How to Unset a Linux Environment Variable in Python
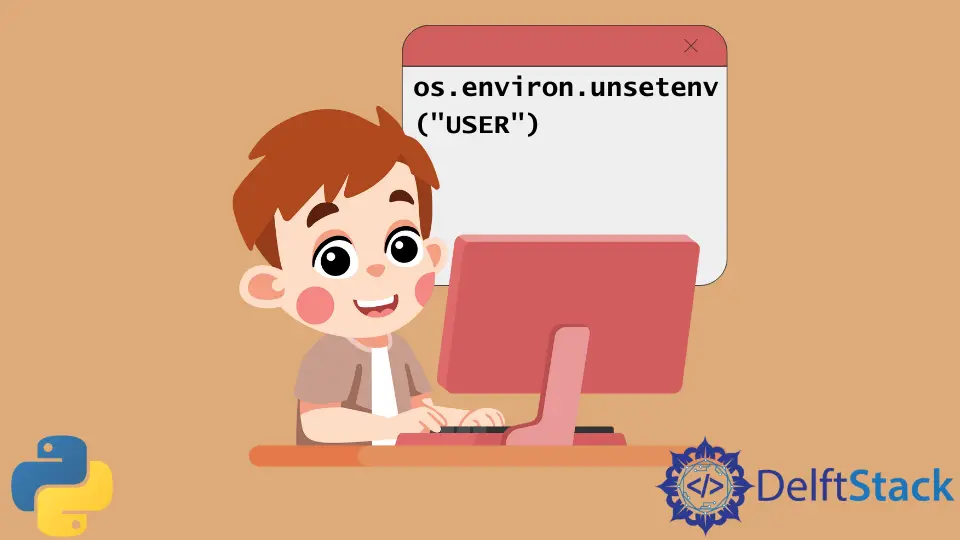
In this article, we will learn different ways to unset a Linux environment variable in Python. The most helpful method is to use the unset
command.
However, we can use several other ways to unset a Linux environment variable in Python. Read the article to explore more.
Unset a Linux Environment Variable in Python
Python works with different operating systems (OS). Linux is one of the operating systems on which Python can be used.
Even though we can use Linux and Python both, there are some distinctions between the two. One of the differences we need to consider is how environment variables are interpreted.
We can use the os.unsetenv()
method in Python if we want to unset an environment variable we previously set.
This function will remove the environment variable from the current process and accept the environment variable’s name as an argument. Plus, the function will delete the environment variable from the current process.
Thus, it is essential to remember that unsetting a shell environment variable using Python will not remove the value from the shell. If the environment variable is set in the shell, then Python’s child processes will still have access to it even if they were started after the Python process.
The unset
command should be used if you want to clear out an environment variable from the shell.
Ways to Unset a Linux Environment Variable in Python
Python provides many options for removing and unsetting a value from an environment variable on Linux in Python.
- Using the
unset
command is the most typical and common approach. With this command, the environment variable will be removed from the shell that is now active. - Python’s
export
command offers an additional method for clearing a Linux environment variable that was previously set. This command will remove the environment variable from the active shell and any child processes. - Lastly, another option is to take advantage of the
os.environ.pop
technique. After executing this method, the environment variable will be removed from theos.environ
dictionary.
The strategy that we choose is based on the requirements that we have.
- The
unset
command is what we would use if we want to clear the environment variable from the shell that we are now working in. - The
export
command is what we would use if we want to clear the environment variable from the currently active shell and any child processes. - We use the
os.environ.pop
function if all we want is to delete the environment variable from theos.environ
dictionary.
Use os.environ
to Delete or Unset One of the Environment Variables
When working with Python on Linux, we may need to delete or unset one of the environment variables.
For instance, if we have the PYTHONPATH
environment variable configured to point to a certain place and we now wish to delete that setting, we can use the code provided in the following example.
# import os library
import os
# Unset the path with the command
os.environ.pop("PYTHONPATH", None)
This action will remove the PYTHONPATH
environment variable from the os.environ
dictionary. Nothing will happen if the environment variable has not been set in this case.
Use del os.environ
to Unset One of the Environment Variables
We can also use the option of using the code presented below to clear an environment variable.
# import operating system
import os
# use this command to unset
del os.environ["PYTHONPATH"]
Output:
KeyError Traceback (most recent call last)
KeyError: 'PYTHONPATH'
This action will remove the PYTHONPATH
environment variable from the os.environ
dictionary. This will result in a KeyError
if the environment variable is not set.
Use os.environ.unsetenv
and os.environ
to Delete and Replace Variable
If we want to clear the value that was assigned to the variable USER
and replace it with the word nobody
, you will proceed as follows:
import os
os.environ.unsetenv("USER")
os.environ["USER"] = "nobody"
The code above clarifies the value assigned to the variable USER
and replaces it with the word nobody
.
Use os.environ.unsetenv
to Unset
We can leave out the second parameter if all we want to do is clear the variable’s value. For instance, the USER
variable would not be set if you did the following.
import os
os.environ.unsetenv("USER")
The code above clears the value of the variable. The USER
variable would not be set.
Use os.environ.get()
to Check if a Variable Is Set Before Unsetting
Checking to see if a variable has been set before unsetting it may also be accomplished with the help of the os.environ.get()
function. If you wish to unset a variable only if it’s presently set, you may utilize this to your advantage.
For instance, the following would only remove the value of the USER
variable if it was already defined as nobody
.
Example code:
import os
if os.environ.get("USER") == "nobody":
os.environ.unsetenv("USER")
As we already know, clearing a variable’s value does not always mean removing it from the environment. It accomplishes nothing more than setting the value to an empty string.
You can use the os.environ.pop()
function if you want to fully remove a variable from the environment. This function will remove the variable from the environment while returning the value it had before it was removed.
Example code:
import os
prev_value = os.environ.pop("USER", None)
print(prev_value) # prints "nobody"
Output:
nobody
This code will remove the USER
variable from the environment and return the value that was there before it was removed.
We hope you find this Python article helpful in understanding ways to unset a Linux environment variable in Python.
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn