How to Use Urlencode in Python
-
URL Encode a Dictionary Using
urlencode()
in Python -
URL Encode a Multi-Valued Dictionary Using
urlencode()
in Python -
Use the
urlencode()
Function in Python 3 -
Use the
urllib.parse.quote_plus()
Function to Quote and Encode URL in Python 3 -
Use the
urllib.parse.quote()
Function to Quote and Encode URL in Python 3 - Conclusion
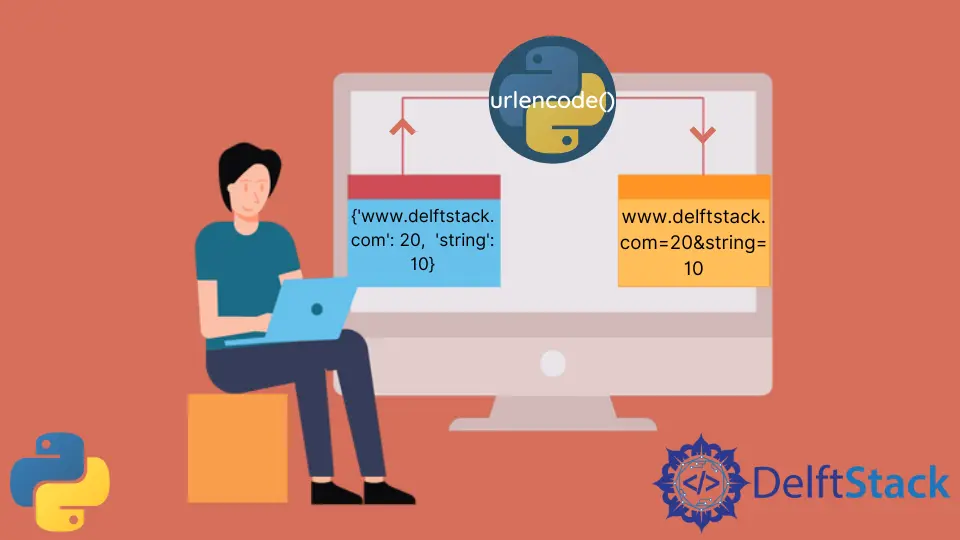
URL stands for uniform resource locator. URL is simply the address of a resource on the web through the URL the browser requests for the webpage to load.
URL encoding means converting characters to a special format using limited US-ASCII
, which is universally accepted by all browsers and web servers.
If a URL contains characters other than the characters defined in the ASCII set, these characters will be converted into a valid ASCII format. It is also known as percent encoding because we see percent symbols frequently in URLs.
Whenever we call a web API or submit an HTTP form data, we use an encoded URL to encode the query string. In Python, we can URL encode a query string using the urlib.parse
module, which further contains a function urlencode()
for encoding the query string in the URL, and the query string is simply a string of key-value pairs.
URL Encode a Dictionary Using urlencode()
in Python
The urlencode()
function from the urllib.parse
module is employed to convert a Python dictionary into a URL-encoded string.
If we want to URL encode a string using the urlencode()
function, we cannot do it directly because it does not accept a string as an argument. We can pass the argument in the form of a dictionary.
The dictionary
is a data type in Python that stores the data in a key-value pair.
For example, we have a query string like this:
queryString = "name=" + "Jhon" + "&" + "age=" + "18"
First, we have to write it in a dictionary format like this:
queryString = {"name": "Jhon", "age": "18"}
In the following code, we have to import the urlib
library, and we will pass our query string to the urlencode()
function of the parse
module of the urlib
library.
Example Code:
import urllib
from urllib.parse import urlencode
queryString = {"name": "Jhon", "age": "18"}
print(urllib.parse.urlencode(queryString))
Output:
name=Jhon&age=18
As we can see, the output is name=Jhon&age=18
. This result represents the URL-encoded form of the input query string, where spaces are replaced by +
symbols, and key-value pairs are connected using &
symbols.
The equals symbol (=
) is used to separate each key from its corresponding value in the URL-encoded string.
URL Encode a Multi-Valued Dictionary Using urlencode()
in Python
Now let’s say our query string, which is in the form of a dictionary, contains multi-valued data like an attribute named colors with values blue
, pink
, and green
, so it’s a multi-valued attribute. We can also URL encode such dictionaries in Python.
The urlencode()
function takes an optional argument doseq
. If we set its value to True
, we can avoid special characters in the output.
Example Code:
import urllib
from urllib.parse import urlencode
queryString = {"jeans": "Bell Bottom", "colors": ["blue", "pink", "green"]}
print(urllib.parse.urlencode(queryString, doseq=True))
In this code, we create a dictionary named queryString
with keys "jeans"
and "colors"
, having corresponding values. Using urllib.parse.urlencode()
with doseq=True
, we encode the dictionary into a URL-encoded format.
Output:
jeans=Bell+Bottom&colors=blue&colors=pink&colors=green
The output, when printed, is jeans=Bell+Bottom&colors=blue&colors=pink&colors=green
, representing the encoded key-value pairs with proper handling of multi-valued parameters.
Use the urlencode()
Function in Python 3
We can encode different parameters using the urlencode()
function in Python. We can pass the query in the function as key-value pairs of a dictionary or as a list of tuples with two elements to perform encoding with this function.
The characters _
, -
, .
, ~
, letters, and digits are not quoted.
In Python 2, this was directly part of the urllib
module. The final result was a string.
Example Code:
import urllib
query = urllib.urlencode({"www.delftstack.com": 20, "string": 10})
print(query)
In this code, we use the urlencode()
function from the urllib
module in Python. We create a dictionary named query
with keys "www.delftstack.com"
and "string"
, each associated with numerical values.
By applying urllib.urlencode()
to this dictionary, we efficiently encode the key-value pairs into a URL-encoded string.
Output:
www.delftstack.com=20&string=10
The output is www.delftstack.com=20&string=10
, representing the encoded URL-ready format of the input dictionary. The above code works with Python 2.
In Python 3, the urllib
was split into different submodules. The urlencode()
function was made a part of the urllib.parse
module.
We can use this function by importing the method from this library.
Example Code:
import urllib
from urllib.parse import urlencode
query = urllib.parse.urlencode({"www.delftstack.com": 20, "string": 10})
print(query)
In this code, we employ the urlencode()
function from the urllib.parse
module in Python. We create a dictionary named query
with keys "www.delftstack.com"
and "string"
, each associated with numerical values.
By utilizing urllib.parse.urlencode()
, we efficiently encode the key-value pairs into a URL-ready format.
Output:
www.delftstack.com=20&string=10
The output is www.delftstack.com=20&string=10
, representing the URL-encoded string derived from the input dictionary.
The urlencode()
function uses the quote_plus()
method internally to perform encoding, and we can change this using the quote_via
parameters. The urlencode
function has two important parameters, encoding
and safe
, and we can specify the ASCII characters safe from the quoting with the safe
parameter.
The default value of this parameter is the \
character. The encoding
parameter accepts the encoding type to deal with non-ASCII characters.
Use the urllib.parse.quote_plus()
Function to Quote and Encode URL in Python 3
We can use the urllib.parse.quote_plus()
function to replace the special characters and spaces in a URL with +
signs in a string. By default, this function is used in the urlencode()
function.
Example Code:
import urllib
from urllib.parse import urlencode
query = urllib.parse.quote_plus("delftstack$urllib")
print(query)
In this code, we utilize the quote_plus()
function from the urllib.parse
module in Python. We create a string named query
containing the text "delftstack$urllib"
.
By applying urllib.parse.quote_plus()
, we effectively replace special characters and spaces in the string with '+'
signs.
Output:
delftstack%24urllib
The output is delftstack%24urllib
, demonstrating the URL-encoded format of the input string. We can access this function in Python 2 directly under the urllib
package.
Use the urllib.parse.quote()
Function to Quote and Encode URL in Python 3
The urllib.parse.quote()
function accepts a string and replaces the special characters with the %xx
. It also accepts the parameters mentioned in the previous functions.
Example Code:
import urllib
from urllib.parse import urlencode
query = urllib.parse.quote("delftstack$urllib")
print(query)
In this code, we employ the quote()
function from the urllib.parse
module in Python. We create a string named query
containing the text "delftstack$urllib"
.
By using urllib.parse.quote()
, we replace special characters in the string with the %xx
format.
Output:
delftstack%24urllib
The output is delftstack%24urllib
, illustrating the URL-encoded representation of the input string.
We can use this function internally with the urlencode()
function, bypassing the urllib.parse.quote
function as the value of the quote_via
parameter.
This function can also be directly accessed in the urllib
package in Python 2.
Conclusion
In conclusion, a Uniform Resource Locator (URL) serves as the address for web resources, facilitating communication between browsers and web servers.
URL encoding is crucial for handling special characters in URLs, ensuring compatibility across all browsers and web servers. When interacting with web APIs or submitting HTTP form data, we employ encoded URLs to encode query strings.
In Python, the urllib.parse
module, featuring the urlencode()
function, offers a convenient method for encoding query strings in URL format. This function accepts dictionaries as input, converting key-value pairs into the required encoded URL.
Additionally, the urllib.parse
module in Python 3 provides other functions like quote_plus()
and quote()
for specialized URL encoding purposes. Overall, these tools empower developers to handle URL encoding efficiently in Python, ensuring the smooth exchange of information in web applications.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn