Python Verbose
- Understanding Verbosity
- Enabling Verbose Mode
- Verbose in Python
- Practical Usage of Python Verbose
- Conclusion
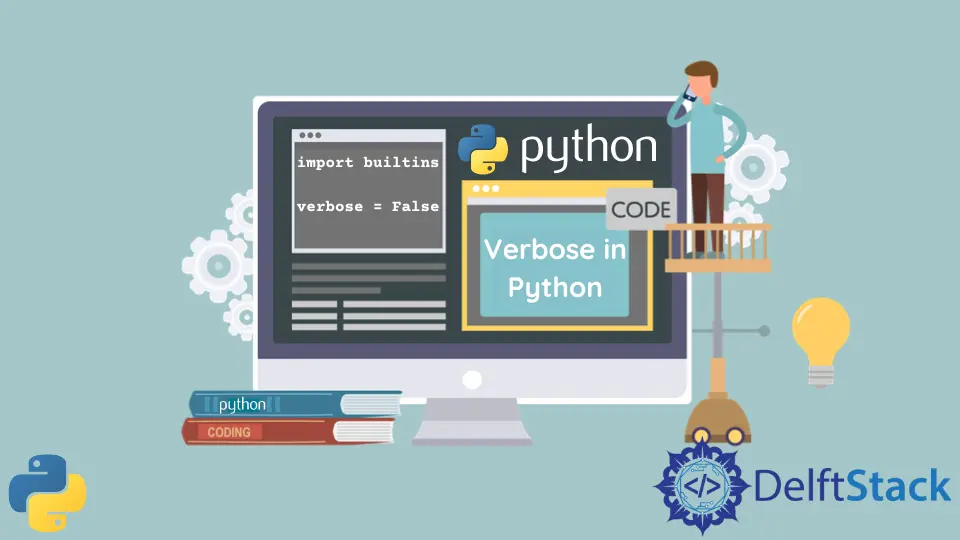
In the world of programming, debugging and understanding code can be challenging tasks. Python, being a versatile and developer-friendly language, offers a powerful tool known as verbose
to aid in the diagnostic process.
This article delves into the details of the verbose mode in Python, exploring its purpose, syntax, and practical applications in various programming scenarios.
Understanding Verbosity
Verbosity, in the context of programming, refers to the level of detail or information provided by a program during its execution. The more verbose a program is, the more information it outputs, aiding developers in understanding the flow of the code and diagnosing potential issues.
Python’s verbose mode is a feature that enhances the visibility of code execution by providing additional information at runtime.
Enabling Verbose Mode
In Python, the verbose mode can be activated using the -v
or --verbose
command-line option. When this option is set, the interpreter produces more detailed output during execution.
This can be particularly useful for troubleshooting, optimizing code, and gaining insights into the inner workings of a program.
Syntax:
python -v script.py
Or
python --verbose script.py
Verbose in Python
Verbose mode is a computing feature and a generic concept found in many computer operating systems and programming languages. In computing, it gives users more information about what the computer is doing, what drivers and software it is loading at startup, or in programming, it would produce detailed output for diagnostic purposes, making a program easier to debug.
In Python, we use verbose as a Boolean flag and can use it instead of using the if
statement. For instance, if we were to define our custom print function, we can do it using simply the if
statement or we can also do it by declaring the verbose as a Boolean variable.
We have used verbose as a generic concept and made our custom print function. Now, whenever we call it, it’ll work as we have defined it.
First, we need to import built-ins. After that, we can make our custom functions.
Take a look at the following code:
import builtins
# create a boolean variable for verbose check and set it to false by default
verbose = False
# Now, to implement verbose in Python, you have to write your custom print function
if verbose:
def print(*args):
return builtins.print(*args, sep="\n")
This code introduces a custom print
function with verbosity control. It defines a Boolean variable verbose
and, if set to True
, overrides the default print
function to print each argument on a new line.
The code provides a way to enhance readability in verbose mode by customizing the printing behavior.
Here’s an example of how you might use this code:
verbose = True # Set verbose mode to True
print("This", "is", "verbose")
If verbose
is True
, this will print each word on a new line. If verbose
is False
, it will use the regular print
behavior.
Practical Usage of Python Verbose
Module Import Debugging:
Verbose mode is highly beneficial for understanding the module import process. When a script is executed with the verbose option, Python displays detailed information about which modules are being imported and where they are located.
python -v my_script.py
Optimizing Code Execution:
For performance optimization, verbose mode provides insights into the time taken by various modules and functions during execution. This information can be crucial for identifying bottlenecks and optimizing critical sections of code.
python -v performance_script.py
Dependency Resolution:
In larger projects with numerous dependencies, verbose mode assists in understanding the order and hierarchy of module imports. This is valuable when dealing with complex codebases with intricate dependencies.
python -v complex_project.py
Troubleshooting Import Errors:
When encountering import errors or issues related to module dependencies, verbose mode helps diagnose the problem by providing a detailed trace of the import process. This facilitates the swift identification of missing or incompatible dependencies.
python -v troubleshooting_script.py
Python Interpreter Internals Exploration:
Verbose mode is a powerful tool for those interested in exploring the internal workings of the Python interpreter. It reveals the sequence of operations performed by the interpreter, shedding light on the underlying mechanisms of code execution.
python -v internals_script.py
Conclusion
In conclusion, the verbose mode in Python is an invaluable tool for developers seeking a deeper understanding of their code’s execution. Whether it’s debugging module imports, optimizing performance, troubleshooting errors, or exploring the internals of the Python interpreter, the verbose mode provides a detailed and insightful view into the runtime behavior of Python programs.
By leveraging this feature, developers can enhance their debugging capabilities and streamline the development and optimization processes.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn