How to Set Environment Variable in Python Script
- How Can We Use Environment Variables
- Setting Environment Variables in Python
-
Set Environment Variables With
os.environ
Method -
Set Environment Variables With
os.environ.setdefault
Method - Conclusion
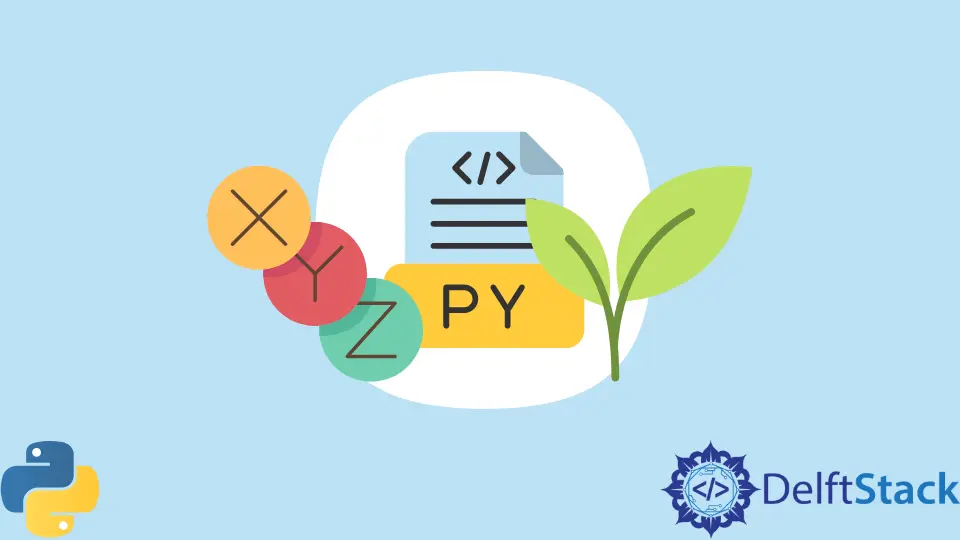
Environment variables are an in-depth way to interact with the processes of a system; it allows users to get even more detailed information about the properties of the system, the path, and the variables that already exist.
How Can We Use Environment Variables
As mentioned above, environment variables impel us from interacting with the system’s processes. We can use the environment variables to access all the variables and keys in the system.
To do that, let us create a new file, name it new.py
and type in these codes:
new.py
:
import os
print("The keys and values of all environment variables:")
for key in os.environ:
print(key, "=>", os.environ[key])
You will see all the variables and keys printed out in the terminal.
We can also view a specific variable. To do that, create a new Python file and type in these codes:
new.py
:
import os
print("The value of HOME is: ", os.environ["USERPROFILE"])
This will print out the system’s HOME
path.
We can also use the environment variables to locate the paths of all the programs we have installed in the system. This is beneficial for us to locate the exact file location of the installed programs.
To do this, we create another file, new.py
, and type in this snippet of code:
new.py
:
import os
print("Set Environment Variables: ", os.environ["PATH"])
You will see all file locations of the programs we have installed in the terminal.
Environment variables also provide a secure and seamless way to protect the token/access keys we use in an API. When we put our keys inside a .env
file, it hides our keys from the view of someone who views our codes and then it also helps us use the keys wherever we need to apply without the need to keep redefining them.
All that needs to be done is create a token and input it inside a .env
file.
Setting Environment Variables in Python
Aside from that, we can access all the paths and environment variables in a system; we can go further and set our variables using two methods, namely: the os.environ
and the os.environ.setdefault
methods.
Set Environment Variables With os.environ
Method
The os.environ
method can create a new variable and assign a value. To do that, we will create a new file, name it new.py
and input these codes:
new.py
:
import os
os.environ["USERNAME"] = "python"
print(os.environ["USERNAME"])
When we run this, we will see python
displayed in the terminal.
Additionally, we can change the value assigned to a variable using the os.environ
method. To do that, we will create a new file, name it new.py
and type in these codes:
new.py
:
import os
home = os.environ["USERNAME"]
print("USERNAME:", home)
This snippet will display the original value assigned to the variable, HP
, in our case. Now to assign a new value to the variable, we will create a new file, name it new2.py
and assign these codes:
new2.py
:
import os
os.environ["USERNAME"] = "USER"
print("USERNAME:", os.environ["USERNAME"])
Here, we have assigned a new value, USER
, to the variable. When we run the code, the terminal will display the newly assigned value, USER
.
Set Environment Variables With os.environ.setdefault
Method
The os.environ.setdefault
method, like the os.environ
method, can create a new variable and assign a value.
Create a new file, name it new.py
and type in these codes:
new.py
:
import os
if not os.environ.get("USERNAME"):
os.environ.setdefault("USERNAME", "1")
else:
os.environ["USERNAME"] = "1"
print(os.environ["USERNAME"])
We use the os.environ.get
method to get the variable, then we use the os.environ.setdefault
method to assign a new variable.
When we run this code, we will see the new value displayed in the terminal.
Conclusion
If you intend to learn the ins and outs of a computer system, utilizing the environment variables is one such way to do it.
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn