How to Count Syllables in Python
-
Use the
if
Statement in Python to Count Syllables - Use Dictionary and List Comprehension in Python to Count Syllables
-
Use Dictionary by
fromkeys()
Function in Python to Count Syllables -
Use the
str()
andset()
Functions in Python to Count Syllables
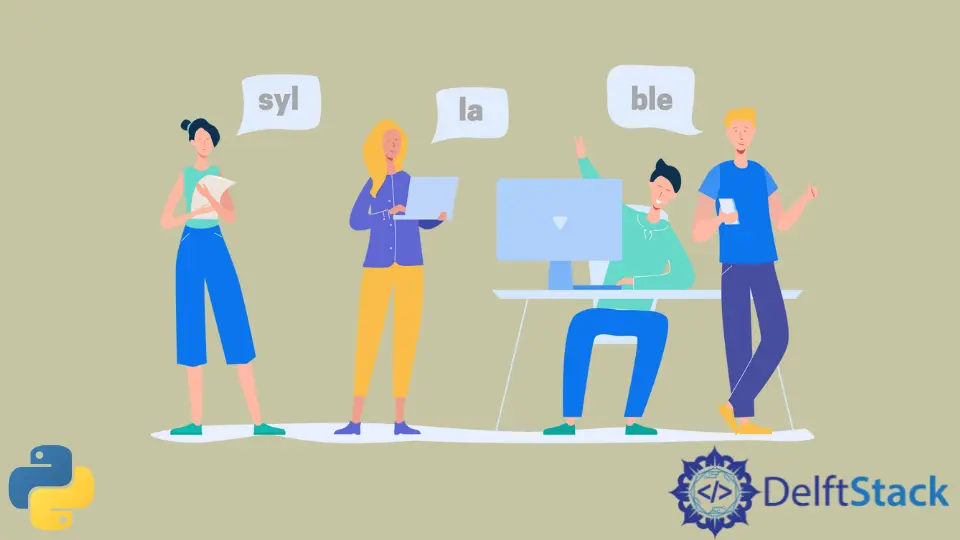
Counting words, phrases, letters, and some specific characters is a common task to do in programming. In all these tasks, counting syllables in a word or a sentence is also a very common thing to do by the user.
In this tutorial, we will see different methods by which we can count syllables in Python.
Use the if
Statement in Python to Count Syllables
In this method, we enter a string with the help of the input()
function, a built-in function in Python.
Example:
word = input("Enter the word:")
syllable_count = 0
for w in word:
if (
w == "a"
or w == "e"
or w == "i"
or w == "o"
or w == "u"
or w == "A"
or w == "E"
or w == "I"
or w == "O"
or w == "U"
):
syllable_count = syllable_count + 1
print("The number of syllables in the word is: ")
print(syllable_count)
Here, the user can enter any word to use the input()
function. The syllable count has been set to 0 initially, and all the syllables to be returned from the word have been mentioned using a for
loop. Note that all the syllables have been given in lower case as well as upper case.
Output:
Enter the word: Beautiful
The number of syllables in the word is: 5
Use Dictionary and List Comprehension in Python to Count Syllables
In list comprehension, a new list is made based on the elements in an existing list. After using list comprehension, the output comes in the form of a dictionary.
Dictionary in Python is a collection of items that are stored in no specific order. Each item in the dictionary has its own value. The value of the item can be known if the item name is known.
Example:
sentence = "Hello, Let us see how many syllables are there in this sentence"
sentence = sentence.casefold()
vowel_count = {s: sum([1 for letter in sentence if letter == x]) for s in "aeiou"}
print(vowel_count)
Note that the function casefold()
is used in the above code. The casefold()
function helps to convert a string or a set of strings to the lower case. So if there are some syllables in the given sentence in the upper case, this function turns these syllables into the lower case. Now there is no need to mention the syllables that are to be returned in the upper case and lower case.
Also, the sum()
method used in the above code calculates the total sum of the values of each item in the list.
Output:
{'a': 3, 'e': 11, 'i': 2, 'o': 2, 'u': 1}
You can see that the output returned is a dictionary that has different items as syllables with their specified value.
Use Dictionary by fromkeys()
Function in Python to Count Syllables
With the help of the fromkeys()
function, a dictionary can be made with different items and their specified value.
In this method also, we use the casefold()
function so that the given set of strings is converted in lower case, and we can take the input from the user itself. Also, even in this method, we initialize the syllable count with 0.
Example:
syllables = "aeiou"
word = input("Enter a word or a sentence: ")
word = word.casefold()
syllable_count = {}.fromkeys(syllables, 0)
for w in word:
if w in syllable_count:
syllable_count[w] += 1
print(syllable_count)
Output:
Enter a word or a sentence: Hello, Let Us See How Many Syllables Are There In This Sentence
{'a': 3, 'e': 11, 'i': 2, 'o': 2, 'u': 1}
Use the str()
and set()
Functions in Python to Count Syllables
The set()
function is used to return a set object in which all the items are in no particular order. This function also removes the items in the set which are repeated.
With the help of the str()
function, any value or an object can be converted into a string.
In this method, the syllables will be the argument of the set function that means a set of syllables is created.
Example:
def syllable_count(str):
count = 0
syllables = set("AEIOUaeiou")
for letter in str:
if letter in syllables:
count = count + 1
print("Total no. of syllables :", count)
str = "beautiful"
syllable_count(str)
Output:
Total no. of syllables : 5
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn