
when the interpreter output is displayed.
Example Code:
print("ggg\nddd")
print("hhh\rkk")
# Both \n and \r together.
print("ccc\r\nbbb")
print("www\n\rjjj")
Output:
ggg
ddd
kkh
ccc
bbb
www
jjj
The first statement printed the letters following \n
on the second line because the escape sequence placed the cursor at the beginning of a new line.
The \r
escape sequence in the second statement placed the cursor at the beginning of the same line, and the letters kk
replaced as many characters as what was already there on that line. \r
does not advance the cursor to the next line.
We also find that both \n\r
and \r\n
behave similarly in this case.
Similarity and Difference Between \n
and \r
in Text Files in Python
If we write the interpreter’s output to a text file, both \n
and \r
behave similarly when alone but differently when together.
Both create a new line of text, unlike what happens when displayed on the screen when used alone.
When used in combination, \r\n
creates only one new line of text, like either \n
or \r
taken alone.
However, \n\r
creates two lines of text. When \n
is followed by \r
, the first escape sequence, \n
creates one line, and the second, \r
, creates another line.
We have to create a text file and use its path to run the following example code.
Example Code:
# Create variables.
new_l = "\n"
sta1 = "YYY\rWWW"
sta2 = "ZZZ\nUUU"
sta3 = "SSS\r\nQQQ"
sta4 = "RRR\n\rMMM"
# Open a file in append mode.
fl = open("/path/to/text/filename.txt", "a")
# Write the variables to the file.
fl.writelines([sta1, new_l, sta2, new_l, sta3, new_l, sta4])
# Close the file.
fl.close()
Output:
YYY
WWW
ZZZ
UUU
SSS
QQQ
RRR
MMM
Conclusion
We should use \n
to create a new line if writing to screen. If we use \r
, the text that follows it overtypes the content of the current line.
When writing to a text file, we may use either \n
or the \r\n
sequence. We also need to bear in mind the end of line sequences in different operating systems.
References
See sections 2.1 and 2.4 in the Python Language Reference.