How to Create Python Script to Open a New Terminal and Run Commands in Linux
- Create Python Script That Opens a New Terminal and Run Commands in Linux
- Create Python Script to Check Python Version in Linux
- Python Script to Keep an Open Terminal Alive and Run Commands in It
-
Pass Commands to the New Terminal Using Python Submodule
subprocess()
in Linux - Conclusion
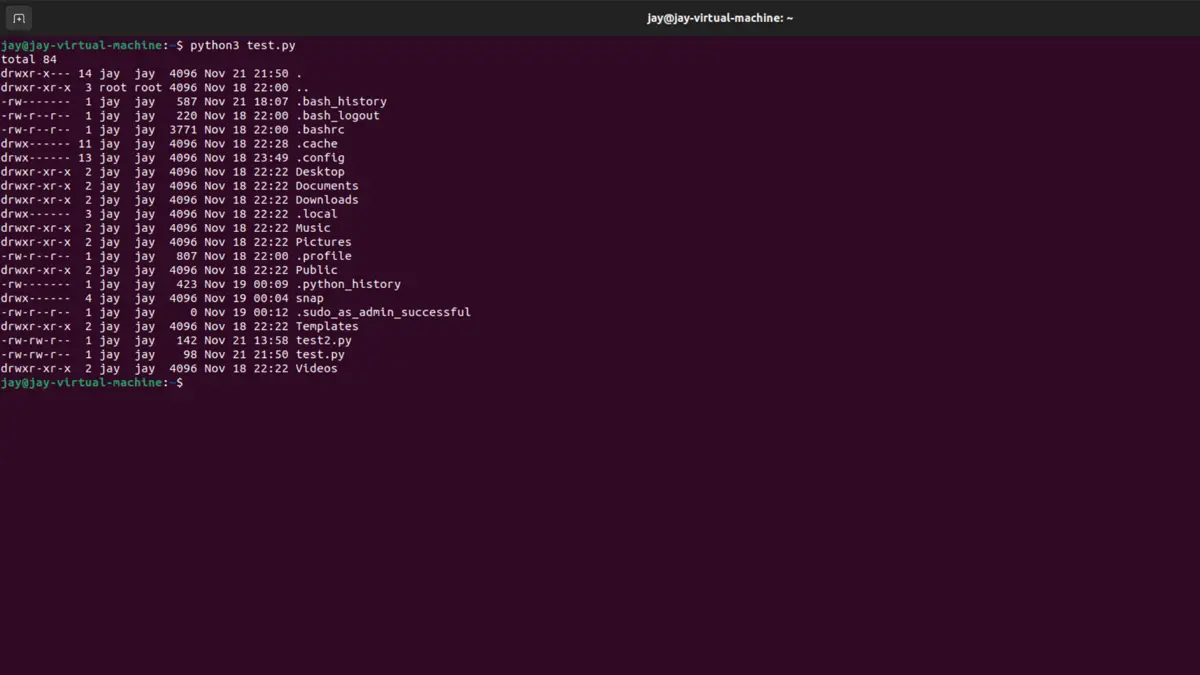
Linux operating system is known for its versatile terminal that programmers extensively use. But there are situations where we find ourselves writing the same set of syntax repeatedly, which can be boring and time-consuming.
Have you ever wondered if there’s a way to pre-write Linux commands in a file and then execute it at once in the terminal? Yes! You can.
You can write pre-written terminal syntax in a file and execute it all at once by writing a Python Script. This article explains how to create a Python script to open a new terminal and run a command for us.
Create Python Script That Opens a New Terminal and Run Commands in Linux
Before we begin, let’s revise some basics. Python scripts are executed in command line interfaces by locating the file and then running it. These files get executed and display the output.
There are many ways to create a Python script to open a new terminal and run a command. The first example is shown how to create a Python script that passes a Linux command to check the Python version inside the system.
Create Python Script to Check Python Version in Linux
If the Python version is needed to be checked by directly writing the command in the terminal, this syntax is used:
python3 --version
Which displays the Python version inside the system, like the one below:
jay@jay-virtual-machine:~$ python3 --version
Python 3.10.6
This is a command line syntax, which means it can only be written in a terminal. If it is wished to be written as plain text inside a text file with a .py
extension (ex: test.py
), it will lead to an error.
python3 --version
Output:
jay@jay-virtual-machine:~$ python3 test.py
Traceback (most recent call last):
File "/home/jay/test.py", line 1, in <module>
python3 --version
NameError: name 'python3' is not defined
A Python script must be created to make the syntax meaningful to both the Python compiler and the terminal to solve this issue.
To create a Python script to open a new terminal and run a command, create a text file with the .py
extension and write the following syntax:
import os
My_Cmmnd = "python3 --version"
os.system("gnome-terminal -e 'bash -c \"" + My_Cmmnd + ";bash\"'")
What does the code do?
-
The Python module
os
is imported in the first line of code. This module helps to pass commands to the operating system. -
The command line syntax that needs to be executed is stored in the variable named
My_Cmmnd
. The syntax used in this example checks the Python version of the system.My_Cmmnd = "python3 --version"
-
The third line of code sends the command to the system.
os.system("gnome-terminal -e 'bash -c \"" + My_Cmmnd + ";bash\"'")
The above code snippet does the following:
- The
os.system()
launches a sub-shell and executes the command inside the brackets. - The
gnome-terminal
command launches a newgnome-terminal
inside the Linux OS. - The variable
My_Cmmnd
contents are sent as a Bash command to the new terminal to execute.
The script executes the syntax and displays the desired output.
Python 3.10.6
jay@jay-virtual-machine:~$
Python Script to Keep an Open Terminal Alive and Run Commands in It
The last example showed how to create a Python script to open a new terminal and run a command by storing it inside a variable.
On the other hand, if the syntax is a one-line command, it can be put directly without storing it inside a variable. Create a file with the .py
extension and write the following syntax:
import os
os.system("gnome-terminal -e 'bash -c \"python3 --version\" '")
The above syntax launches a new terminal and executes the command. But using this method closes the terminal immediately after the command is executed.
Solving this issue requires placing a sleep
timer with the command:
import os
os.system("gnome-terminal -e 'bash -c \"python3 --version; sleep 5\" '")
The above syntax keeps the new terminal open for 5 seconds, though it can be increased per the requirement. If the terminal is required to be kept indefinitely active, use the syntax below.
import os
os.system("gnome-terminal -e 'bash -c \"python3 --version; bash\" '")
This keeps the terminal open and active until it is closed by the user.
Pass Commands to the New Terminal Using Python Submodule subprocess()
in Linux
We learned how to create a Python script to open a new terminal and run a command using the Python sub-module os
. Although using this in-built library to pass commands to the terminal is not recommended anymore.
That is because os
has been deprecated in the newer versions of Python. To avoid security risks, the Python sub-module subprocess
must be used instead.
To create a Python script to open a new terminal and run a command using subprocess
, create a file with a .py
extension similar to previous examples, and write this code inside it:
import subprocess
My_Cmmnd = "ls"
process = subprocess.Popen(
"gnome-terminal -e 'bash -c \"" + My_Cmmnd + ";bash\"'",
stdout=subprocess.PIPE,
stderr=None,
shell=True,
)
The UNIX command ls
lists the files in the current directory. As a result, a list of the files in the current directory is displayed if this command is run.
The subprocess()
module is imported first; then, the commands are stored in a variable. Inside the variable named process
, the arguments are defined for the subprocess()
.
The subprocess.Popen
constructor launches a child process inside a new gnome-terminal
when it reads the gnome-terminal -e
syntax. Lastly, the Python script opens a new terminal and runs the command.
Output:
jay@jay-virtual-machine:~$ python3 test.py
Desktop Downloads Pictures snap test2.py Videos
Documents Music Public Templates test.py
To learn about some other features that the Linux shell provides, let’s explore the use of subprocess()
to send arguments to the shell. For instance, the command in the program below, ls -la
, lists all the metadata and hidden files.
import subprocess
My_Cmmnd = "ls -la"
process = subprocess.Popen(
"gnome-terminal -e 'bash -c \"" + My_Cmmnd + ";bash\"'",
stdout=subprocess.PIPE,
stderr=None,
shell=True,
)
Output:
This command is executed as a string when the argument is passed to the shell. As a result, the shell interprets the string as a command argument at the beginning of the execution of our subprocess()
.
A drawback to the above method is that when the shell is called explicitly using shell=true
, it passes all the metacharacters, including white spaces, to the child process, which poses a threat in events of a shell injection.
To avoid such a situation, use a Python list instead. When using a list, the command to call is placed at the list’s 0th index, and the other arguments follow it.
Call the command used in the previous example as a list.
import subprocess
subprocess.run(["ls", "-la"])
Output:
The result of the command can also be stored inside a variable by setting the option capture_output=True
.
import subprocess
saved_results = subprocess.run(["ls", "-la"], capture_output=True)
print(saved_results.stdout)
Output:
It can be seen that the output displays the desired result, but there is no indexing, and the filenames are spread in an unorganized manner. This happens because the system saved the result of the ls -la
command in bytes.
To get the result as a string instead, set the option text=True
.
import subprocess
saved_results = subprocess.run(["ls", "-la"], capture_output=True, text=True)
print(saved_results.stdout)
Output:
Conclusion
This article explains how to create Python scripts to open a new terminal and run commands. The reader who goes through the article would be able to create a Python script to open a new terminal and run commands easily in Linux.